Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial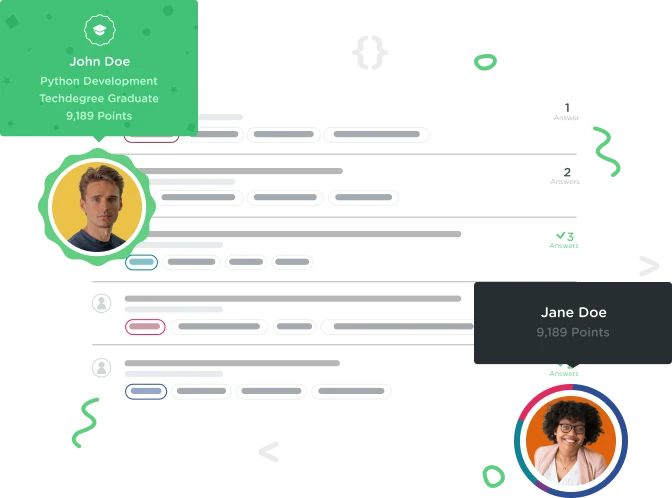
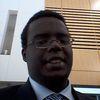
Leonard Morrison
Courses Plus Student 32,914 PointsI'm getting a 404 on the Cards page
For some reason, I can't access my cards file. It gives me the 404 message for some reason. Can someone please point out the error and help me out. Thanks in advance.
doctype html
html(lang="en")
head
title FlashCards Home
body
header
h1 Welcome to Leban's Flashcards!
section#content
h2= prompt
if hint
p
i Hint: #{hint}
else
p("Sorry, pal. You'll be on your own for this one!")
footer
Copyright 2018 by Leban Mohamed
cards.js:
const express = require('express');
const router = express.Router();
const { data } = require('../data/flashcardData.json');
const { cards } = data;
router.get('/:id', (req, res) =>
{
res.render('card', {
prompt: cards[req.params.id].question,
hint: cards[req.params.id].hint
});
});
module.exports = router;
index.js:
const express = require('express');
const router = express.Router();
//Testing the Middleware...
router.use(
(req, res, next) => {
console.log('Testing the Middleware...');
next();
});
let firstNames = ["Leban", "Hanad", "Abdi", "Shogo", "Mikako" ];
let lastNames = ["Mohamed", "Afrah", "Afrah", "Kanemoto", "Sunagawa"];
//Main Registration
router.get('/', (req, res) => {
const name = req.cookies.name;
if(name)
{
res.render('index', {name});
}
else
{
res.redirect('hello');
}
});
//Practice for the tables
router.get('/practice', (req, res) => {res.render('tablepractice', {firstNames, lastNames})})
//Get cookies from the hello page
router.get('/hello', (req, res) => {
res.render('hello', {name: req.cookies.name});
});
//
router.post('/hello', (req, res) => {
res.cookie('name', req.body.name);
res.redirect('/');
});
//Goodbye button
router.post('/goodbye', (req, res) =>
{
res.clearCookie('name');
res.redirect('/hello')
});
module.exports = router;
app.js
const express = require('express');
const bodyParser = require('body-parser');
const cookieParser = require('cookie-parser');
const app = express();
app.use(bodyParser.urlencoded({ extended: false}));
app.use(cookieParser());
app.set('view engine', 'pug');
const mainRoutes = require('./routes');
const cardRoutes = require('./routes/cards');
app.use(mainRoutes);
app.use('/cards', cardRoutes);
//Goodbye Action
app.use((req, res, next) =>
{
const err = new Error("Sorry, but the page you're looking for is in another castle!");
err.status = 404;
next(err);
})
app.use((req, res, next) =>
{
const err = new Error("Something's wrong with the page. Leban's hard at work making a fix. So go watch a Youtube video or something.");
err.status = 500;
next(err);
})
app.use((err, req, res, next) =>
{
res.locals.error = err;
res.status(err.status);
res.render('error', err);
})
app.listen(3000, () => {
console.log("The console is currently running on localhost:3000")
});
Card Data JSON file
{
"data":{
"title": "Leban's Fantastic Flash Cards!",
"cards": [
{
"Question": "What arcade game did Mario make his first appearance?",
"Hint": "He was jumping over barrels",
"Answer": "Donkey Kong (1981)"
},
{
"Question": "What programming language works with Express",
"Hint": "Starts with a \"J\"",
"Answer": "JavaScript"
},
{
"Question": "What is the Capital of Rhode Island",
"Hint": "Prov...",
"Answer": "Providence"
},
{
"Question": "Which Canadian Prime Minister was elected, only to be trounced within a year?",
"Hint": "Joe Who?",
"Answer": "Joe Clark"
},
{
"Question": "What year was Leban Mohamed born?",
"Hint": "Bill Clinton was inaugurated on this year for his 1st term.",
"Answer": "1993"
},
{
"Question": "Who composed the music for Sonic the Hedgehog 2?",
"Hint": "Leader of Dreams Come True",
"Answer": "Masato Nakamura"
},
{
"Question": "The arcade title that Luigi debuted?",
"Hint": "Something's coming out the plumbing poor Luigi's in bind",
"Answer": "Mario Bros. (1983)"
},
{
"question": "What is one way a website can store data in a user's browser?",
"hint": "They are delicious with milk",
"answer": "Cookies"
},
{
"question": "What is a common way to shorten the response object's name inside middleware?",
"hint": "It has the same abbreviation as \"resolution\"",
"answer": "res"
},
{
"question": "How many different values can booleans have?",
"hint": "Think: binary",
"answer": "2"
},
{
"question": "Which HTML element can contain JavaScript?",
"hint": "It starts with an \"s\"",
"answer": "<script>"
}
]
}
}
1 Answer

Usman Mehmood
Full Stack JavaScript Techdegree Student 14,330 PointsHey Leban,
You actually shouldn't be able to access the '/cards/' page because now it doesn't exist. You have to put in the number/id at the end to get a valid page.
ex. http://localhost:3000/cards --> would not work
http://localhost:3000/cards/1 --> works!
Phil Thomas
665 PointsPhil Thomas
665 PointsYour app is missing one line of code
app.set('views', path.join(__dirname, 'views'));
Adding this line before setting view engine will be perfect and the route will work properly after that.