Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial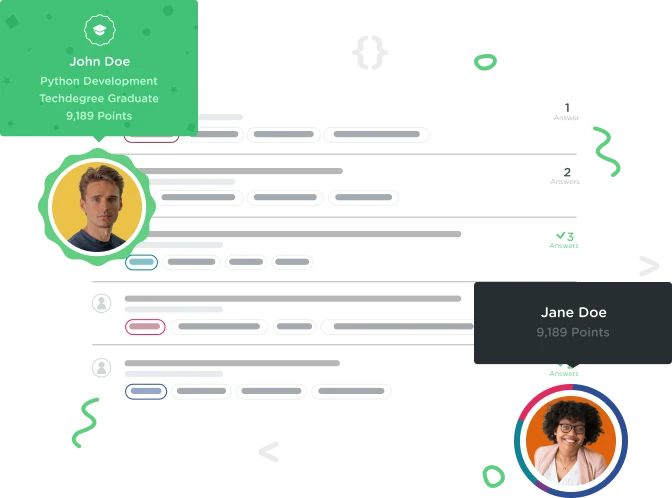

drstrangequark
8,273 PointsI'm getting an error here that I can't quite understand.
While writing this code challenge, I came up with something I THINK might work but the error it's giving me is that there is an unexpected " } " symbol on FrogStats.cs at line 10. I don't understand why that symbol is unexpected since it's closing the opening curly brace in the for loop. I'm not saying my solution is correct, I'm just surprised that THAT is the error it's throwing. Can anyone help explain why that curly brace is unexpected?
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
int i = 0;
int frogTongues = 0;
for(i < frogs.Length; i++)
{
int tongueLength = frogs.GetTongueLength;
frogTongues += tongueLength;
}
return frogTongues / frogs.Length;
}
}
}
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int TongueLength { get; }
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
1 Answer

andren
28,558 PointsIt complains about that because your for
loop declaration is incomplete. A for
loop has three parts, one which is executed before the loop starts, one that acts as the condition for the loop, and one that is executed after each loop.
You can leave the first part empty (though it's not common to do so) but you still have to place a semicolon separator so that the for
loop knows where each part starts and stops.
That means that if you change your for
loop to look like this:
for(; i < frogs.Length; i++)
Then that error would go away, though it's worth mentioning that it's not the only error in your code. Also as mentioned above it's not all that common to leave the first part empty, it's standard practice to use it to initialize the index variable. So if you want your code to follow best practices I would actually remove the int i = 0;
line from your solution and instead use the for
loop to initialize i
like this:
for(int i = 0; i < frogs.Length; i++)
Since you only asked for clarification on this error I won't explain the other issues with your code as you probably want to try to fix them on your own, but if you need more help with the challenge then feel free to ask me and I'll give you more help.
drstrangequark
8,273 Pointsdrstrangequark
8,273 PointsThank you very much! As you mentioned, I'll give a crack at fixing the other errors but I'll definitely come back if I can't figure it out.