Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial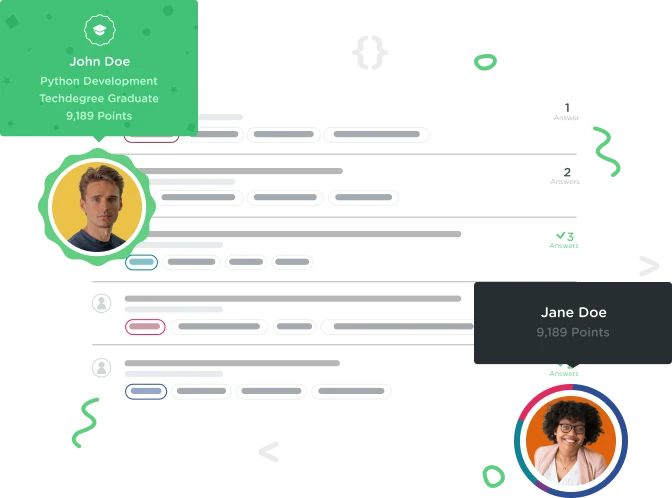
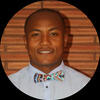
Christopher Hill
1,425 PointsI'm getting an error message saying the string must be an integer. I'm totally unsure where to go from here.
I'm getting an error message saying the string must be an integer. I'm totally unsure where to go from here. I know I need to print the specific index that start with the letter A.
continents = [
'Asia',
'South America',
'North America',
'Africa',
'Europe',
'Antarctica',
'Australia',
]
print("Continents:")
for continent in continents:
print(continent[0,3,5,6])
2 Answers
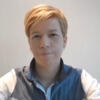
Ave Nurme
20,907 PointsHi Christopher
I think you wanted to access the continents starting with 'A' and had something like this in mind:
- continents[0], continents[3], continents[5], continents[6]
This is actually an interesting approach and it works but in this challenge you need to use a for-loop to find all the continents starting with the letter 'A' so let the for-loop do the job for you :-)
I've created two examples for you:
Example #1:
for continent in continents:
if continent[0] == 'A':
print(f'* {continent}')
In this first example we are looping over all the continents in the list. If the continent's first character is 'A', we print it out.
Example #2:
for continent in continents:
if continent.startswith('A'):
print(f'* {continent}')
Here's a slightly different approach. Startswith() is Python's string method which is quite easy to use and in this case it checks whether the string starts with 'A'.
I think in this particular challenge example 1 is more suitable so example 2 is just extra knowledge for you at this point.
Hope this helps!
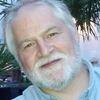
Jeff Muday
Treehouse Moderator 28,720 PointsI get the feeling you have programmed in other languages! I see what you were trying to do... that is clever.
HINT: the Challenges automated grader is simple-minded so avoid extra print statements since it can become confused.
One of the things I like about Python is that for every problem there are many different and correct solutions. I am suggesting one below that uses a conditional statement.
continents = [
'Asia',
'South America',
'North America',
'Africa',
'Europe',
'Antarctica',
'Australia',
]
for continent in continents:
if continent[0] == 'A':
print('* ' + continent)
But another solution that uses your approach would work too (see below). The only reason it would not suggest this be a choice you would make is that the automated grader sometimes will supply its own data during its test, and if that is the case, the indices of the 'A' continents could change without you knowing about it!
But for now, this approach works!
continents = [
'Asia',
'South America',
'North America',
'Africa',
'Europe',
'Antarctica',
'Australia',
]
indexes = [0,3,5,6]
for index in indexes:
print('* ' + continents[index])
Good luck with your Python journey. It's a lot of fun!