Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial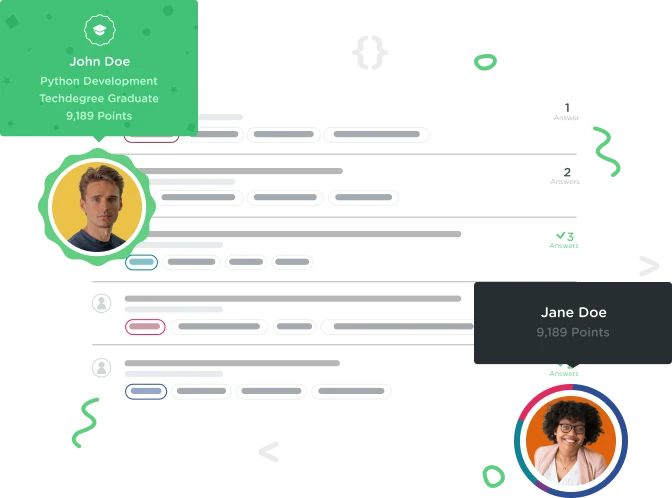

Dewi Tjin
2,275 PointsI'm getting an syntax error..
Around line 17, create a function named 'arrayCounter' that takes in a parameter which is an array. The function must return the length of an array passed in or 0 if a 'string', 'number' or 'undefined' value is passed in.
<script>
if (typeOf name === 'undefined' || typeOf name !== NaN || typeOf name === ""){
return 0;
};
function arrayCounter([name]){
return name.length;
};
</script>
3 Answers
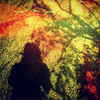
Kristen Law
16,244 PointsThe typeof
operator is used to get the value type of a variable. Without this operator, you are comparing the value stored inside the variable instead of the value type of the variable.
It's not necessary to create the array since you're only defining the function and not calling it. However, if you do create an array, make sure it contains ints, strings, etc. Right now the compiler thinks apple
and eggs
are variables, and it is creating an error since it can't find where they are defined. You can fix this by making those strings (put them in quotes).
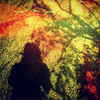
Kristen Law
16,244 PointsMake sure your "if statement" is inside your function. Also, your parameter name
should not be in square brackets.
See below for a few other adjustments to make:
function arrayCounter(array){
if (typeof array === 'string' || typeof array === 'number' || typeof array === 'undefined'){
return 0;
}
return array.length;
}

Dewi Tjin
2,275 PointsThe question wanted to pass an array.. so I created a variable with an array and called it..
var x = [apple,eggs]
function arrayCounter(x){
if (x === 'string' || x === 'number' || x === 'undefined'){
return 0;
}
return x.length;
}
And why did you use the key word typeOf??

Dewi Tjin
2,275 PointsThank-you!