Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial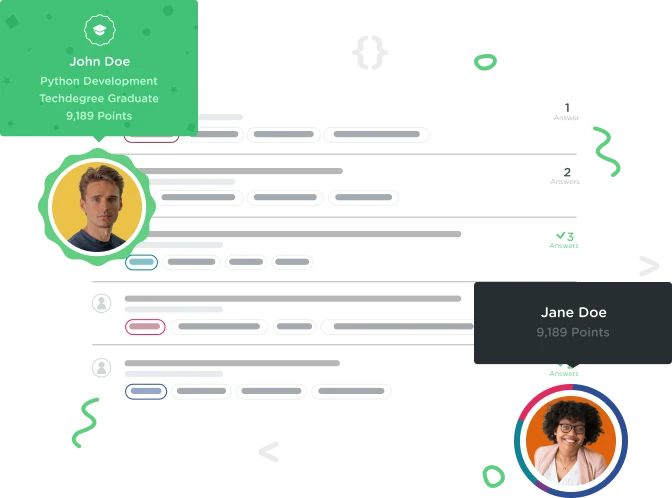
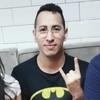
Daniel Sarmiento
10,265 PointsI'm getting an "undefined" error when I preview. Please Can you point out the error?
This is what I coded in my Js file:
var times = 10;
/* Gets a random color */
function getColor() {
return Math.floor(Math.random() * 256 );
}
/* Puts together a random RGB color */
function RandomRGB() {
var RGB;
var rgbColors = 3;
for (var i = 0; i < rgbColors; i++) {
if ( i === (rgbColors - 1)) {
RGB += getColor() + " ";
break;
}
RGB += getColor() + ' ,';
}
return RGB;
}
/* Puts together a number of RGB colors each one inside a div ready to be
printed out */
function RGBcolors(manyRGBs) {
var html;
for (var i = 0; i < manyRGBs; i++) {
html += "<div style: \"background-color: rgb(" + RandomRGB() + ")>" + "</div>";
}
return html;
}
document.write(RGBcolors(times));
2 Answers
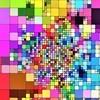
james south
Front End Web Development Techdegree Graduate 33,271 Pointsthe undefined comes from not initializing the html variable to the empty string. also the style tag needs to be followed by an equal = not a colon : and you were missing a quote " mark. finally, the divs need a size in order to appear, so in a css file i added a height and width. so here is my js:
var times = 10;
/* Gets a random color */
function getColor() {
return Math.floor(Math.random() * 256 );
}
/* Puts together a number of RGB colors each one inside a div ready to be printed out */
function RGBcolors(manyRGBs) {
var html = "";
for (var i = 0; i < manyRGBs; i++) {
html += "<div style=\"background-color: rgb(" + getColor() +","+getColor()+","+getColor()+ ")\"></div>";
}
return html;
}
document.write(RGBcolors(times));
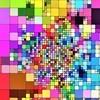
james south
Front End Web Development Techdegree Graduate 33,271 Pointsyou could do something like this:
function colors(){
var cols = "";
for (var i = 0; i < 3; i++){
cols += getColor() + ",";
}
return cols.substring(0,cols.length-1);
}
and then call it like:
function RGBcolors(manyRGBs) {
var html = "";
for (var i = 0; i < manyRGBs; i++) {
html += "<div style=\"background-color: rgb(" + colors() + ")\"></div>";
}
return html;
}
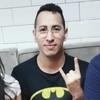
Daniel Sarmiento
10,265 PointsThanks!
Daniel Sarmiento
10,265 PointsDaniel Sarmiento
10,265 PointsThank you!
and also
How can I implement a function that stores the 3 RGB colors so that I don't need to call "getColor()" three times? Any hints?
Thanks again!