Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial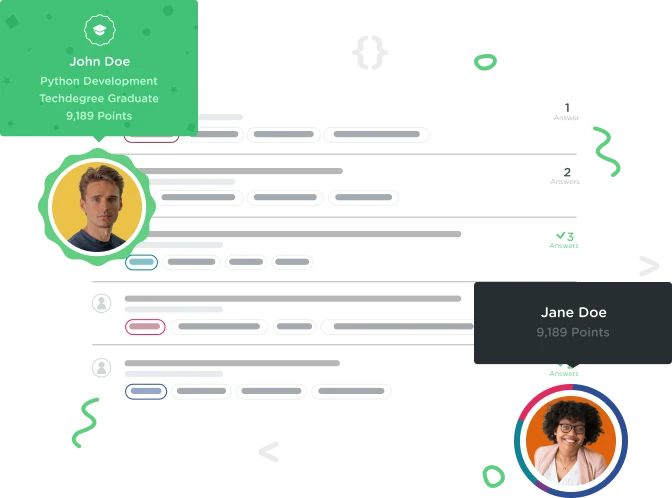

Jason Smith
8,668 Pointsi'm getting the error can't find combo, what am i doing wrong?
also, am i on the right track with the code?
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
def combo (iter1, iter2):
new_list = []
counter = 0
for index in iter1, iter2:
newlist.append(counter, counter)
counter + 1
return new_list
2 Answers
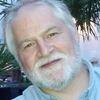
Jeff Muday
Treehouse Moderator 28,722 PointsJason,
I can tell you understand the concept-- let's build on that. Your code is pretty close to the answer. The thing I love about Python is there are so many different ways to get at an answer-- not just a single correct code solution.
They tell us iter1 and iter2 must be of the same length. Great, that means we only need to step through the first iterable. We use your counter variable to extract the parallel values into a tuple. The tuples are then appended to your new list.
Note a tuple is kind of like a list, but it is surrounded by parenthesis and is not mutable like a list.
e.g. (iter1[counter], iter2[counter])
def combo (iter1, iter2):
new_list = []
counter = 0
for index in iter1: # fix this
new_list.append( (iter1[counter], iter2[counter]) ) # fix this
counter + 1
return new_list
*Here is another solution that shortens things up-- the enumerate() is super handy to use! * Note: we use the '_' underscore character when we want to ignore something that is returned.
def combo(iter1, iter2):
new_list = []
for counter, _ in enumerate(iter1):
new_list.append( (iter1[counter], iter2[counter]) )
return new_list
And even shorter using a "list comprehension". Personally, I think many list comprehensions are pretty strange looking but some Pythonistas insist they are faster-- I think readability and clear intentions are more important than trying to be slick.
def combo(iter1, iter2):
return [ (iter1[idx], iter2[idx]) for idx, _ in enumerate(iter1) ]

Jason Smith
8,668 Pointsthese are great, thanks!