Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial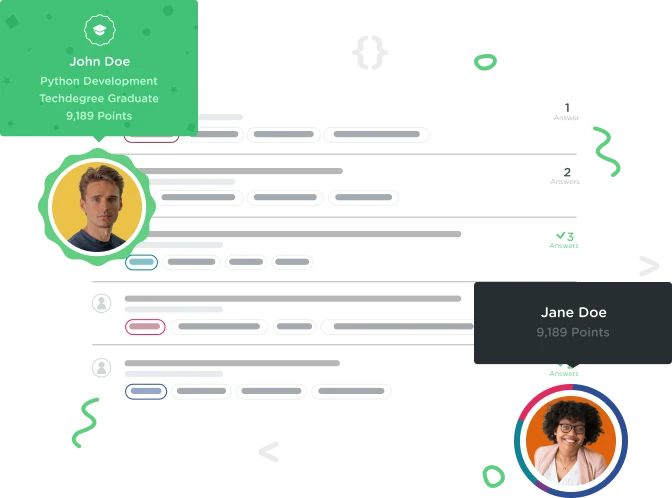
jackmartin3
5,559 PointsI'm getting the wrong answer but my code runs without error, what am i missing/not seeing here?
I'm working my way through this track and struggling a bit with code challenges, but i'm getting there.
Usually taking a break and coming back does wonders for me.
Anyway... i've tried this problem and my code appears to run fine but gives me the wrong answer at the end.
I'm guessing its because i am doing something wrong with the counter and it isnt updating the end value correctly.
How do i fix this?
#here is my string
a_string = "This is a test string that repeats words like This and is and a and test to give me interesting counter results"
#--------------------------------------------------------------------------------------------------------------
#create function named word_count() that takes a string argument
def word_count(a_string):
#create a dictionary that will be returned
my_dictionary = {}
#split the string using the .split() method/fuctnion (Still not sure on terminology)
split_string = a_string.split()
#loop through the words in the split string
for word in split_string:
#create a counter for the number of times a word appears
counter = 0
#go through and check if the word appears more than once
if word in split_string:
counter +=1
#make the word the key and the counter the value and update into the dictionary
my_dictionary.update({word: counter})
return my_dictionary
#------------------------------------------------------------------------------------------------------------
#call the function with my string
word_count(a_string)
2 Answers
jackmartin3
5,559 PointsI went about it a different way in the end. I figured that its easier to check if a word is in the dictionary and if it isn't, add it to the dictionary with the value of 1. If it already exists, increase the value by one.
For anyone interested, i ended up with this:
#here is my string
a_string = "This is a test string that repeats words like This and is and a and test to give me interesting counter results"
#--------------------------------------------------------------------------------------------------------------
#create function named word_count() that takes a string argument
def word_count(a_string):
#split the string and make it all lower case (had errors with this earlier so made it all lower)
split_string = a_string.lower().split()
#create an empty dictionary to store the words and values
my_dictionary = {}
# make a loop that goes through each word in the split string
for word in split_string:
#if it does exist, increase the value by 1.
if word in my_dictionary:
my_dictionary[word] += 1
#if the word doesnt exist in the dictionary, add it with the value of 1.
else:
my_dictionary[word] = 1
#print the dictionary
print(my_dictionary)
#return the dictionary
return my_dictionary
#------------------------------------------------------------------------------------------------------------
#call the function with my string
word_count(a_string)

Josh Keenan
20,315 PointsYour code isn't working out how many of each word is in the string, but just adding the word to the dictionary; the count also goes up with every word in the string, and in the dictionary you'll have each item with the same value. Example: The string input is: 'This is my string'
{'This': 4, 'is': 4, 'my': 4, 'string': 4}
Your for loop can't tell the difference between each word, essentially it is thinking: ~For every word in this string, I add 1 to the count and add the word to the dictionary along with the value of the count
jackmartin3
5,559 PointsI see, thanks Josh.
The downside is, i have no clue how to fix it without restarting the dictionaries section for the 4th time. I wonder why i cant take in dictionaries?
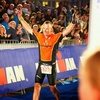
Steve Hunter
57,712 PointsI think counter
will get set to zero on every iteration of the loop - so counter++
will increment it to 1 at the most (only if the if
is true - which I think it will always be?). On the next loop, it'll be reset to 0.
Add each word to the dictionary first? That'll pull out duplicates, giving you a list of keys, perhaps - you can only add a key once. Then do the count per key, updating the value with the count. That might work - but I've no idea how to do that in Python! I might try in Java to see if that helps here.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsNice solution - good work.