Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial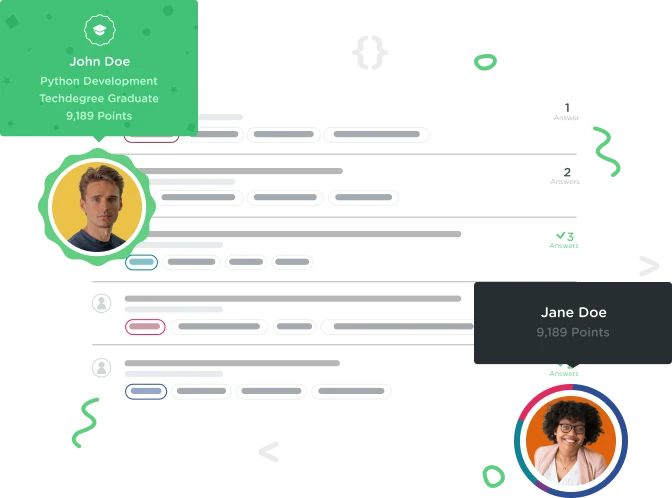

Aaron Haughton
2,606 PointsI'm getting this error in my quiz of arrays: Make sure you're removing the 6th item from the array at index 5!
I'm putting this in and it works in my Xcode playground:
arrayOfInts.remove(at: 5)
is this incorrect removing the 6th entry in my array?
// Enter your code below
var arrayOfInts = [1,2,3,4,5,6]
arrayOfInts.append(7)
arrayOfInts + [8]
let value = arrayOfInts[4]
arrayOfInts.remove(at:5)
let discardedValue = arrayOfInts
2 Answers
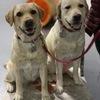
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Aaron,
You're almost there! You are correctly removing the value from the array. To understand where the error is, we need to distinguish between the value that a method returns, and the value of an entity that has been mutated by a method.
In the specific case of Array.remove(at:)
, this method will:
- remove an element from an array (i.e., it will mutate the array by taking the value at the specified index out of the array);
- return the value removed.
So the following line does two things:
arrayOfInts.remove(at:5)
It mutates arrayOfInts
such that it goes from [1,2,3,4,5,6,7]
to [1,2,3,4,5,7]
. But it also returns the value 6
. In your case, you haven't assigned the result of the method call to a variable or constant, so that 6
is just lost.
In your subsequent line,
let discardedValue = arrayOfInts
You have assigned the value of arrayOfInts
, which let's remember is [1,2,3,4,5,7]
to discardedValue
.
Hope that points you in the right direction,
Cheers
Alex
PS. You might have been surprised to note that I described your array as being [1,2,3,4,5,6,7]
and not [1,2,3,4,5,6,7,8]
(you can verify that this is true in a Playground). The reason is this line:
arrayOfInts + [8]
Your code here creates an expression that combines arrayOfInts
and an array literal [8]
, which evaluates to [1,2,3,4,5,6,7,8]
. However, you do not save that evaluated value anywhere, and so arrayOfInts
stays as [1,2,3,4,5,6,7]
and [1,2,3,4,5,6,7,8]
is lost.
Strictly, the challenge should have failed your code there since it does not meet the instructions:
Next, add another item to the array by concatenating an array. When concatenating, assign the results of the expression back to
arrayOfInts
.
(You did the first sentence but not the second). To fulfill the task you could have done this:
arrayOfInts = arrayOfInts + [8]
Or taken advantage of Swift's syntactic sugar which provides the +=
operator to combine concatenation and assignment into a single operator:
arrayOfInts += [8]

Aaron Haughton
2,606 PointsThanks. This helped.