Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial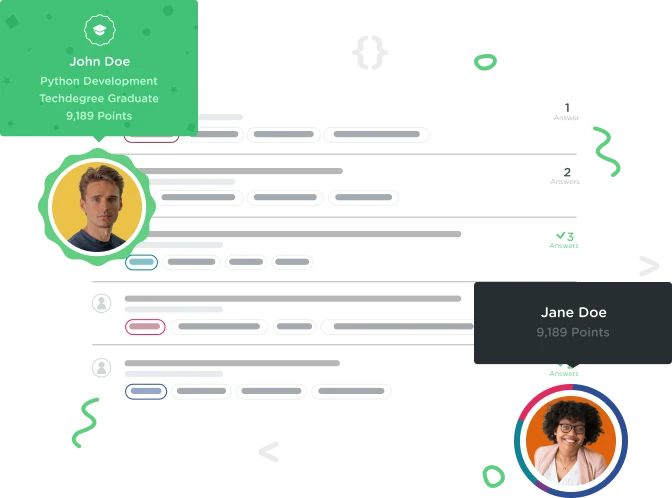

MARLON LOZANO
767 PointsIm having a hard time understanding returns in functions. Exactly what is a return?
i understand the return gathers information from the function and can be executed by a variable for calling the function but im not very sure i know what return does?
2 Answers
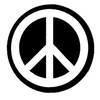
john larson
16,594 PointsMarlon, hi. In programming there seems to be a lot of focus on making functions that can be used not just once, but as many times as they are useful. You haven't got to the point yet where it will become apparent why this matters. At this point in your learning just know... it's a thing. Reusable functions. Ill try to make an example and maybe clarify return
function add(a, b){
return a + b
}
//instead of just printing a + b when the function runs,
// a + b is sent back to the function call
// these following three lines are three separate function calls
// to the same function
//getting three different results
add(2, 2) //send in 2, 2: 4 is returned
add(4, 4) //send in 4, 4: 8 is returned
add(6, 6) // send in 6, 6: 12 is returned
// none of these have been printed to the page
// they are waiting for you to do something with them
// therefore you can use them however you like
// if you just printed the numbers to the page
// you would be done with them
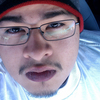
Chyno Deluxe
16,936 PointsReturns allow you return information base on the logic of the function.
for example lets say you had a simple adding app that takes to numbers and adds them together. You can do something like this.
function sum(n1,n2){
return n1 + n2;
}
var num1 = 5;
var num2 = 10
var total = sum(num1, num2); // 15
so instead of writing
var total = num1 + num2; // 15
you are creating a reusable function that will return that information and turn the variable into that returned value.
I hope this helps.

MARLON LOZANO
767 PointsWhen you say reuse the function what do you mean? are you adding a value to your variables after the function or are those getting returned? sorry if im confusing you i just still dont get it . seems simpler to write (var total = num1 + num2;)