Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial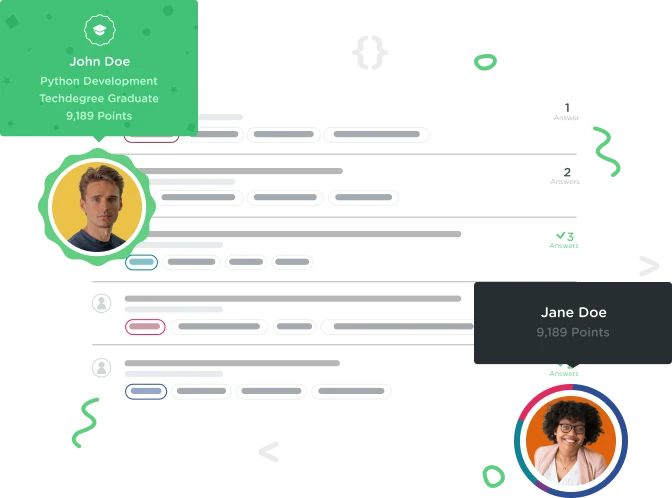

Cindy L Francies
13,318 PointsI'm having a problem with Getting the Response Body.
I've matched my code agains the video, it works up until the previous point. Here's my code.
var request = http.get("http://teamtreehouse.com/"+ username + ".json", function(response){
console.log(response.statusCode)});
request.on("error", function(error) {
console.error(error.message);
});
//read the data
response.on('data', function(chunk) {
console.log('BODY: ' +chunk);
});
thoughts appeciated... tx
9 Answers

james white
78,399 PointsHi Cindy,
You didn't give a link to the "Getting the Response Body" challenge.
I'm guessing it's:
..where the challenge says:
Challenge Task 1 of 2
Without renaming any of the variables, modify the data callback to concatenate the stream of data to the responseBody.
If you look at the video (and download the zip files for the project), you'll see code like this:
function get(username){
//Connect to the API URL (https://teamtreehouse.com/username.json)
var request = http.get("http://teamtreehouse.com/" + username + ".json", function(response){
var body = "";
//Read the data
response.on('data', function (chunk) {
body += chunk;
});
response.on('end', function(){
This should give you some idea of the type of code the challenge is looking for..
So the specific code given at the start of the challenge
(and remember the challenge says NOT to change the names of the variables given in the code) is:
var http = require("http");
var request = http.get("http://teamtreehouse.com/chalkers.json", function(response){
var responseBody = "";
response.on("data", function(dataChunk) {
});
response.on("", function(){
console.log(responseBody);
});
});
request.on("error", function(error){
console.error(error.message);
});
First thing you might notice is that, just to trick you (or get you to think),
they've changed the var (variable) named 'body' (that's used in the videos)
to the var 'responseBody' on this line:
var responseBody = "";
...and (just to really mess with your head) on the they've changed
the response call back line:
response.on("data", function(dataChunk) {
Notice: 'chunk' has been changed to 'dataChunk'.
However the code templating (pattern) is basically intact (nearly the same).
Now on to the tricky concatenation part.
If you want to do it the 'hardcore' javascript way,
use the standard javascript convention '+=' to concatenate
dataChunk on to the var defined empty string responseBody
with a line like:
responseBody += dataChunk;
Or (the other way which is easier for me to understand)
is just to use the plus sign (+) like so:
responseBody = responseBody + dataChunk;
To make the full code look like this:
var http = require("http");
var request = http.get("http://teamtreehouse.com/chalkers.json", function(response){
var responseBody = "";
response.on("data", function(dataChunk) {
//This code line should work: responseBody += dataChunk;
//but I also passed with the line below:
responseBody = responseBody + dataChunk;
});
response.on("", function(){
console.log(responseBody);
});
});
request.on("error", function(error){
console.error(error.message);
});
..and lo and behold, with such code the Treehouse answer engine should like you..
(i.e. you pass part 1 of 2 for the challenge!)
Hopefully these "thoughts" was what you were looking for (appreciated)..
..and I should add that the second part of the challenge:
Challenge Task 2 of 2
See the console.log(responseBody); in that callback around line 10? Fix the listener so that the callback gets executed when the response has fully finished.
Is easy by comparison. This line:
response.on("", function(){
...just needs to be changed to:
response.on("end", function(){
Don't get confused by the substitution of double quotes for single quotes..I guess they are interchangeable in javascript.
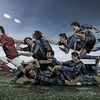
San Francisco
28,373 PointsGreat answer thank you

thomas howard
17,572 Pointsamazing work, James!!
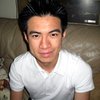
jason chan
31,009 Pointsvar http = require("http");
var request = http.get("http://teamtreehouse.com/chalkers.json", function(response){
var responseBody = "";
response.on("data", function(dataChunk) {
//This code line should work: responseBody += dataChunk;
//but I also passed with the line below:
responseBody = responseBody + dataChunk;
});
response.on("end", function(){
console.log(responseBody);
});
});
request.on("error", function(error){
console.error(error.message);
});

Steve Gifford
4,810 PointsI am having the same issue as this person, looks like someone else reported it as well. Perhaps you can look at my code and make sure I'm not missing anything obvious?
//Solution: use node.js to connect to treeshouse's API to get profile information to print out var http = require("http"); var username = "sgifford007";
function printMessage(username, badgeCount, points) { var message = username + " has " + badgeCount + " total badge(s) and " + points + " points in Javascript"; console.log(message); }
//Connect to the APR URl (https://teamtreehouse.com/username/json) var request = http.get("http://teamtreehouse.com/" + username +".json", function(response){ console.log(response.statusCode); //Read data response.on('data', function (chunk) { console.log('BODY: ' + chunk); });
//Parse data //Print data });
request.on("error", function(error){ console.log('problem with request: ${error.message}'); });
It runs fine except that the body chunk does not come back. if I use a body variable and (typeof) it does return that it's a string. But again I get nothing returned in the console.
Help please?
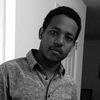
Abinet Kenore
10,082 Points/*Here the whole code for Challenge task 1 of 2 */
var https = require("https"); var request = https.get("https://teamtreehouse.com/chalkers.json", function(response){ var responseBody = "";
response.on("data", function(dataChunk) {
//This code line should work: responseBody += dataChunk;
//but I also passed with the line below:
responseBody = responseBody + dataChunk;
});
response.on("end", function(){
console.log(responseBody);
});
});
request.on("error", function(error){ console.error(error.message); });
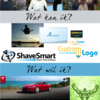
amadeo brands
15,375 PointsThanks great input :)
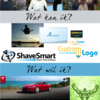
amadeo brands
15,375 PointsThanks great input :)

farhaday m
7,998 Pointsvar http = require("http"); var request = http.get("http://teamtreehouse.com/chalkers.json", function(response){ var responseBody = "";
response.on("data", function(dataChunk) {
responseBody = responseBody + dataChunk;
});
response.on("end", function(){
console.log(responseBody);
});
});
request.on("error", function(error){ console.error(error.message); });

Marlene Guzman
9,231 PointsThe solution is simpler than i thought it would be. I tried so many things and i was starting to go into a rabbit hole. so they want you to add
responseBody += dataChunk;
so your code should look like this..
const https = require("https");
const request = https.get("https://teamtreehouse.com/chalkers.json", response => {
let responseBody = "";
response.on("data", dataChunk => {
responseBody += dataChunk;
});
response.on("", () => {
console.log(responseBody);
});
});
request.on("error", error => {
console.error(error.message);
});

Deloris Luckett
6,418 Pointsconst https = require("https"); const request = https.get("https://teamtreehouse.com/chalkers.json", response => { var responseBody = "";
response.on("data", function(dataChunk) { responseBody = responseBody + dataChunk; });
response.on("", function() {
console.log(responseBody);
});
});
request.on("error", function(error) { console.error(error.message); });
the above code passes

Namra Hudaibia
6,712 Pointsworst course on treehouse ..disappoint........;(
Rafael silva
23,877 PointsRafael silva
23,877 Pointsconst https = require("https"); const request = https.get("https://teamtreehouse.com/chalkers.json", response => { let responseBody = "";
});
request.on("error", error => { console.error(error.message); });