Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial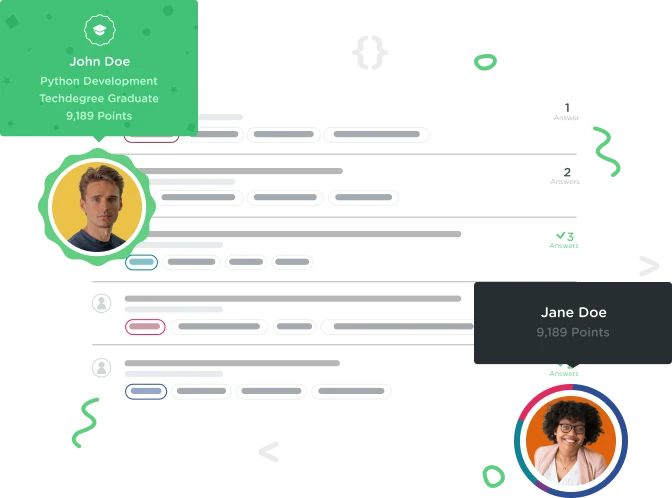

maryclairegilmore
Courses Plus Student 30 PointsI'm having a small problem with my code
The Challenge: First, declare a variable named myArray and assign it to an empty array. Great! Now populate myArray with two strings. Put your full name in the first string, and your Skype handle in the second. Next, declare a function named cutName. It should expect a parameter name. cutName should return an array by breaking up the input string into individual words. Example: cutName("Douglas Crockford") should return ["Douglas", "Crockford"] Example: cutName("John B. Smith") should return ["John", "B.", "Smith"] Declare a new variable named myInfo and assign it to an empty object literal. Add the following three key-value pairs to myInfo: Key: fullName Value: The result of calling cutName on the name string within myArray. Key: skype Value: The Skype handle within myArray. Key: github Value: If you have a github handle, enter it here as a string. If not, set this to null instead.
My Code: var myArray = [];
myArray = ['Mary Claire Gilmore', 'maryclairegilmore'];
function cutName(name) { var testname = myArray.splice[0]; return name; }
var myInfo = { fullName: cutName(myArray[0]) [0], skype: cutName(myArray[1]), github: 'maryclairegilmore', };
1 Answer

Shawn Rieger
9,916 PointsYou have a few issues here. your cutName function will never work.
function cutName(name) { // <-- you pass in name
var testname = myArray.splice[0]; // <-- this is not proper syntax for array.splice, also maybe you mean to use array.slice?
return name; // <-- return name without modifying it.
}
Splice method actually changes the contents of an array while slice returns a portion of an array without modifying it. Check out: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/slice https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/splice
Also...
var myInfo = {
fullName: cutName(myArray[0])[0], // <-- although your technically returning array here, this is not the corrent way to handle this
skype: cutName(myArray[1]),
github: 'maryclairegilmore',
};
By the looks of what you're doing, I think this is more what you're trying to accomplish...
var myArray = ['Mary Claire Gilmore', 'maryclairegilmore'];
var myInfo = {
fullName: myArray[0],
skype: myArray[1],
github: 'maryclairegilmore',
};