Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial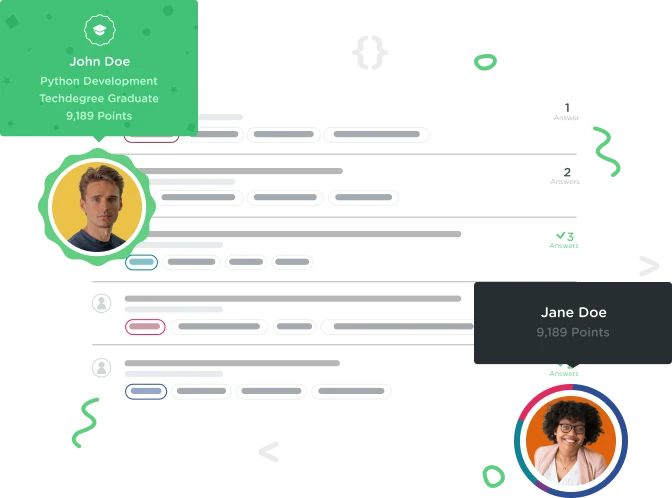
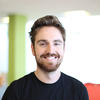
Kristian Woods
23,414 PointsI'm having an issue with back-facing visibility
So, when I click on the button, I want the whole element and its contents to disappear. I'm using CSS to create a flip effect. So I have two divs placed together using absolute positioning. However, when I click on the button, one div disappears faster than the other. So you can see the h1, 'Header', reversed. I don't want that effect. I want the whole element(s) to disappear at the same time.
<!DOCTYPE html>
<html lang="">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="new_flip.css">
<title></title>
</head>
<body>
<div class="wrapper">
<div class="flip_container container">
<div class="side_a item1">
<h1>Header</h1>
</div>
<div class="side_b">
<h1>Flip</h1>
<a href="#" class="btn">SEE MORE</a>
</div>
</div>
<div class="flip_container container">
<div class="side_a item2">
<h1>Header</h1>
</div>
<div class="side_b">
<h1>Flip</h1>
<a href="#" class="btn">SEE MORE</a>
</div>
</div>
<div class="flip_container container">
<div class="side_a item3">
<h1>Header</h1>
</div>
<div class="side_b">
<h1>Flip</h1>
<a href="#" class="btn">SEE MORE</a>
</div>
</div>
<div class="flip_container container">
<div class="side_a item4">
<h1>Header</h1>
</div>
<div class="side_b">
<h1>Flip</h1>
<a href="#" class="btn">SEE MORE</a>
</div>
</div>
<div class="flip_container container">
<div class="side_a item5">
<h1>Header</h1>
</div>
<div class="side_b">
<h1>Flip</h1>
<a href="#" class="btn">SEE MORE</a>
</div>
</div>
<div class="flip_container container">
<div class="side_a item6">
<h1>Header</h1>
</div>
<div class="side_b">
<h1>Flip</h1>
<a href="#" class="btn">SEE MORE</a>
</div>
</div>
<div class="flip_container container">
<div class="side_a item7">
<h1>MyHeading</h1>
</div>
<div class="side_b">
<h1>Flip</h1>
<a href="#" class="btn">SEE MORE 5</a>
</div>
</div>
</div>
<script src="target.js"></script>
</body>
</html>
* {
box-sizing: border-box;
}
body {
font-family: sans-serif;
}
.wrapper {
display: grid;
grid-template-columns: repeat(auto-fit, 1fr);
grid-auto-rows: minmax(200px, 1fr);
}
@media screen and (min-width: 1024px) {
.wrapper {
grid-template-columns: repeat(7, 1fr);
}
}
/*
------- FLIP OVERLAY -- START
*/
.flip_container {
position: relative;
color: #fff;
transition: all .9s ease-in-out;
transform-style: preserve-3d;
}
.flip_container:hover {
transform: rotateY(-180deg);
}
.side_a {
position: absolute;
top: 0;
right: 0;
bottom: 0;
left: 0;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
}
.side_b {
position: absolute;
top: 0;
right: 0;
bottom: 0;
left: 0;
background-color: #666;
display: flex;
flex-direction: column;
justify-content: space-around;
align-items: center;
}
.side_a:hover,
.side_b:hover{
cursor: pointer;
}
.side_a,
.side_b {
backface-visibility: hidden;
}
.side_b {
transform: rotateY(180deg);
}
.item1 {
background-color: #004875;
}
.item2 {
background-color: #125c8a;
}
.item3 {
background-color: #166a98;
}
.item4 {
background-color: #1875a8;
}
.item5 {
background-color: #2c87b8;
}
.item6 {
background-color: #369acd;
}
.item7 {
background-color: #40a9dd;
}
.btn {
color: #40a9dd;
background-color: #fff;
padding: 10px;
text-decoration: none;
border-radius: 4px;
transition: all .4s;
}
.btn:hover {
color: #fff;
background-color: #40a9dd;
}
.hide {
opacity: 0;
}
/*
------- FLIP OVERLAY -- END
*/
let side_a_collection = document.querySelectorAll('.side_a'); //array / NodeList
let side_b_collection = document.querySelectorAll('.side_b'); //array / NodeList
let btns = document.querySelectorAll('.btn'); //array / NodeList
let headings = document.querySelectorAll('h1'); //array / NodeList
let container = document.querySelectorAll('.container'); //array / NodeList
side_b_collection.forEach((sideb) => {
sideb.addEventListener('mouseover', () => {
sideb.style.backgroundColor = 'orange';
});
sideb.addEventListener('mouseleave', () => {
sideb.style.backgroundColor = '';
});
});
btns.forEach((btn) => {
btn.addEventListener('click', (e) => {
if (e.target.tagName === 'A') {
if (e.target.textContent == 'SEE MORE') {
let button = e.target;
let parentDiv = e.target.parentElement.parentElement;
parentDiv.classList.toggle('hide');
}
}
});
});
headings.forEach((header) => {
if (header.textContent.toLowerCase() == 'myheading') {
header.textContent = 'New';
}
});
Here is a link to my CodePen, to see it in action:
1 Answer
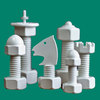
Steven Parker
231,269 PointsApparently, the opacity transition isn't working for "side_b". That's a puzzle I don't have a ready solution for. And the explicit opacity settings seem to override the backface hiding, but this one might be normal.
But you can explicitly hide "side_a" to eliminate seeing 'Header' reversed by adding this to your CSS:
.hide .side_a {
display: none;
}
Kristian Woods
23,414 PointsKristian Woods
23,414 PointsHey, Steven. Thanks for the response! that worked!
I tried adding the hide class to all children elements at the same time
is this a good solution?
Steven Parker
231,269 PointsSteven Parker
231,269 PointsIt's not necessary to add the class to the child elements, since the only thing affected by the class is opacity and it is always inherited.