Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial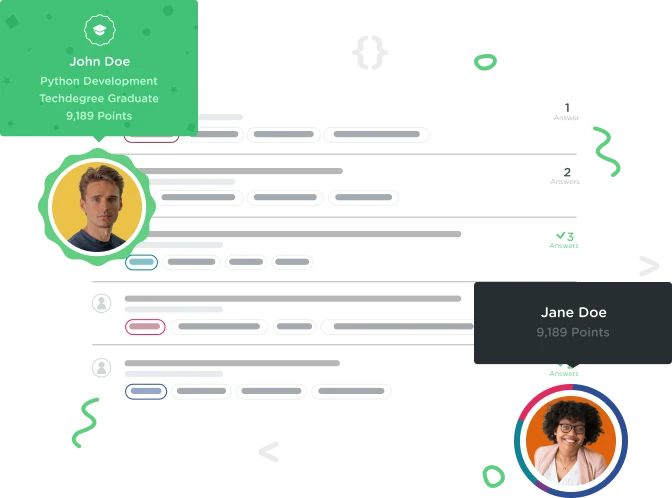

Yusuf Mohamed
2,631 PointsI'm having some trouble with sending integer data with an intent. (android)
Hello!
I'm currently making an interval training app. In the app I need to send from one activity to another class about how man intervals the user has chosen.
So I made this intent that sends the data to a recycler view. The problem is that the int automatically turns into zero by itself which leads to the recycler list not being created. I've tried to make the list with a random number and it works, so nothing wrong with that part of the code.
Also after some debugging I narrowed down the problem to the getintent part of the because before I send the the data the number is correct but after I've sent it the number always turns into zero.
It would have been super nice if you could take a look at the code and see if you could solve the problem. Here's the code:
public class MainActivity extends AppCompatActivity {
Button decrementButton;
TextView intervalCountTextView;
Button incrementButton;
int intervalCount = 0;
Button intervalButtonSetter;
private int numberOfIntervals;
private static final String TAG = "MainActivity";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
decrementButton = findViewById(R.id.decrementButton);
intervalCountTextView = findViewById(R.id.intervallCount);
incrementButton = findViewById(R.id.incrementButton);
intervalButtonSetter = findViewById(R.id.intervalButtonSetter);
decrementButton.setVisibility(View.INVISIBLE);
decrementButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
intervalCount--;
intervalCountSetter();
}
});
// make sure you can't decrement past zero
incrementButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
intervalCount++;
intervalCountSetter();
}
});
intervalButtonSetter.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
sendDataToAdapter();
}
});
}
private void intervalCountSetter() {
String intervalCountString = Integer.toString(intervalCount);
intervalCountTextView.setText(intervalCountString);
if (intervalCount > 0) {
decrementButton.setVisibility(View.VISIBLE);
} else {
decrementButton.setVisibility(View.INVISIBLE);
}
}
private void sendDataToAdapter() {
String numberOfIntervalsString = intervalCountTextView.getText().toString();
numberOfIntervals= Integer.parseInt(numberOfIntervalsString);
Intent intent = new Intent(this, CustomAdapter.class);
if (numberOfIntervals > 0) { intent.putExtra("Interval Count", numberOfIntervals);
Log.d(TAG, "sendDataToAdapter: We have the numbers");
Log.d(TAG, "sendDataToAdapter: " + numberOfIntervals);
startTimeActivity();
} else {
Toast.makeText(this, "You have to have at least 1 interval.", Toast.LENGTH_LONG).show();
}
}
private void startTimeActivity() {
Intent intent = new Intent(this, TimeActivity.class);
startActivity(intent);
Log.d(TAG, "startTimeActivity: TimeActivity has been started" + numberOfIntervals);
}
}
public class TimeActivity extends AppCompatActivity {
public static final String TAG = TimeActivity.class.getSimpleName();
private int numberOfIntervals;
private ArrayList<Integer> WTV = new ArrayList<>();
private ArrayList<Integer> WET = new ArrayList<>();
private ArrayList<Integer> RTV = new ArrayList<>();
private ArrayList<Integer> RET = new ArrayList<>();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_time);
Log.d(TAG, "onCreate: Started");
//Intent intent = getIntent();
//numberOfIntervals = intent.getIntExtra("Interval Count", 0);
//Log.d(TAG, "" + numberOfIntervals);
initializeViews();
}
private void initializeViews() {
Log.d(TAG, "initializeViews: Preparing views");
//Make sure they can change through the R.strings
WTV.add(R.string.work_text_view);
WET.add(R.string.default_time_value);
RTV.add(R.string.rest_text_view);
RET.add(R.string.default_time_value);
initializeRecyclerView();
}
private void initializeRecyclerView() {
Log.d(TAG, "initializeRecyclerView: Initialize RecyclerView");
RecyclerView intervalRecyclerView = findViewById(R.id.intervalRecyclerView);
CustomAdapter adapter = new CustomAdapter(this, WTV, WET, RTV, RET);
intervalRecyclerView.setAdapter(adapter);
intervalRecyclerView.setLayoutManager(new LinearLayoutManager(this));
}
}
public class CustomAdapter extends RecyclerView.Adapter<CustomAdapter.ViewHolder> {
private static final String TAG = "CustomAdapter";
private ArrayList<Integer> mWorkTW = new ArrayList<>();
private ArrayList<Integer> mWorkET = new ArrayList<>();
private ArrayList<Integer> mRestTW = new ArrayList<>();
private ArrayList<Integer> mRestET = new ArrayList<>();
private Context mContext;
private int numberOfIntervals;
public CustomAdapter( Context context , ArrayList<Integer> mWorkTW, ArrayList<Integer> mWorkET, ArrayList<Integer> mRestTW, ArrayList<Integer> mRestET) {
this.mWorkTW = mWorkTW;
this.mWorkET = mWorkET;
this.mRestTW = mRestTW;
this.mRestET = mRestET;
this.mContext = context;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View customView = LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.time_row, viewGroup,false);
ViewHolder holder = new ViewHolder(customView);
return holder;
}
@Override
public void onBindViewHolder(@NonNull ViewHolder viewHolder, int i) {
Log.d(TAG, "onBindViewHolder: called");
viewHolder.workTextView.setText(R.string.work_text_view);
viewHolder.restTextView.setText(R.string.rest_text_view);
viewHolder.workEditText.setHint(R.string.default_time_value);
viewHolder.restEditText.setHint(R.string.default_time_value);
}
@Override
public int getItemCount() {
Intent intent = new Intent();
numberOfIntervals = intent.getIntExtra("Interval Count", numberOfIntervals);
Log.d(TAG, "" + numberOfIntervals);
return numberOfIntervals;
}
public class ViewHolder extends RecyclerView.ViewHolder {
TextView workTextView;
EditText workEditText;
TextView restTextView;
EditText restEditText;
ConstraintLayout parentLayout;
public ViewHolder(@NonNull View itemView) {
super(itemView);
workTextView = itemView.findViewById(R.id.workTextView);
workEditText = itemView.findViewById(R.id.workEditText);
restTextView = itemView.findViewById(R.id.restTextView);
restEditText = itemView.findViewById(R.id.restEditText);
parentLayout = itemView.findViewById(R.id.parentLayout);
}
}
}
2 Answers

Ken Alger
Treehouse TeacherYusuf;
I'm a bit sleep deprived so am probably missing it, but where are you passing the intent
information from sendDataToAdapter
to the startActivity
function? I see that you are creating a new intent in startTimeActivity()
but is the value of numberOfIntervals
in the intent at that point?
Again, I could be missing it, but I don't see the connection there.
:shrug:
Ken

Yusuf Mohamed
2,631 PointsI have created two intents, one is for sending the data to my CustomAdapter class and the other intent is to start the TimeActivity.

Yusuf Mohamed
2,631 PointsShould it matter that when I write Intent intent = getIntent that it doesn't work?

Ken Alger
Treehouse TeacherI see the two different intents, but where are you passing information from the intent in sendDataToAdapter
to another one?

Yusuf Mohamed
2,631 PointsBumpidity bump
Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsTonnie Fanadez
UX Design Techdegree Graduate 22,796 Pointsfollowing