Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial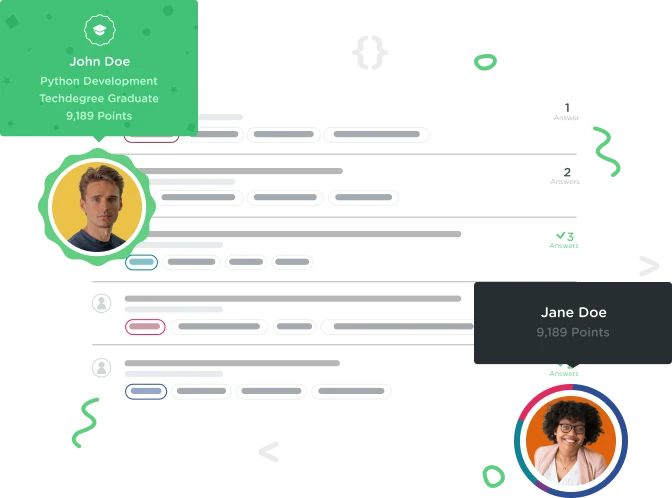

Zack Massingill
868 PointsI'm having trouble checking for the '$' symbol in a string
I can figure out how to check for letters, and then throw an exception, but i dont know how to check for the '$' symbol in the string.
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
private String normalizeDiscountCode(String discountCode){
discountCode=discountCode.toUpperCase();
char symbol='$';
for(int i=0;i<discountCode.length();i++){
if(!Character.isLetter(discountCode.charAt(i))||discountCode.indexOf(i)!=symbol){
throw new IllegalArgumentException("a letter, or a $ symbol, is required");
}
}
return discountCode;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
}
2 Answers

Zack Massingill
868 PointsI figured it out, my logic was incorrect. It should have been checking for both letters and the symbol, not either or.
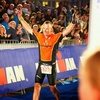
Steve Hunter
57,712 PointsHi Zack,
Glad you got that sorted; sorry not to get back to you last night.
Did you figure out a cleaner way of managing the loop? You've gone down the local counter variable route but a for:in
loop is a little cleaner.
That would look like:
for(char letter : discountCode.toCharArray()){
}
This does the same as your loop; it iterates over `discountCode
one char at a time, storing each char in turn within the letter
variable. But it doesn't require a counter variable or any incrementing. Once you've got that set up you can do the tests:
for(char letter : discountCode.toCharArray()){
if(! Character.isLetter(letter) && letter != '$'){
throw new IllegalArgumentException("Informative error message");
}
}
Putting all that together gives you the whole method:
private String normalizeDiscountCode(String discountCode){
for(char letter : discountCode.toCharArray()){
if(! Character.isLetter(letter) && letter != '$'){
throw new IllegalArgumentException("Informative error message");
}
}
return discountCode.toUpperCase();
}
I hope that helps,
Steve.