Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial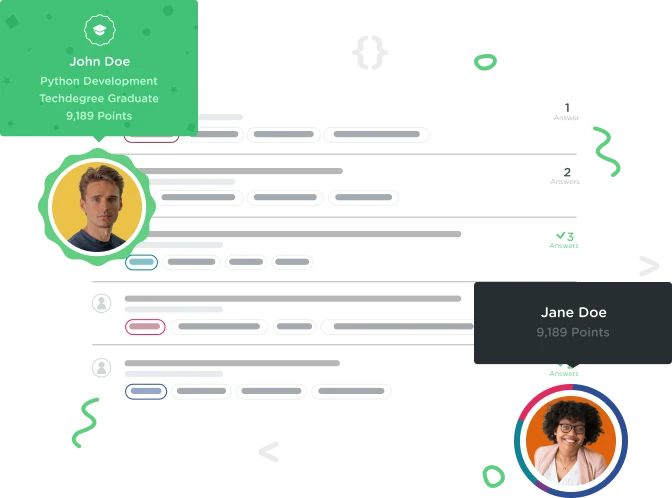

Ben Gordon
690 PointsI'm having trouble understanding loops. In the "While and Do While Loops Tutorial," what does the line "Int w = 0;" mean
Also have trouble understanding the statement:
printf("letter %d is %c \n", w, letters[w]);
2 Answers
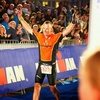
Steve Hunter
57,712 PointsHi Ben,
The w
is a counter used to access the array at each element. So, the letters
array contains three letters, one at index zero, the next at 1, then the last at 2.
Setting w
to zero means that accessing the array like letters[w]
will give you the first element of the array.
The printf
statement prints a formatted string. This means you can insert flags in your string (the things with a % sign) and then the code will insert values of variables into your string. %d
lets you insert a number and %c
lets you insert a char.
So,
printf("letter %d is %c \n", w, letters[w]);
inserts the number of the index w
at point %d and the contents of letters[w]
at point %c. Your output will be something like "Letter 1 is a", then "Letter 2 is b" as you carry on looping through the code. The \n
inserts a new line so it doesn't all follow on the same one.
Does that make sense?
Steve.
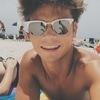
garyguermantokman
21,097 PointsThe i is an iterator, i starts out at an initial value of 0 and continues until it reaches the value count (condition becomes false).
for (int i = 0; i < 4; i++) {}
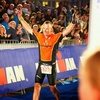
Steve Hunter
57,712 PointsAs is w
in the video before the quiz regarding do ... while
loops. Exactly the same principle; using a variable as an index counter.
Traditionally, the letters i
, j
and k
are used for this. For some reason the video regarding while
loops uses w
. Exactly the same concept, however.
The question came from the tutorial video, not the linked quiz. ;-)
Steve.
Ben Gordon
690 PointsBen Gordon
690 PointsSteve, thanks for the explanation. I understand most of the concept now, but what do you mean when you say "the w is a counter used to access the array at each element?" Also, from my understanding now, "w" is used only to show how many times the loop is run through, correct?
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi Ben,
No problem; glad to help.
The
w
is being used for two things, yes. It is being used to terminate the loop after three iterations, yes. But it is also being used to access the array and to print out each element in that array one at a time. The part of theprintf
statement that saysletters[w]
is accessing theletters
array one element at a time. As the loop lops,w
is first 0, then 1, then 2. The loop is told to continue only whenw
is less than 3, so these are the three values ofw
we are dealing with.At first pass,
w
is zero, theprintf
prints out (among other things)letters[w]
which is the same asletters[0]
which is thechar
'a'. The next loop, because we incrementw
with++w
,w
is now 1 soletters[w]
is the same asletters[1]
which is the nextchar
, 'b'. And so on.So,
w
is being used in two ways. One to control the termination of the loop (else we'd be here all day!) and secondly to access the array at each element, one-at-a-time.I hope that's a little clearer!
Steve.
Ben Gordon
690 PointsBen Gordon
690 PointsYes, thanks for the explanation. Finally have a clear idea as to what for and while loops are.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsExcellent. For loops are best used when you know how many times you want to iterate (or can easily calculate that). While loops and do while loops are best used when looping is conditional upon other stuff that changes - they can loop once, not at all, or forever.
Happy coding!