Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial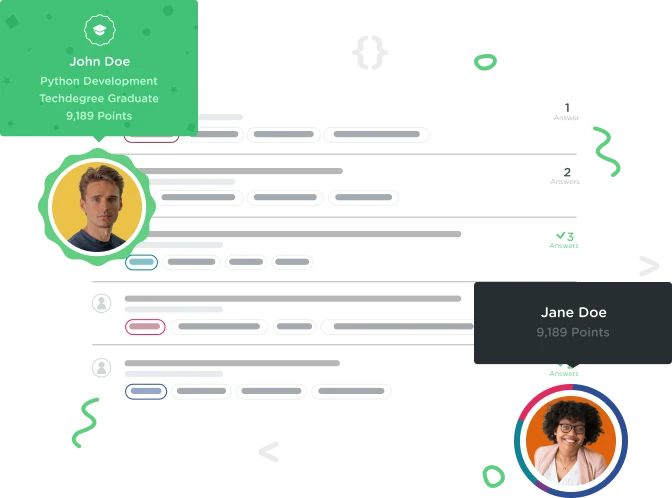
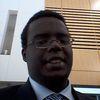
Leonard Morrison
Courses Plus Student 32,914 PointsI'm having trouble with Pundit Authorization and I'm creating my Blog using Ruby on Rails.
I am currently working on my Blog using Ruby on Rails. I'm trying to implement authorization using Pundit.
I'm having trouble trying to get the Update Policy to work. I keep getting the Update Policy wrong and the Create method doesn't even allow to write to write an entry before submitting. I hope that somebody could nudge me in the right direction in terms of where I need to go from here.
Here's the Post Controller:
class PostsController < ApplicationController
before_action :authenticate_user!, except: [:index, :show]
after_action :verify_authorized, except: [:index, :show]
def index
@posts = Post.order(created_at: :desc).page(params[:page]).per(10)
#authorize @posts
end
def new
@post = current_user.posts.build
authorize @post
if @post.save
redirect_to @post, notice: 'Post was successfully created.'
else
render :new
end
end
def show
set_post
end
def edit
set_post
end
def update
set_post
@post.update(post_params)
redirect_to @post
end
def destroy
set_post
authorize @post
@post.destroy
redirect_to action: "index", notice: "The post was removed"
end
def upvote
@post.upvote_from current_user
authorize @post
end
def downvote
@post.downvote_from current_user
authorize @post
end
def create
@post = current_user.posts.build(post_params)
authorize @post
respond_to do |format|
if @post.save
format.html {redirect_to @post, notice: 'Blog post has been posted!'}
format.json {render :show, status: :created, location: @post}
else
format.html {render :new}
format.json {render json: @post.errors, status: :unprocessable_entity}
end
end
end
private
def post_params
params.require(:post).permit(:title, :content, :header_image, uploads: [])
end
def set_post
@post = Post.friendly.find(params[:id])
# authorize @post
end
end
(Note: I use a Role Table with role_id and name.) Here's the Post Policy:
class PostPolicy < ApplicationPolicy
class Scope < Scope
def resolve
scope.where(user_id: @user.try(:id))
end
end
attr_reader :user, :post
def initialize(user, record)
@user = user
@post = post
end
def show?
true
end
def index?
true
end
def create?
is_contributor_or_admin?
end
def update?
is_author_of_post_or_admin?
end
def destroy?
is_author_of_post_or_admin?
end
private
def user_not_authorized
flash[:alert] = "Can't let you do that, " + @user.username + "!"
end
def is_admin?
@user.role_id == 1
end
def is_contributor_or_admin?
@user.role_id == 1 || @user.role_id == 2
end
def is_author_of_post_or_admin?
@user.role_id == 1 || @post.user.username == @user.username
end
end
If there is more information that you need, please let me know. Thanks in advance! Thank you very and I hope you all have a successful New Year!