Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial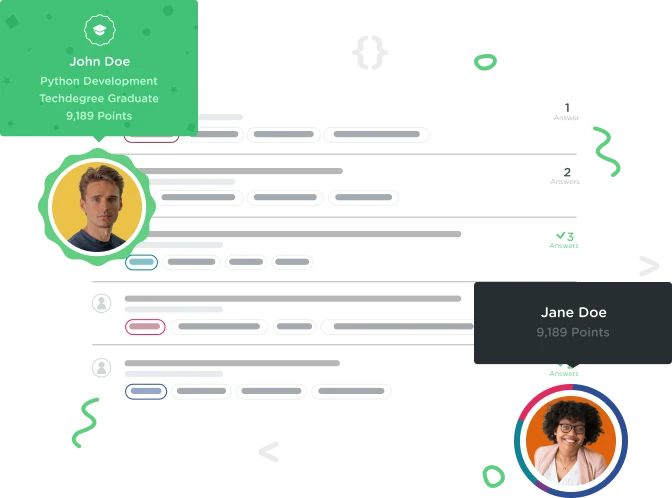
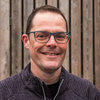
Chris Guy
Python Web Development Techdegree Graduate 14,615 PointsI'm having trouble with the first testing code challenge
Hi,
I'm stuck on the first testing code challenge,
Now add a test that creates an instance of the Writer model and, using self.assertIn, make sure the email attribute is in the output of the mailto() method.
I have created an instance of the Writer model (named 'writer') and I'm trying to access the output of the mailto() method by using writer.mailto or writer.mailto() - but both fail and I don't understand why. My code is pasted below, any help appreciated as I've been staring at this for a while now!
# articles/tests.py
from django.test import TestCase
from .models import Writer
class WriterModelTestCase(TestCase):
'''Tests for the Writer model'''
writer = Writer.objects.create(
name="George Orwell",
email="george@1984.com",
bio="Dystopian fiction is my game."
)
self.assertIn(writer.email, writer.mailto)
# articles/models.py
from django.db import models
class Article(models.Model):
headline = models.CharField(max_length=255)
publish_date = models.DateTimeField()
content = models.TextField()
writer = models.ForeignKey('Writer')
def __str__(self):
return self.headline
class Writer(models.Model):
name = models.CharField(max_length=255)
email = models.EmailField()
bio = models.TextField()
def __str__(self):
return self.name
def mailto(self):
return '{} <{}>'.format(self.name, self.email)
2 Answers
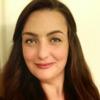
Jennifer Nordell
Treehouse TeacherHi there, Chris Guy ! Looks like you're doing terrific! I only see two things that are sort of missing. First, the test case should be a method on that class you just made. I might suggest defining a method named something like test_mailto_method
passing in self
. Secondly, mailto
is not an attribute, it is a method so where you have writer.mailto
you will need writer.mailto()
.
Hope these hints help, but let me know if you're still stuck!
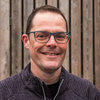
Chris Guy
Python Web Development Techdegree Graduate 14,615 PointsHey Jennifer Nordell
Thanks, that made sense (in the end) - though I also needed to add a setUp to make it work as expected...
from django.test import TestCase
from .models import Writer
class WriterModelTestCase(TestCase):
'''Tests for the Writer model'''
def setUp(self):
self.writer = Writer.objects.create(
name="George Orwell",
email="george@1984.com",
bio="Dystopian fiction is my game."
)
def test_mailto_method(self):
self.assertIn(self.writer.email, self.writer.mailto())
Is this what you had in mind?