Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial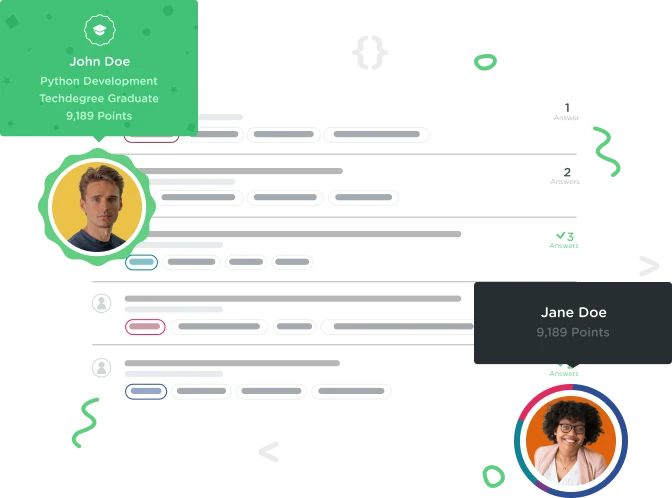

Cobie Fisher
4,555 PointsI'm just a bit confused
The instructions on "Adding Instance Methods" objective is a little unclear or incomplete (it's either that or I just don't get it)
The instructions are this:
"Given the struct below in the editor, we want to add a method that returns the person’s full name. Declare a method named getFullName() that returns a string containing the person’s full name. Note: Make sure to allow for a space between the first and last name"
If I'm supposed to call my method getFullName(), does that mean that there are no inputs between the braces? Since structures have stored properties with no real value, how am I supposed to return the person's full name?
Can someone tell me the answer or tell me what to do in greater detail?
24 Answers
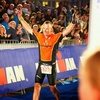
Steve Hunter
57,712 PointsHi Cobie,
Without the link to the challenge I'm guessing a bit on this.
So, you've got a struct
that has two stored properties, first & last name. The challenge is to write a method that returns the full name of the individual, like "Steve Hunter". That about right?
I'll guess at the struct:
struct Person{
let firstName: String
let lastName: String
}
Hopefully, that's somewhere near. Now you want a method that return firstname + " " + lastName
. We can use interpolation to do that:
struct Person{
let firstName: String
let lastName: String
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}
I hope that helps - if not, let me have the link to the challenge.
Steve.
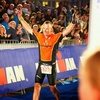
Steve Hunter
57,712 PointsThis worked:
struct Person {
let firstName: String
let lastName: String
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}
let aPerson = Person(firstName: "Steve", lastName: "Hunter")
let fullName = aPerson.getFullName()
The code you have posted looks fine. Can you post the rest of it? The issue may be there.
Steve.
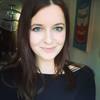
Marina Alenskaja
9,320 PointsHi Steve Just a thought: Wouldn't it be possible and make more sense to do something like this:
func getFullName(first: String, last: String) -> String {
If you can see what I mean by this.. I just think it would make more sense to write the names when actually creating the instance instead of in the method?
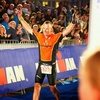
Steve Hunter
57,712 PointsHi Marina,
The getFullName()
method is an accessor method. It enables easy, but indirect, access to the properties of a struct instance, or the member variables of a class instance. Let's stick to a struct
for now as that's what's relevant to the point. The Person
struct is initialized by setting its two properties, firstName
and lastName
such that we can create an instance of Person
, as above like:
let myIdentity = Person(firstName: "Steve", lastName: "Hunter")
So, now we have an instance of the Person
struct called myIdentity
. We can access the properties that have been set using dot notation:
myIdentity.firstName // this outputs "steve"
myIdentity.lastName // guess what this outputs ;-)
Accessor methods, and helper methods, are used within the struct to enable better, more relevant, access to the properties. It isn't a strange requirement to want to have access to a person's full name - that's kinda what it's for! So, we create a function to help with accessing the instance's full name. We pass nothing into this method as we just want to get something out of the instance. We just want the full name; the instance already has all the information it needs to return this - so there's no need to pass in any parameters; there's no new information here. So, this accessor method simply returns the above two lines of code in one string from within the instance (allowing it to access the properties directly).
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
This works for every instance of Person
.
Your example has two parameters passed into the instance - that information already exists within the instance so there no requirement to add extra.
I hope I explained my understanding of that OK!
Steve.
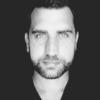
Jhoan Arango
14,575 PointsHello:
Remember that methods do not need to have parameter in order for them to work. This challenge is asking you to create one that does not necessarily have them. But it does tell you that it needs to return a string.
When there is an indication of a return, then now you know that this method has to give back a value of some type.
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
Since this function is inside a struct, it's called a method, or an instance method. So what you are returning are the store properties related to the struct. So when creating an instance, you can call this function, on the values that you give the instance.
Good luck, hope this helps.
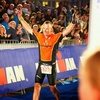
Steve Hunter
57,712 PointsYou need to put inverted commas around the strings you are passing in, like firstName:
"Steve
".
let aPerson = Person(firstName: "raphael", lastName: "reiter")
Hope that works for you!
Steve.
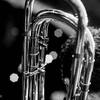
Raphael Reiter
6,820 Pointsthanks Steve? what a silly mistake...

Jon Barnett
2,004 Pointscorrect answer as of 18th Aug 2018, the challenge has changed slightly, no longer getFullName, just fullName()
struct Person {
let firstName: String
let lastName: String
snip
}
The space required is just part of the returned string, it's there just before the second backslash
so to see a returned string with the space included-
let anyone = Person(firstName: "Bill", lastName: "Bloggs")
anyone.fullName()
will show "Bill Bloggs" in Xcode's right hand er... bit.
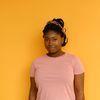
Marilyn Magnusen
14,084 PointsAll that is needed for the code to pass the first task:
struct Person {
let firstName: String
let lastName: String
func fullName() -> String {
return "\(firstName) \(lastName)"
}
}

Morgan Waage
11,580 PointsI had parenthesis around String here:
func getFullName() -> (String) {
When I removed them it worked.
Thanks!

Jimmy Auldridge
11,578 PointsI am also a bit confused then these challenges ask for a return fromt the method. Im not sure if im supposed to specify what its returning at the start of the function or just simply add a return at the end. How do i know when asked?
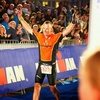
Steve Hunter
57,712 PointsHi Austin,
Have you tried removing the brackets around the returned type so have -> String
rather than -> (String)
Steve.
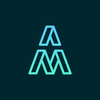
Austin McKinney
3,750 PointsWow, that worked. Wonder why the treehouse compiler rejected the (String) version though? It seems odd that it worked in Xcode, but not in the compiler. Is the method you suggested a better way or is this just a limitation of the treehouse compiler?
Thanks for your help, Steve!
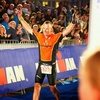
Steve Hunter
57,712 PointsI'm not sure what best practice is; maybe Jhoan can help with that. But for single returned values, I never use brackets. It is possible to return more than one value, a tuple for example, which then does need the brackets around the returned data types.
Steve.

Morgan Waage
11,580 PointsWhat about the next step? When I add these lines to the code posted above:
let aPerson = Person(firstName: "John", lastName: "Smith")
let fullName = aPerson.getFullName()
I get an error saying: Bummer! The value being assigned to fullName must be the result of calling the instance method getFullName()
Isn't that what I am doing? It returns the correct result in Xcode.

Charlotte Glasson
4,191 PointsJust copied your code, had the same error as you when put inside the function but if you put it after all the code outside of it then it is correct :)

Vivien Gauthier
1,537 PointsHi!
I don't understand, my code worked in the playground, but the code challenge says that it can't pass the compiler :/ ...
struct Person {
let firstName: String
let lastName: String
func getFullName() -> String {
let fullName: String = firstName + " "+lastName
return fullName
}
}
let aPerson = Person(firstName: "Vivien", lastName: "Gauthier")
aPerson.getFullName()
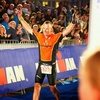
Steve Hunter
57,712 PointsHi there,
You need to assign the return value of getFullName
into a constant called fullName
- just doing that inside the method isn't what it is after.
let aPerson = Person(firstName: "Vivien", lastName: "Gauthier")
let fullName = aPerson.getFullName() // assign this
You can simplify your return
inside the method to just this (if you use concatentation):
func getFullName() -> String {
return firstName + " " + lastName
}
Or, if you prefer interpolation:
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
But creating the constant fullName
inside the struct is not what the challenge is looking for.
I hope that helps,
Steve.

Vivien Gauthier
1,537 PointsThank you Steve, makes total sense.
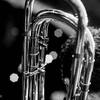
Raphael Reiter
6,820 Pointsi dont understand why my code doesnt work...
struct Person {
let firstName: String
let lastName: String
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}
let aPerson = Person(firstName: raphael, lastName: reiter)
let fullName = aPerson.getFullName()
gaborcsecsetka
8,446 PointsI think you need to put inverted commas around the strings like "Raphael" and "Reiter" everything else seems fine :D
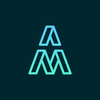
Austin McKinney
3,750 PointsCan someone please explain what I'm doing wrong? The code runs fine in Xcode, but the Treehouse editor keeps giving me the following error message: " Bummer! The value being assigned to fullName must be the result of calling the instance method getFullName()"
struct Person {
let firstName: String
let lastName: String
func getFullName() -> (String) {
return "\(firstName) \(lastName)"
}
}
let aPerson = Person(firstName: "Brian", lastName: "McKinney")
let fullName = aPerson.getFullName()

Rich Braymiller
7,119 Pointsi hate being confused...hoping I can eventually get this stuff...

Siamak Pourhabib
2,962 PointsI guess you're just missing init in your code
let aPerson = Person.init(firstName: "Siamak", lastName: "Pourhabib")
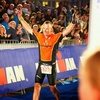
Steve Hunter
57,712 PointsAs far as I know, the init method is called implicitly, not explicitly. You don't need to call the method in your code. It works as a constructor of each class instance which the class itself triggers.

Siamak Pourhabib
2,962 PointsThank you Steve! you're actually right. The following code should be passed:
struct Person {
let firstName: String
let lastName: String
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}
let aPerson = Person(firstName: "Siamak", lastName: "Pourhabib")
let fullName = aPerson.getFullName()
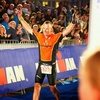
Steve Hunter
57,712 PointsI think the correct code has been posted in this thread for quite some time. I hope you enjoy your Treehouse experience!

Siamak Pourhabib
2,962 PointsOh yea that's right. I should have read the whole above messages. I do enjoy learning here. Thank you!

hashemmaraqa
2,100 PointsI might sound silly, but why do you put a slash before (first name) and (last name). and where is the space you were supposed to put
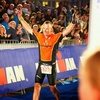
Steve Hunter
57,712 PointsThe slash is the syntax for string interpolation. The format \(var_name)
is the way of inserting the value of var_name
into a string. It's covered in the videos. As far as I know this hasn't changed with the later versions of Swift; this response does relate to the Swift language from 2015 - there have been many revisions of it since then!
And the space between the first & last name is between the closing bracket of firstName)
and the slash of \(lastName
:
return "\(firstName) \(lastName)"
^
Or you can use concatenation which is a little ugly:
return firstName + " " + lastName
but the space is more obvious.
I hope that explains.
Steve.

Nathalie Dory
2,912 PointsI don't understand the point of putting the function in the Struct. Why can't it be outside the Struct?
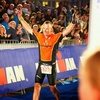
Steve Hunter
57,712 PointsHi Nathalie,
The reason the function is inside the struct is so that every instance of the struct can have that functionality.
In this challenge, we create an instance of the struct called aPerson
. That, and every, instance has the getFullName()
function which returns the full name of the created struct instance. We are able to create as many instances of Person
as we like and all of them will have the getFullName()
function accessible with dot notation. So ...
let steve = Person(firstName: "Steve", lastName: "Hunter")
steve.getFullName()
... will work just fine. Yes, you could write the same functionality outside the struct:
let fullName = steve.firstName + " " + steve.lastName
But you'd have to do that for every instance. Using the internal function avoids duplication of code. Plus, this is an example - it is using very simple code. In the real world, the function could be doing some complex maths on stored properties. or accessing the internet to gather data, or controlling an external device - anything! So, internalising the func allows each instance of the struct possess built-in functionality which is consistent across all instances of the struct.
I hope that makes sense!
Steve.

Nathalie Dory
2,912 PointsYes it does now! Thanks
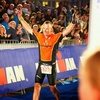
Steve Hunter
57,712 PointsNo problem!

Nathalie Dory
2,912 Pointsokay now reviewing everything, and I don't understand how the function doesn't take the parameters of first name and last name in order to get the result. Surely that is neccesary? How does it know what information to take in? How come sometimes it does and sometimes it doesn't need parameters??
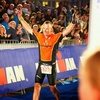
Steve Hunter
57,712 PointsHi Nathalie,
The func doesn't need the parameters as it is part of that instance of the struct. Internal functions can access the stored properties in the struct so there's no need to pass them in as parameters.
Some functions do need parameters if they aren't able to directly access the variables/constant they need. But for functions inside a struct or a class, the stored properties or member variables are directly accessible from inside.
I hope that help,
Steve.

Nathalie Dory
2,912 Pointsyes thank you

Michael touboul
3,053 PointsThx a lot Steve Hunter -> you saved me after hours of trying alone, Hours of frustration! The instructions were very confusing to me, Thx again. Just to be clear with my self regarding the learning process - is it normal to not complete this challenge/quiz on my own?
Jhoan Arango
14,575 PointsJhoan Arango
14,575 PointsYou got the struct right !
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsLucky guess! :-)
Jhoan Arango
14,575 PointsJhoan Arango
14,575 PointsOr just to good at coding ? lol
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsI need to get out more, that's true! :-)
To be fair, Treehouse naming conventions are so strong that it isn't difficult to guess roughly what they'll call things.
Jhoan Arango
14,575 PointsJhoan Arango
14,575 PointsThat is true, but somethings the instructions can be a bit confusing. Specially if you are new to the language.
Cobie Fisher
4,555 PointsCobie Fisher
4,555 PointsThanks! This worked!
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsTrue - it does all take a little time to sink in.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsNo problem, Cobie Fisher - glad it worked. :-)
Jhoan Arango
14,575 PointsJhoan Arango
14,575 PointsSteve Hunter do you have an e-mail address ? If so can you e-mail me at jhoannarango@msn.com
Juan Davin
1,074 PointsJuan Davin
1,074 PointsHi Steve, correct me if im wrong, but the job could be easily done without the struct right? then whats struct exactly for?
Josh Rondestvedt
3,830 PointsJosh Rondestvedt
3,830 PointsThis code still doesn't pass the challenge.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi Josh,
Can you post a link to the current challenge? Swift has changed a lot since this thread was created. Looking at the comments on here, the code did pass the challenge so something must have changed. A link would help.
Thanks,
Steve.
Josh Rondestvedt
3,830 PointsJosh Rondestvedt
3,830 PointsSteve Hunter https://teamtreehouse.com/library/objectoriented-swift-3/complex-data-structures/adding-instance-methods
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsThanks Josh,
One reason that the above code doesn't work in this challenge (a different challenge to the one you're doing - Swift 3 didn't exist back then!) is that the original challenge wanted a function named
getFullName()
. The challenge you're doing just wants one calledfullName()
. So, changing that for step one gives you:That should work for you.
Steve.
Neiko Frye
3,840 PointsNeiko Frye
3,840 PointsHey Steve Hunter Replying a year later, and still hoping I get an answer :)
I was wondering if we could pass in values in the Person struct WITHOUT specifying the variable type. I know in past courses I have watched instructors enter _ before the variable type.
Can we do this? so when I call the getFullName method I can do the following below: aPerson = Person("Steve", "Hunter")
rather than: aPerson = Person(firstName: "Steve", lastName: "Hunter")
Jhoan Arango
14,575 PointsJhoan Arango
14,575 PointsNeiko Frye ,
You can, you just need to ignore the "argument label" in the initializer's body with the underscore.
In this example, I created an extension of the Struct "Person" so that you do not lose the memberwise initializer that comes standard in the Struct type. This way, you can have 2 initializers to choose from.
Hope this helps