Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial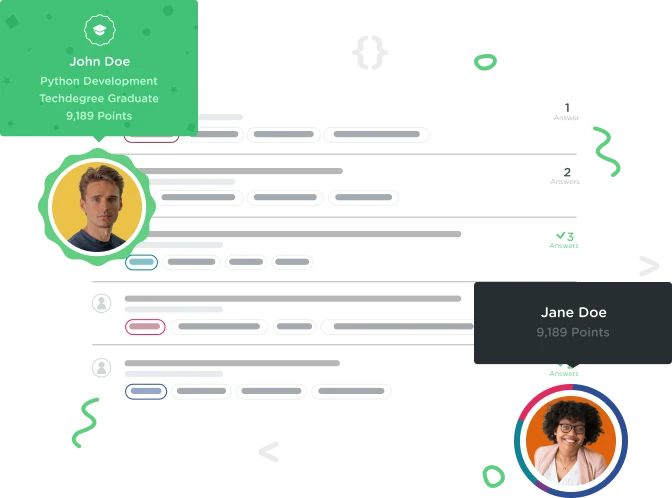

McKenzie Warren
5,698 PointsI'm just stuck on this override method...
First off, I was getting this error saying not all code paths return a value, which is why there's that random "return true;" underneath the foreach statement. But the foreach statement states that if an index of "sequence" == that index -1 (so the index immediately preceding it), it should return true, as is the prompt, right? Otherwise (else), it should return false. Does that not cover all code paths? or do I need to break out of the foreach statement as soon as it equates to true or something...?
Thanks in advance, guys!
namespace Treehouse.CodeChallenges
{
class SequenceDetector
{
public virtual bool Scan(int[] sequence)
{
return true;
}
}
}
namespace Treehouse.CodeChallenges
{
class RepeatDetector : SequenceDetector
{
public override bool Scan(int[] sequence)
{
foreach (int i in sequence)
{
if (sequence[i] == sequence[i-1])
{
return true;
}
else
{
return false;
}
}
return true;
}
}
}
1 Answer

Adam McGrade
26,333 PointsInside your foreach
loop, i
represents the value in the at the current index in sequence
rather than the index itself.
Imagine for example the sequence was [5,6,6]. In the first iteration of the loop, in your if
condition, you would be testing sequence[5] == sequence[5-1]
.
There is no value at index 5 or at index 4 as the array only contains 3 elements ( index 0, index 1, index 2). This would result in an exception being thrown.
In this instance it makes more sense to use a for
loop. This allows you to access the value at the current index sequence[index]
and compare it to the next value, at sequence[index + 1]
.
So you would loop through each element in the sequence from the start of the sequence to the second last value of the sequence (you cant compare the last value with a value at an index that is larger than the size of the array).
If the value at the current index is equal to the value at the next index, return true because there is a match.
By default you would return false, because you only want to return true, in the instance a match is found.
public override bool Scan(int[] sequence)
{
for (int i = 0; i < sequence.Length - 1; i++)
{
if (sequence[i] == sequence[i+1])
return true;
}
return false;
}
Hope that helps
McKenzie Warren
5,698 PointsMcKenzie Warren
5,698 PointsThanks, Adam!!!