Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial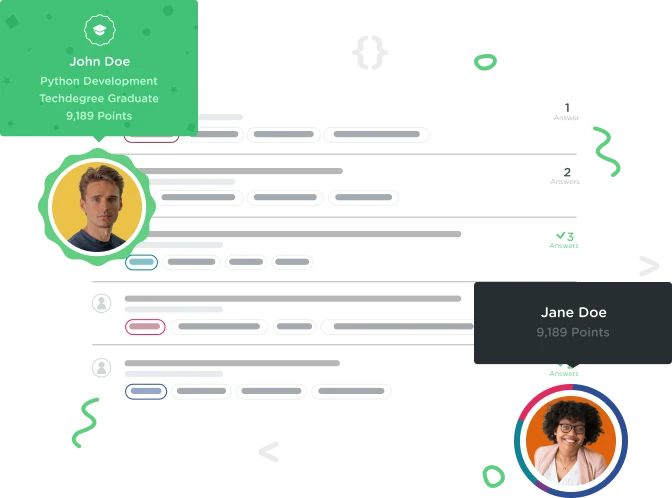
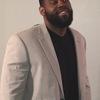
Willie Suggs
Courses Plus Student 5,879 Pointsim kinda lost, please help. i keep getting "your code takes to long to run" message
public void charge() {
mBarsCount = MAX_ENERGY_BARS;
mBarsCount++;
while (!isFullyCharged()) {
isFullyCharged();
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() { return mBarsCount == MAX_ENERGY_BARS; }
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_ENERGY_BARS;
mBarsCount++;
while (!isFullyCharged()) {
isFullyCharged();
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
}
}
3 Answers
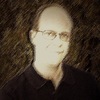
Jason Anders
Treehouse Moderator 145,858 PointsHey Kendra,
That kind of error means you've created an infinite loop. You're on the right track, but you have the incrementing line outside of the loop, so the value of mBarsCount will never increase in the view of the loop. There are also a couple of lines that shouldn't be there.
The line of code that was pre-loaded is no longer needed, so that can be deleted. The line of code that you have inside of the loop is calling the function, which doesn't need to be done.
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
So, you may have been over thinking this one. I posted the corrected method for your reference. I hope it makes sense. Keep Coding! :)

Simon Coates
28,694 Pointsyour loop just keeps running the test.
while (!isFullyCharged()) {
isFullyCharged();
}
loops need to make a change to something that controls the loop. Also for debugging purposes, you should probably get used to using something like eclipse. It would give you the ability to trace the program execution line by line, step into methods etc. You be able to observe the looping. If you need to locate an error and aren't running in an environment that supports tracing program execution, then you can use a debug library, or more simply System.out.println statements to get a view on what lines are executing and what values the variables hold. There are also a couple good approaches to debugging on paper. It helps to have an approach to debugging.
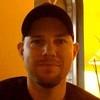
Jeremy Hill
29,567 PointsThe first thing you want to do is comment out or delete the first line of code that is in method charge(), it doesn't need to be there. Then you need to use the two elements that is asked of us in the challenge which is to use the '!' and the isFullyCharged() method so you could say while(! isFullyCharged()) {increment the mBarsCount} and that is all you need to do. If you are still confused on it I can paste the code for you to see. I hope this helps.