Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial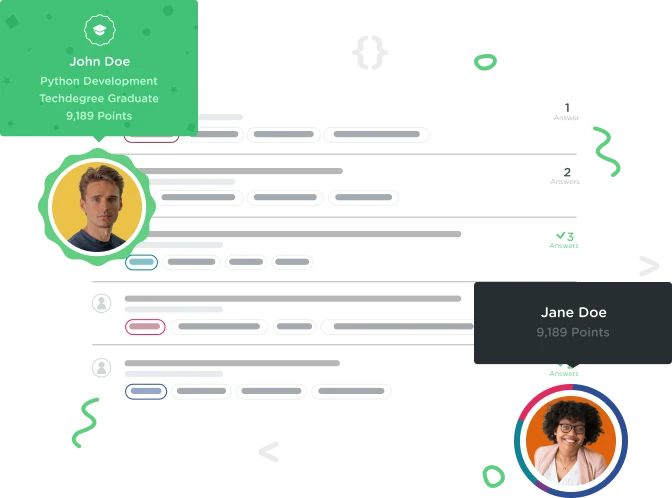

Adlan Ilyasov
2,476 PointsIm kinda stuck on this one.
Not sure what to replace "false" with.
const laws = document.getElementsByTagName('li');
const indexText = document.getElementById('boldIndex');
const button = document.getElementById('embolden');
button.addEventListener('click', (e) => {
const index = parseInt(indexText.value, 10);
for (let i = 0; i < laws.length; i += 1) {
let law = laws[i];
// replace 'false' with a correct test condition on the line below
if (false) {
law.style.fontWeight = 'bold';
} else {
law.style.fontWeight = 'normal';
}
}
});
<!DOCTYPE html>
<html>
<head>
<title>Newton's Laws</title>
</head>
<body>
<h1>Newton's Laws of Motion</h1>
<ul>
<li>An object in motion tends to stay in motion, unless acted on by an outside force.</li>
<li>Acceleration is dependent on the forces acting upon an object and the mass of the object.</li>
<li>For every action, there is an equal and opposite reaction.</li>
</ul>
<input type="text" id="boldIndex">
<button id="embolden">Embolden</button>
<script src="app.js"></script>
</body>
</html>
1 Answer
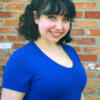
Bella Bradbury
Front End Web Development Techdegree Graduate 32,790 PointsHello Adlan!
The question is a little confusing, but it's essentially saying that there's a text field in the content of the site where we can enter the index value of one of the list items and it will make only the list item associated with the index that we entered stand out. i.e. If we entered 0 it would bold the first li item, 1 would be the second li item, and 2 would be the third. The numbers are one off because an index begins with 0 and not one.
In this challenge we're using a for
loop to iterate over each of the three li elements to see if any of their index's match the text field input. We only need to write the conditional statement, the rest is taken care of.
In the parameters for the for
loop, i
is set up to refer to each individual li item as the loop iterates. To be able to bold the correct list item of the three provided we'll need to write a conditional that checks if the value of the text input is equal to one of the list items' index. We'll use i
(which has already been established by the for
loop) to refer to the individual li items, then a strict equality operator (===
) to compare i
with the index
for each of the li items. All in all, the conditional we've build would be:
(i === index)
Hope this helps!