Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial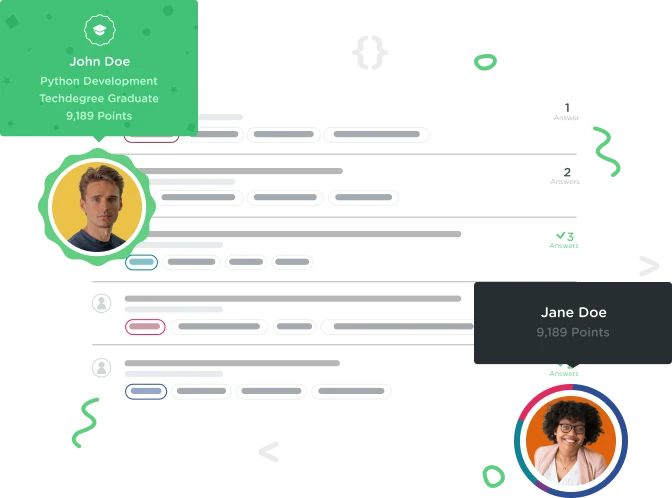
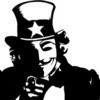
behar
10,799 PointsIm lost.
Cant figure it out, it says im using "unsafe" operations when im trying to create the TreeSet. I dont even know what that means. Help would be much appreciated!
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.List;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public TreeSet getAllAuthors() {
Set<String> authors = new TreeSet<String>();
for (BlogPost post : mPosts) {
authors.add(post);
}
return authors;
}
}
1 Answer

Manish Giri
16,266 PointsThere are a few problems in your code.
First, look at what you're asked to return in the challenge -
Create a method in the Blog class called getAllAuthors that loops over all the posts and returns a java.util.Set of all the authors
It says returns a java.util.Set. Whereas, in your method signature you have - public TreeSet getAllAuthors() {
. This returns a TreeSet
, not a Set
. So, you should change the signature to return Set<String>
instead.
Next, look at the second half of the instruction -
and returns a java.util.Set of all the authors, which are stored as Strings
Your Set
object should store all the authors which are stored as strings, meaning the names of the authors. So, you correctly declare your Set
object of type String
- Set<String> authors = new TreeSet<String>();
But in your for loop, you are adding a BlogPost
object, not a String
object -
for (BlogPost post : mPosts) {
authors.add(post);
}
HINT - BlogPost
class has a getAuthor()
method using which you can get the author name from the post
object.
And lastly, as for the "uncheck" warning, I think its due to the return of TreeSet
. TreeSet
is a raw type, meaning you lose the type safety which comes with generics when you use the correct type, like TreeSet<String>
, or TreeSet<Integer>
, etc. Java compiler warns you when you try to use raw types. If you fix the issue I mentioned in Step 1, that should take care of the warning.
Hope this helps.
behar
10,799 Pointsbehar
10,799 PointsHey again mate! Excellent explanation, and i think im close now. But i cant figure out you get loop thru the authors?
But the mPosts has no .getAuthor(). So yea, thats where im stuck, how do i get the authors??
Manish Giri
16,266 PointsManish Giri
16,266 PointsmPosts
is aList
object, so it wouldn't know whatgetAuthor()
is. It will only have access to methods defined in theList
interface injava.util.List
.I was referring to your earlier
for
loop itself -Here you're looping through the
List
ofBlogPost
objects, andpost
represents the objects in the loop. Now if you look in theBlogPost
class, you have this method, which returns the name of the author as aString
.So, every
BlogPost
object has access to this method, and you can use it in yourfor
loop to get the author's name add add it to theSet
, like so-Hope this helps.
behar
10,799 Pointsbehar
10,799 PointsGotcha, i dont know what i was thinking, i had some idea of looping thru the .getAuthor method. It didnt make any sense tho haha. Ty so much for your patience!