Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial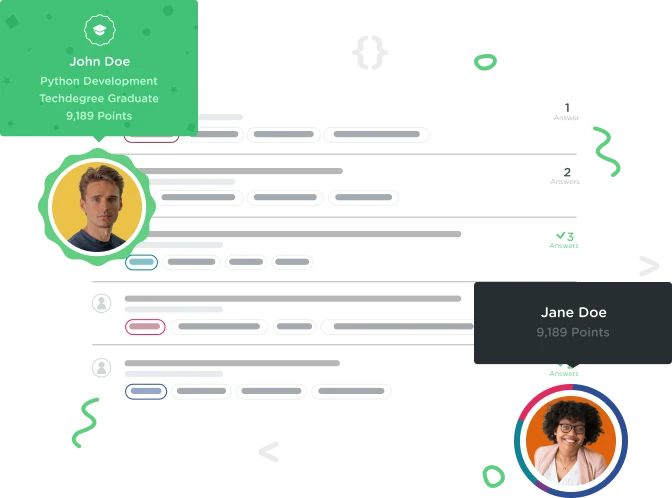

Cheri Castro
226 PointsI'm lost, my blood drive program isn't working correctly.
#Lab 10-3 Blood Drive
#the main function
def main():
endProgram = 'no'
while endProgram == 'no':
option = 0
print ('Enter 1 to enter in new data and store to file')
print ('Enter 2 to display data from the file')
option = int(input('Enter now ->'))
# declare variables
pints = [0] * 7
totalPints = 0
averagePints = 0
if option == 1:
# function calls
pints = getPints(pints)
totalPints = getTotal(pints, totalPints)
averagePints = getAverage(totalPints, averagePints)
writeToFile(averagePints, pints)
else:
readFromFile(averagePints, pints)
endProgram = input('Do you want to end program? (Enter no or yes): ')
while not (endProgram == 'yes' or endProgram == 'no'):
print ('Please enter a yes or no')
endProgram = input('Do you want to end program? (Enter no or yes): ')
#the getPints function
def getPints(pints):
counter = 0
while counter < 7:
pints[counter] = input('Enter pints collected: ')
counter = counter + 1
return pints
#the getTotal function
def getTotal(pints, totalPints):
counter = 0
while counter < 7:
totalPints = totalPints + pints[counter]
counter = counter + 1
return totalPints
#the getAverage function
def getAverage(totalPints, averagePints):
averagePints = int(float(totalPints) / 7)
return averagePints
#the writeToFile function
def writeToFile(averagePints, pints):
outFile = open('blood.txt', 'a')
print >> outFile, int('Pints Each Hour')
counter = 0
while counter <= 7:
outFile.write(str(pints[counter]) + '\n')
counter = int(counter + 1)
outFile.write('Average Pints')
outFile.write(str(averagePints) + '\n\n')
outFile.close()
#the readFromFile function
def readFromFile(averagePints, pints):
inFile = open('blood.txt', 'r')
str1 = inFile.read()
print (str1)
pints = inFile.read()
print (pints)
print #adds a blank line
averagePints = inFile.read()
print (averagePints)
inFile.close()
# calls main
main()
To me, the instructions were confusing, as well as, my instructor skipped giving us a lab, so perhaps Lab 9 should've been learned before Lab 10, to build on things. I think that I put most of these things in the right spots but, after I choose 1 or 2 and it asks me how many pints did I take in, it results in an error: Traceback (most recent call last): File "C:/Users/0wner/Desktop/Programming logic/Python Labs/Lab 10-3 test.py", line 80, in <module> main() File "C:/Users/0wner/Desktop/Programming logic/Python Labs/Lab 10-3 test.py", line 20, in main totalPints = getTotal(pints, totalPints) File "C:/Users/0wner/Desktop/Programming logic/Python Labs/Lab 10-3 test.py", line 44, in getTotal totalPints = totalPints + pints[counter] TypeError: unsupported operand type(s) for +: 'int' and 'str' So, I tried changing totalPints = ("totalPints" + "pints[counter]") which didn't fix the issue.I had also tried totalPints= int(totalPints + pints[counter]) I've worked through several other issues but haven't quite got this yet. I was so proud of myself yesterday, I did a whole lab all by myself without having to ask for any help. I recognized and worked through all my mistakes all by myself. Today, not so much.