Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial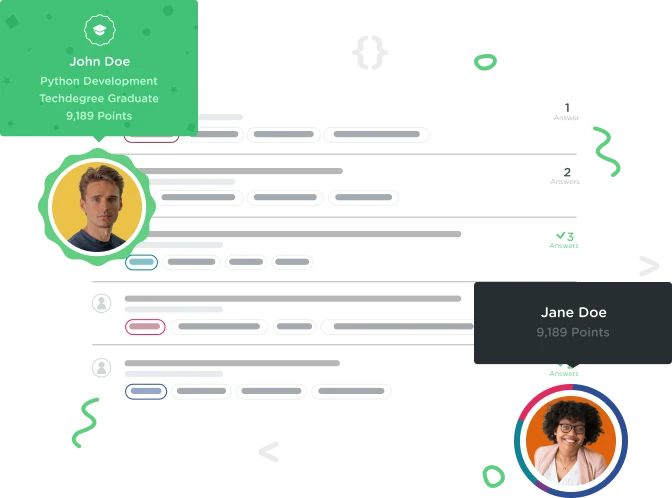
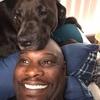
Corey Draper
3,452 PointsI'm lost on this one
I'm stuck on selecting multiple elements.
var listItems = document.getElementByTagName('ul');
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
var collection = document.getElementById('#rainbow');
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
<!DOCTYPE html>
<html>
<head>
<title>Rainbow!</title>
</head>
<body>
<ul id="rainbow">
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
<script src="js/app.js"></script>
</body>
</html>
3 Answers
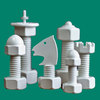
Steven Parker
229,744 PointsFirst, there's no "getElementByTagName" method. There is a "getElementsByTagName" (plural) method, but you also need to restrict the set to a particular list.
The challenge wants you to set the variable listItems
to refer to a collection of "all list items in the <ul> element with the ID of rainbow". So we know the target needs to be list item elements (li), and we need to select them all but restrict the selection to just those within the "rainbow" list. So you could do this:
var listItems = document.getElementById('rainbow').getElementsByTagName("li");
Like many things in programming, that's not the only correct solution, and a more efficient approach is to use "querySelectorAll" with a descendant selector:
var listItems = document.querySelectorAll('#rainbow li');

David Weissman
5,051 PointsI tried about 12 different permutations of querySelectorAll, but based on the Guil's video, there was no way to know the syntax for doing it for an unordered list with a particular ID, since the examples in the video were referring to classes (.error-not-purple) and the nth-child of a list. Is there some kind of summary of the syntax rules for this? The confusion is that the syntax seems to be different depending on exactly what you're looking for, and there was no way to intuit this without some sort of general guidelines. This is really one of the first lessons in the whole front end development track that has really not explained things clearly.
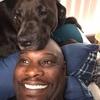
Corey Draper
3,452 PointsThank you. I was way off. I tried different approaches.