Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial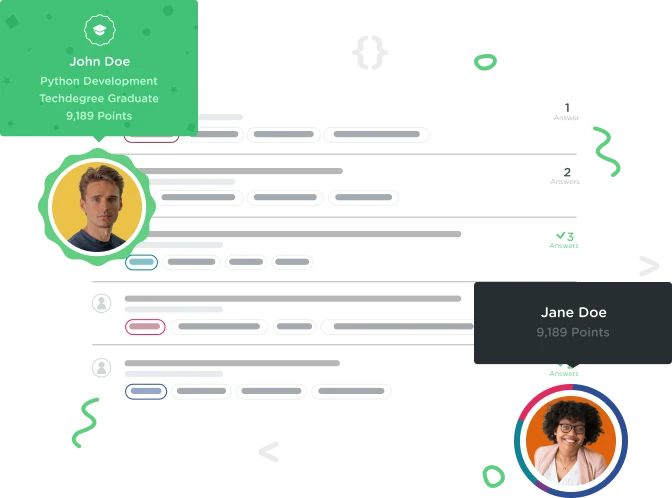

Rodrigo Gomez-Palacio
1,125 PointsI'm lost on what the purpose of all of this is. Why am I creating another method with the same name?
I have several questions:
Why is it necessary to put an "m" before certain methods and not others?
Why do I have to create a new method with the same name "drive" and no arguments? What is the purpose of this? I don't know what to put within this method because I don't know what I'm trying to achieve in this exercise.
Thanks
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive(){
mBarsCount = 1;
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
5 Answers

Craig Dennis
Treehouse TeacherHi Rodrigo,
The m
prefix is for member variables. This is a coding style used to help distinguish between local variables and class variables. While not required. it is the style we are sticking to during the beginning Java courses, and what you will encounter as/if you get into Android development.
Creating a new method with the same name but different arguments is creating a new method signature. This allows you to overload methods and basically set defaults. In this case you should call the original drive
method and pass in a one. This way users of the class can just call drive
and it will default to 1 OR they can choose how many laps to drive.
Does that clear things up?

Alandus James
Courses Plus Student 597 PointsI'm having a hard time with understanding calling the original method and passing a one to it. I'm not asking for just the answer I want to understand why its the correct answer. Do I need to make laps as a class variable then add it to the method. This is what i have so far and I'm sure its wrong.
public void drive() { drive(laps++)
}

Craig Dennis
Treehouse TeacherThe idea here is that you can create a default value. So now there are two methods:
drive()
which takes no params
and drive(laps)
which takes the number of laps
If you call the drive()
with no params it drives the default value of 1. The way it does that is by calling the drive(laps)
and passing in the default value, which is 1.
More clear?

Alandus James
Courses Plus Student 597 PointsThis might be incorrect but I think this is how i understand it. When I write this :
public void drive() { drive(1); }
The 1 is replacing laps in this method : public void drive(int laps) {}
I'm getting confused on what you mean by passing the default value.

Craig Dennis
Treehouse TeacherSorry...the idea is we want the method drive()
with no parameters to just assume it drives 1 lap, as we think that is the most common usage. Yes it is simply just calling the other method, and passing it the assumption that we want, but the caller doesn't need to think about it.
Yes you are correct in your thought process. This is called method overloading.

Rodrigo Gomez-Palacio
1,125 PointsI accidentally tampered with the code that was added by the program and I can't remember what it was. I recommend Treehouse add a "reset" option to be able to start from scratch on an exercise. Anyhow, can someone help me by providing the original code?

ISAIAH S
1,409 PointsHere's the original code:
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}