Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial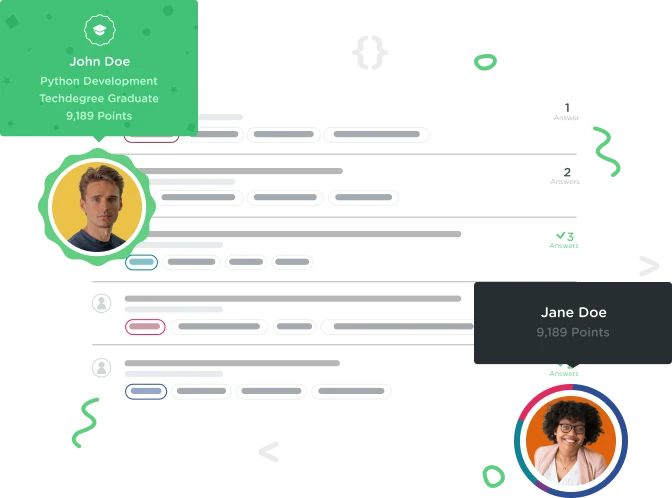

Jaynesh Khatri
398 PointsI'm making a Rock Paper Scissors experiment in JavaScript. I don't know what I'm doing wrong. Please Help. The code
console.log("Video Game");
var playerOne = prompt("Player One R=Rock P=Paper S=Scissors");
var playerTwo = prompt("Player Two R=Rock P=Paper S=Scissors");
//Combinations for Ties
if ((playerOne = "r"||"R") && (playerTwo = "r"||"R")) {
console.log("Tie");
}
else if ((playerOne = "p"||"P") && (playerTwo = "p"||"P")) {
console.log("Tie");
}
else if ((playerOne = "s"||"S") && (playerTwo = "s"||"S")) {
console.log("Tie");
}
//Different Combinations to victory
else if ((playerOne = "r"||"R") && (playerTwo = "s"||"S")) {
console.log("Player One Wins!!!!");
}
else if ((playerOne = "s"||"S") && (playerTwo = "r"||"R")) {
console.log("Player Two Wins!!!!");
}
else if ((playerOne = "P"||"p") && (playerTwo = "r"||"R")) {
console.log("Player One Wins!!!!");
}
else if ((playerOne = "r"||"R") && (playerTwo = "p"||"P")){
console.log("Player Two Wins");
}
else if ((playerOne = "s"||"S") && (playerTwo = "p"||"P")){
console.log("Player One Wins!!!!");
}
else if ((playerOne = "P"||"p") && (playerTwo = "s"||"S")){
console.log("Player Two Wins!!!!");
}
2 Answers
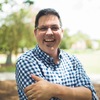
Dave McFarland
Treehouse TeacherThis won't work:
playerOne = "r"||"R"
That code doesn't test the value of the input, it assigns a new value to the playerOne
variable.
To test to if the player typed 'r' or 'R' you need to use the ===
operator and you need to use the || operator between 2 different conditions, like this:
playerOne==='r' || playerOne==='R'
Or even better:
playerOne.toUpperCase() === 'R'
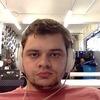
Iago Wandalsen Prates
21,699 PointsAlso, some possible improvement you could do: instead checking if both users are rock, and than saying its a tie, you could just check if playerOne is equal to playerTwo, and save two if statements.
Nesting conditionals will make your code more readable, and executing faster. Instead of checking every single time what are both the inputs, you can first check the first input, and inside it, check for one of the possible inputs (draws have been handled already), and if it checks, give that result, else you give the other result.
Other than that, good job on learning JavaScript, its an awesome language.
Iago Wandalsen Prates
21,699 PointsIago Wandalsen Prates
21,699 PointsIt is a valid javascript code, it just doesn't do what he wants.
playerOne = 'r' || 'R' will evaluate if 'r' is a truthy value, and as it is, it will assign 'r' to player one.
Dave McFarland
Treehouse TeacherDave McFarland
Treehouse TeacherThanks Iago Wandalsen Prates for pointing that out. Yes, it is valid -- in other words it won't generate a syntax error. It's just not correct for what he wants to do. I've updated my response to be more accurate. Thanks!
James Barnett
39,199 PointsJames Barnett
39,199 PointsPesky logic bugs
Jaynesh Khatri
398 PointsJaynesh Khatri
398 PointsThanks for the help