Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial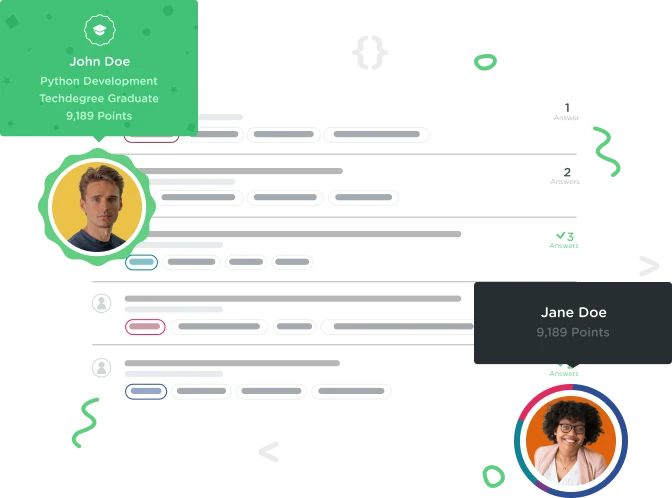

mrx3
8,742 PointsI'm new to JavaScript and I have some questions about Methods, Events, Objects, and Properties.
I picked up two books from Barnes and Nobel bookstore awhile ago. One book is JavaScript and Jquery The Missing Manual and the other is Javascript & Jquery by Jon Duckett. They are both great books, but I got lost in the Jon Duckett book in the first chapter. I kinda of understand that Javascript works with "objects", and that objects are things like buttons, form fields, and a document window. Events are things that happen when we "interact with certain web elements." I could be way off on my definitions. I don't understand what methods are, or properties. I'm a little ways away from the front-end development track. I have made about 50 websites of my own, all responsive, and stored on my computer. I wanted to start to practice making my sites interactive. Most of my sites are static, and I kinda want to make them interactive. So if someone could give a little help with what methods, events, objects, and properties are, I would really appreciate it. My last question is do the JavaScript courses on treehouse go into detail about methods, events, objects, and properties. Thank you again in advance for any help.
2 Answers
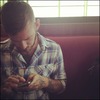
Erik McClintock
45,783 PointsAs Victor mentions, the courses here on Treehouse are a great place to start. Additionally, if you're looking for a book that will give you simpler, more basic examples and clearer definitions, check out Head First JavaScript Programming from O'Reilly Media. Their Head First series is fantastic, and gives great examples, definitions, uses a lot of images, quizzes, puzzles, project ideas, etc. to really help you cement things in your head.
As far as a quick rundown of these concepts, though (very high level), see below:
You can think of an OBJECT as just that: any real world object. For this example, let's say our object is an animal. Now, as you now, animals have many different distinct features, and (depending on the animal) they can perform any number of activities. Those features of the animal are its PROPERTIES, and the activities that it can perform are its METHODS. If you're familiar with a FUNCTION at all, you can realize that a method is simply a function that belongs specifically to an object.
So, let's take a look at an example of our animal object (which is just a collection of properties and methods):
// "cat" is the name of our animal object
var cat = {
// inside of our "cat" object, we see it has a few properties that define who/what it is, in this case name, age, fur color, and what his status is
name: "Whiskers",
age: 4,
furColor: "black",
status = "awake",
// we also see that this cat knows how to meow and how to sleep; these are its methods, or the activities that it can perform
meow: function() {
// this alert statement would pop up a JavaScript alert box that would say "Hello, my name is Whiskers." inside of it)
alert("Hello, my name is " + this.name + ".");
},
sleep: function() {
// this function would set the value of Whiskers' "status" variable equal to "asleep"
this.status = "asleep";
}
}
So, if someone wanted to know some information about this cat object, you would simply let them know by calling the property that said person was interested in. Say they wanted to know your cat's name? You would write the following:
// this would output "My cat's name is Whiskers.", by calling the "name" property of the "cat" object
alert("My cat's name is " + cat.name + ".");
If they wanted to know what color fur Whiskers has?
// this would output "Whiskers has black fur.", by calling the "furColor" property of the "cat" object (as well as getting his name at the beginning of it)
alert(cat.name + " has " + cat.furColor + " fur.");
And if they wanted to know how old he was, and if he was awake?
// this would output "Whiskers is 4 years old and is currently awake."
alert(cat.name + " is " + cat.age + " years old and is currently " + cat.status + ".");
Now, if you wanted to your cat to tell people his name on his own, you would simply call the method that he has (i.e. the activity that he knows how to perform) to make him do just that:
// this would create a JavaScript alert that would read "Hello, my name is Whiskers.", because that's what we defined inside the "meow" method
cat.meow();
And if you wanted Whiskers to go to sleep, you would make that happen by calling his "sleep" method:
// this would set the cat's "status" property equal to the value of "asleep", because that's what we defined inside the "sleep" method
cat.sleep();
The syntax make look odd if you're unfamiliar with programming languages, but hopefully this helps to give you a slightly better picture of what these different terms mean. Just remember that an object can be thought to represent an actual object, and the qualities of that object are its properties, and its abilities or activities that it can perform are its methods.
An event is when the user does something in the environment, like clicking their mouse, scrolling the page, or pressing a key on their keyboard.
Hope this helps!
Happy coding!
Erik
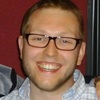
Victor Learned
6,976 PointsI would highly recommend you start going through the Javascript lessons from the beginning. Reading books like that are great but if you don't understand the foundation (properties, methods, objects) you will just continue down the rabbit hole and be confused. The lessons on Treehouse do cover all these things in a clear fashion so I think once you go through all of your questions above should be answered.

mrx3
8,742 PointsThanks Victor. I believe you're right.
mrx3
8,742 Pointsmrx3
8,742 PointsThanks Erik. I will defiantly look at the book you mentioned.
mrx3
8,742 Pointsmrx3
8,742 PointsGot the Book Erik. I have only read the first 15 pages. I'm practicing on making inline-block layouts with Guil, so I've been extremely busy. Thank you again for your suggestion and help. You also got the best answer. Take care.
Erik McClintock
45,783 PointsErik McClintock
45,783 PointsFantastic! Let me know how you like the book; I have found that series to be immensely helpful across a wide range of topics, including JavaScript (and jQuery). Additionally, I find that it's always nice to learn the same information from a variety of resources so you have the chance to learn it in different ways and with different examples.
Inline-block layouts are a great tool to have in your arsenal, and are also how I choose to do layouts now over using floats (most of the time).
Let me know if you need anything else!
Erik