Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial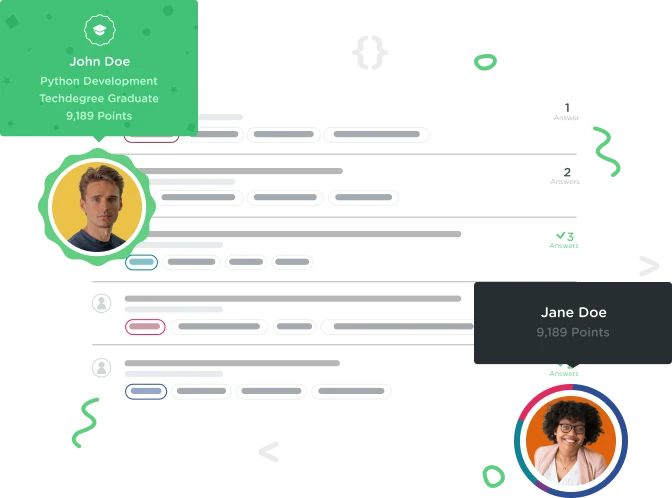
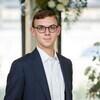
akiva grobman
9,160 PointsI'm not getting any specific error message only "Try again!".
I'm not getting any specific error message only "Try again!", I can't find the problem, any ideas? Thanks in advance.
import random
class Die:
def __init__(self, sides=2):
if sides < 2:
raise ValueError("Can't have fewer than two sides")
self.sides = sides
self.value = random.randint(1, sides)
def __int__(self):
return self.value
def __add__(self, other):
return int(self) + other
def __radd__(self, other):
return self + other
class D20(Die):
def __init__(self):
super().__init__(sides=20)
from dice import D20
class Hand(list):
def __init__():
super().__init__()
@property
def total(self):
return sum(self)
@classmethod
def roll(cls, num_of_dice):
h = Hand()
for _ in len(num_of_dice):
h.append(D20())
retrun h
2 Answers
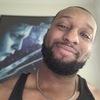
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsHi akiva,
I’m seeing too small errors, both of which are easy to skip over, so no fret.
The first is, in your for loop in hands.py you’re trying to get the len
of a number when you should be using the range
function here. So instead of len(num_of_dice)
try range(num_of_dice)
.
That said you would have figured that out on your own if not for the other small error. You’ve spelled return
incorrectly. So a hand object is not actually being returned from the roll class method right now. Fix those two things and you’ll be good to go.
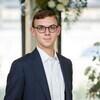
akiva grobman
9,160 PointsThis is the code, I'm getting a "Can't get the length of a `Hand" message. Thanks.
from dice import D20
class Hand(list):
def __init__():
super().__init__()
@property
def total(self):
return sum(self)
@classmethod
def roll(cls, num_of_dice):
h = Hand()
for _ in range(num_of_dice):
h.append(D20())
return h
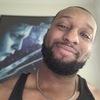
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsOK akiva,
So the error is being caused by something in the superclass’s init method. It didn’t pop up for me originally because when I copy/pasted your code into the challenge the first thing I did was remove the init method you added (because it wasn’t a part of the instructions). I didn’t mention that to you because I didn’t think it would affect your code in any functional way (and I didn’t want to tell you to remove the init method simply because it wasn’t necessary to this particular challenge).
As it turns out though, the list class’s init method seems to be passing the instantiated Hand object into the len function, and there’s nothing that tells the python interpreter how to deal with that. So the program is crashing.
Remove the init method, or at least switch out: super().__init__()
for pass
. That should get you moving forward.
akiva grobman
9,160 Pointsakiva grobman
9,160 PointsThank you very much, I have made those changes and I'm now getting a new error "Can't get the length of a
Hand
".Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsBrandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsCan you post a copy of your code? Based on the error it sounds like you’re attempting to pass a Hand object as an argument into the
len
function. So I’d need to see that to evaluate it.That said, you don’t need to use the
len
function at all for either of the two challenges.