Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial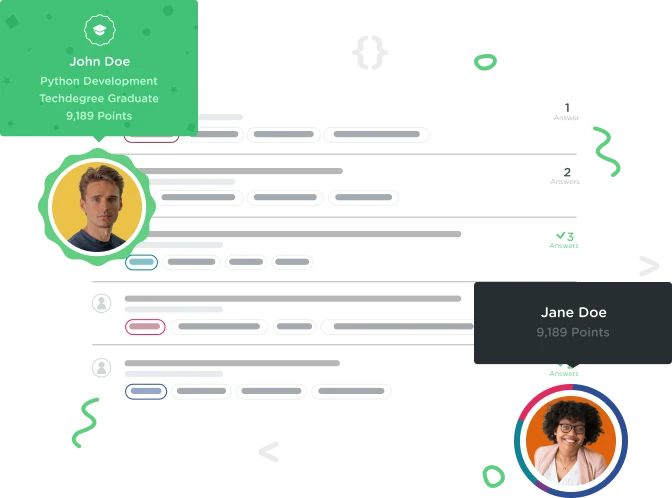
Gary Christie
5,151 PointsI'm Not getting it
The challenge question is "The identical() method is being called but the variables are not identical. See if you can fix the code."
This is what is in the code"
var myUndefinedVariable;
var myNullVariable= null;
if(myNullVariable == myUndefinedVariable) {
identical ();
}
I'm not really understanding this at all.
5 Answers

Ken Alger
Treehouse TeacherGary;
The other somewhat difficult concept to grasp in this challenge, and topic overall, is the difference between undefined and null in JavaScript. The myNullVariable
, in this example, was given a value of "null", so when JavaScript goes to look for a value it finds one. The myUndefinedVariable
is not given a value, so when JavaScript goes to look for a value for it it cannot find one, therefore it should be expected to give a "not found exception" or some other way of presenting a state of "not foundness." In JavaScript that is equivalent to undefined.
The identity (===) operator behaves identically to the equality (==) operator except no type conversion is done, and the types must be the same to be considered equal.
Reference: Javascript Tutorial: Comparison Operators
The == operator will compare for equality after doing any necessary type conversions. The === operator will not do the conversion, so if two values are not the same type === will simply return false. It's this case where === will be faster, and may return a different result than ==. In all other cases performance will be the same.
Hopefully that helps.
Ken
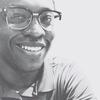
Ricardo Hill-Henry
38,442 PointsThe problem lies with the key difference between == and ===. Javascript does automatic type conversion when comparing to things using ==. This means something like:
var one = "1";
if (one == 1){
//do something
}
would be true, and the code would execute. Values like undefined, null, 0 and I believe false, are consider equal when using the == comparison operator. It's actually a good idea to always use === which is what the challenge wants. This is one of those things in Javascript that you should definitely note, or else you may experience some bugs down the road.
if(myNullVariable === myUndefinedVariable) {
identical();
}

Chris Shaw
26,676 PointsValues like undefined, null, 0 and I believe false, are consider equal when using the == comparison operator.
That's partially correct, null
and undefined
are the same as well as 0
and false
but they they all don't with equal one another with the exception of null
and undefined
, 0
and false
when using the double equals. See the below
null == false // false
null == undefined // true
undefined == false // false
false == 0 // true
0 == undefined // false
0 == null // false
However when using the strict type and value comparison operator all of the above changes to false.
null === false // false
null === undefined // false
undefined === false // false
false === 0 // false
0 === undefined // false
0 === null // false
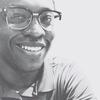
Ricardo Hill-Henry
38,442 PointsYeah, I was iffy about 0 and false when comparing them to undefined and null, since undefined and null work on objects, while 0 and false are usually used for values or data types. Thanks for clearing that up.

Chris Shaw
26,676 Pointssince undefined and null work on objects
I think you're confusing JavaScript with another language as both undefined and null can't be compared to an Array
nor an Object
.
({}) == null // false
({}) == undefined // false
[] == null // false
[] == undefined // false
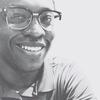
Ricardo Hill-Henry
38,442 PointsLooks like I need to go pick up a Javascript book again.

Chris Shaw
26,676 PointsThe === operator will not do the conversion, so if two values are not the same type === will simply return false
You're almost there, the triple equals does both a type and value comparison, there would be no point to just comparing the two types as then we could put any values on the left and right side of the operator of the same type and get a pass.

Ken Alger
Treehouse TeacherChris;
Right, it compares both type and value. What I was saying is that as it is checking if the types aren't the same, it doesn't care what the values are. If the types are the same, then it checks the values. Not sure what the last part of your statement is referring to.
Gary Christie
5,151 PointsKen, the way you answered my question seems like I'm suppose to get it. I think I'm just tired or something. Its just not registering to me. I'm looking at Chris and Ricardo's conversation and its making realize I'm completely lost. I'm going to watch the video like 10 more times

Chris Shaw
26,676 PointsThe most simple way I can answer the question is by the following.
-
==
will always match two variables together regardless of whether they have the same type -
===
will never match two variables unless they both have the same type and value
Gary Christie
5,151 PointsI think I get you Chris. == would give two vars that are falsey's a True outcome, and === will give two vars that are falsey's a false outcome because the === knows that there are two different vars although they are both falsey's.such as undefined vs a null. I hope that made sense to you.