Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial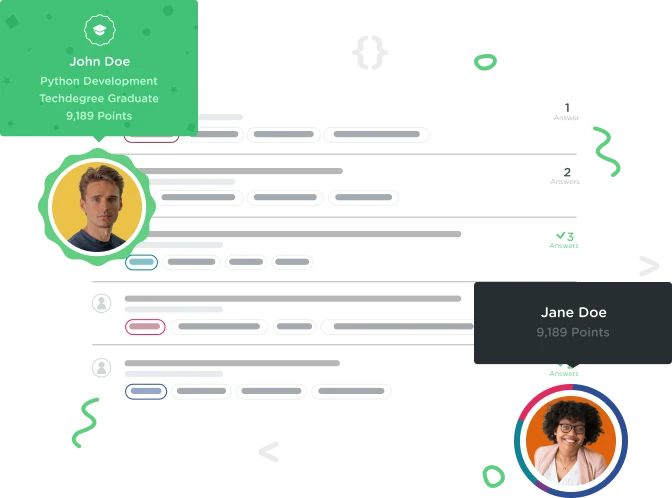

Dustin Shelley
Courses Plus Student 4,608 PointsI'm not getting JSON data.
I'm not getting any JSON data. Its running and making API calls just not returning any data. Any suggestions?
import UIKit
class ViewController: UIViewController {
private let apiKey = "010101010110"
override func viewDidLoad() {
super.viewDidLoad()
let baseURL = NSURL(string:"https://api.forecast.io/forecast/\(apiKey)/")
let forecastURL = NSURL ( string: "33.3777503,-84.763111,13", relativeToURL: baseURL)
let sharedSession = NSURLSession.sharedSession()
let downloadTask: NSURLSessionDownloadTask = sharedSession.downloadTaskWithURL(forecastURL!, completionHandler: { (location: NSURL!, response: NSURLResponse!, error: NSError!) -> Void in
var urlContents = NSString(contentsOfURL: NSURL(), encoding: UInt(), error: NSErrorPointer())
println(urlContents)
})
downloadTask.resume()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
}
}
3 Answers

Stone Preston
42,016 Pointsyou have too many coordinates in your URL. you should only have 2.
// you provided 3 coords instead of 2. remove the ,13:
let forecastURL = NSURL ( string: "33.3777503,-84.763111,13", relativeToURL: baseURL)
remove that last one
let forecastURL = NSURL ( string: "33.3777503,-84.763111", relativeToURL: baseURL)
or remove the comma if its supposed to be part of the last coord
let forecastURL = NSURL ( string: "33.3777503,-84.76311113", relativeToURL: baseURL)

Dustin Shelley
Courses Plus Student 4,608 PointsThanks for catching that. Closed and opened Xcode again, fixed coordinates. Now I'm getting "fatal error: unexpectedly found nil while unwrapping an Optional value" in this line.
let downloadTask: NSURLSessionDownloadTask = sharedSession.downloadTaskWithURL(forecastURL!, completionHandler: { (location: NSURL!, response: NSURLResponse!, error: NSError!) -> Void in

Stone Preston
42,016 Pointspost your new code after modifying the URL

Dustin Shelley
Courses Plus Student 4,608 Pointsimport UIKit
class ViewController: UIViewController {
private let apiKey = "0101010"
override func viewDidLoad() {
super.viewDidLoad()
let baseURL = NSURL(string:"https://api.forecast.io/forecast/\(apiKey)/")
let forecastURL = NSURL ( string: "33.378109, -84.799675", relativeToURL: baseURL)
let sharedSession = NSURLSession.sharedSession()
let downloadTask: NSURLSessionDownloadTask = sharedSession.downloadTaskWithURL(forecastURL!, completionHandler: { (location: NSURL!, response: NSURLResponse!, error: NSError!) -> Void in
var urlContents = NSString(contentsOfURL: NSURL(), encoding: UInt(), error: NSErrorPointer())
println(urlContents)
})
downloadTask.resume()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
}
}

Stone Preston
42,016 Pointsremove the space between the coords. URLs cant have spaces:
let forecastURL = NSURL ( string: "33.378109,-84.799675", relativeToURL: baseURL)

Dustin Shelley
Courses Plus Student 4,608 PointsFixed that. Sorry copying and pasting coordinates is giving me problems. Still no Json data anywhere. Debug output shows nil. Simulator is white.

Stone Preston
42,016 Pointsits probably nil because of this:
var urlContents = NSString(contentsOfURL: NSURL(), encoding: UInt(), error: NSErrorPointer())
the URL you are passing in is NSURL() which is just an empty URL. try passing in the location URL instead:
var urlContents = NSString(contentsOfURL: location, encoding: UInt(), error: NSErrorPointer())