Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial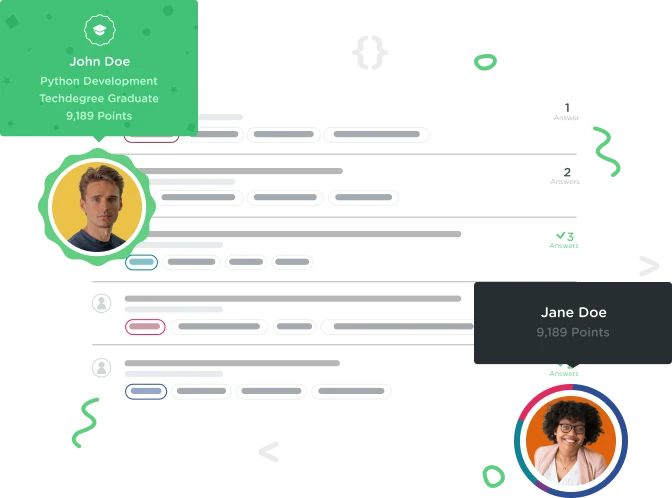

Joshua Faisal
4,493 PointsIm not getting the same output as Megan here in the terminal. What's wrong with my code? Any help is appreciated.
Megan is running lion_log.animal and is getting a good ouput and im just getting an error. I need help figuring out what's wrong and debugging. The part of the video where Megan runs lion_log.animal is at 4:35 https://teamtreehouse.com/library/relational-databases-with-sqlalchemy/the-c-and-r-of-crud#notes
Here is my code:
#animals
#ID/Name/Habitat/Size
#zookeeper log
#ID/Animal ID (foreign Key)/notes
from sqlalchemy import create_engine, Column, Integer, String, Integer, String, ForeignKey
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker, relationship
engine = create_engine("sqlite:///zoo.db", echo = False)
Session = sessionmaker(bind=engine)
session = Session()
Base = declarative_base()
class Animal(Base):
__tablename__ = "animals"
id = Column(Integer, primary_key=True)
name = Column(String)
habitat = Column(String)
size = Column(String)
logs = relationship("Logbook", back_populates="animal")
#name of class when doing reltionship
#what this does is create a connection between logs atribute and animal atribute when on log and you select animal attr it wil give you the animal that relates to the foreign ID.
#and when you are on animal table when u sleect log attr its going to go to the log book and find all the notes relating to this ID and put it in a list for you
def __repr__(self):
return f'''
\nAnimal {self.id}\r
Name= {self.animal_id}\r
Habitat = {self.notes}\r
Size = {self.size}
'''
class Logbook(Base):
__tablename__ = "logbook"
id = Column(Integer, primary_key=True)
animal_id = Column(Integer, ForeignKey("animals.id"))
#this animal.id will be like id when created it will be a column of IDs from the animal table so it shows ID and can match with animal from animal class
notes = Column(String)
animal = relationship("Animal", back_populates = "logs")
def __repr__(self):
return f'''
\nLogbook {self.id}\r
Animal ID = {self.animal_id}\r
Notes = {self.notes}
'''
if __name__ == "__main__":
Base.metadata.create_all(engine)
heres my terminal:
Python 3.11.4 (tags/v3.11.4:d2340ef, Jun 7 2023, 05:45:37) [MSC v.1934 64 bit (AMD64)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>> import models
>>> lion = models.Animal(name="lion", habitat="savannah")
>>> lion.name
'lion'
>>> lion.id
>>> models.session.add(lion)
>>> models.session.commit()
>>> lion.id
2
>>> tiger.id
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
NameError: name 'tiger' is not defined. Did you mean: 'iter'?
>>> lion_log = models.Logbook(animal_id=2, notes='great pouncer')
>>> models.session.add(lion_log)
>>> models.session.commit()
>>> lion_log.id
2
>>> lion_log.animal
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "C:\Users\18329\Downloads\SQLALCH-Relational-DB\models.py", line 32, in __repr__
Name= {self.animal_id}\r
^^^^^^^^^^^^^^
AttributeError: 'Animal' object has no attribute 'animal_id'
>>> lion_log.Animal
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: 'Logbook' object has no attribute 'Animal'. Did you mean: 'animal'?
>>>
1 Answer
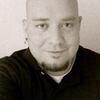
Brian Jensen
Treehouse StaffHiya, Joshua Faisal 👋
The issue appears to be in your dunder repr for the Animal class. Currently, you have this:
def __repr__(self):
return f'''
\nAnimal {self.id}\r
Name= {self.animal_id}\r
Habitat = {self.notes}\r
Size = {self.size}
'''
The error is thrown because self.animal_id
is not a valid attribute of the Animal class. You will instead want to use Name = {self.name}
.
On a side note, there is also an issue with Habitat = {self.notes}
, you will instead want it to be Habitat = {self.habitat}
I hope that helps you get back on track!
Joshua Faisal
4,493 PointsJoshua Faisal
4,493 PointsFinally got it. Thanks a lot