Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial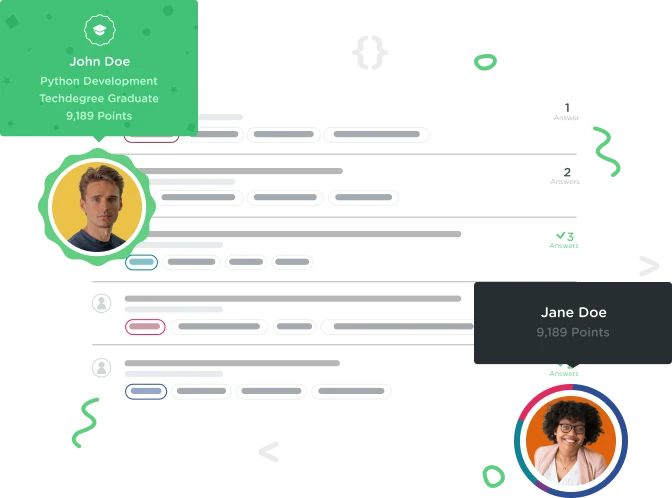

Austin Greed
Python Web Development Techdegree Student 1,966 PointsI'm not getting where i am wrong..plz help me
question is to refactor the code by adding the function main
def show_help():
# print out instructions on how to use the app
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see your current list.
""")
def show_list(shopping_list):
# print out the list
print("Here's your list:")
for item in shopping_list:
print(item)
def add_to_list(shopping_list, new_item):
# add new items to our list
while True:
# ask for new items
new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
else new_item == 'SHOW':
show_list(shopping_list)
continue
shopping_list.append(new_item)
print("Added {}. List now has {} items.".format(new_item, len(shopping_list)))
return shopping_list
def main():
show_help()
# make a list to hold onto our items
shopping_list = []
add_to_list(shopping_list, new_item)
show_list(shopping_list)
main()
2 Answers
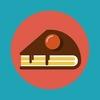
tc11
19,518 PointsHey Austin,
The main function has to be properly placed on line 22, and then you must properly indent the rest of the code into the main function.
This should be your final output:
#Beginning of main on line 22
def main():
# rest of the code indented at least 4 spaces into the main function.
show_help()
# make a list to hold onto our items
shopping_list = []
while True:
# ask for new items
new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list(shopping_list)
continue
add_to_list(shopping_list, new_item)
show_list(shopping_list)
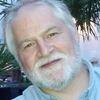
Jeff Muday
Treehouse Moderator 28,717 PointsYou are so close! When you encapsulated the shopping_list = [] in the main() function, you effectively made it a local variable. That's an ok design choice. But it could also be global in scope (e.g. move it to the top of the program).
There are so many ways to program python, so bear in mind what I show here isn't the only good way to do it. It's all up to you to make the design choices YOU like!
First, we have to take advantage of the loop and indent two lines at the bottom, so they are in the loop:
def add_to_list(shopping_list, new_item):
# add new items to our list
while True:
# ask for new items
new_item = input("> ")
# be able to quit the app
if new_item.upper() == 'DONE':
break
elif new_item.upper() == 'HELP':
show_help()
continue
elif new_item.upper() == 'SHOW':
# changed from else to elif so we can evaluate new_item
show_list(shopping_list)
else:
# this else and the indentation allows us to add the item and print a message for user
shopping_list.append(new_item)
print("Added {}. List now has {} items.".format(new_item, len(shopping_list)))
return shopping_list
Here, we can just take advantage that add_to_list() returns the value of the completed shopping list
def main():
show_help()
# make a list to hold onto our items
shopping_list = []
shopping_list = add_to_list(shopping_list, new_item)
show_list(shopping_list)
You could even simplify it this way too:
def main():
show_help()
shopping_list = add_to_list( [], new_item )
show_list(shopping_list)