Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial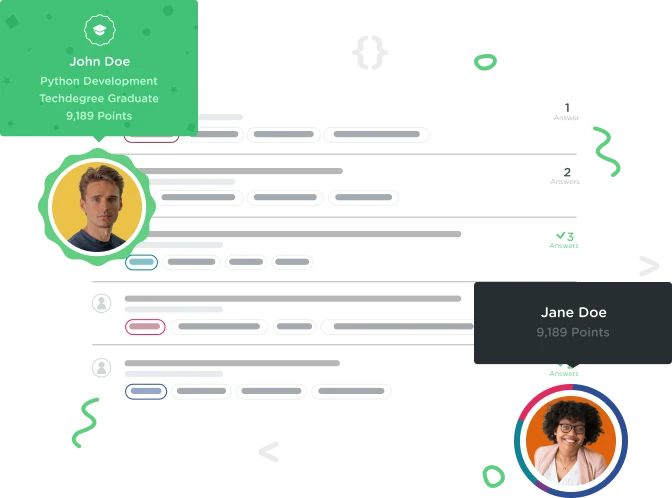

ms28
Python Web Development Techdegree Student 1,067 PointsI'm not quite understanding how this works... The try thing. I'll google around though.
Is try a if statement in disguise?
3 Answers
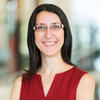
Louise St. Germain
19,424 PointsHi Matt,
I suppose a try statement is at least a cousin of the if statement! :-) It does have an extra beginning section that the if doesn't have, though.
Here's a regular if statement:
# -------------------------
# Normal if statement
# -------------------------
if ("cupcake" == 42):
# Then do whatever is in this block, but only if that condition ("cupcake" == 42) is true.
# Otherwise, skip the stuff in this section.
The thing about exceptions is that if they aren't handled properly, they just immediately grind the whole program to a halt. So let's say you tried to use a normal if statement to deal with a ValueError using something like this:
my_integer = int("cupcake")
if (my_integer == ValueError): # or something... This isn't the right way to handle exceptions so I'm making stuff up
print("What kind of integer is that?")
Problem is, the int("cupcake") will generate a ValueError and immediately stop the program, so the program dies before getting to the next if statement where you attempt to handle the situation.
Instead, the try block is there to say, hey, I know this may cause a problem, but if it does, hang on - don't stop the program, give me a chance to deal with it! Like so:
# -------------------------
# Try block
# -------------------------
# (The normal if statement doesn't have this first section.)
# Start by warning Python, hey, I know this next bit might cause an issue. But I have a plan!
# If anything in this try block throws an exception, see below for how to handle it.
try:
my_integer = int("cupcake")
# This is kind of like an if statement, which says, if you get an exception called ValueError...
except ValueError:
# Do this instead of dying
print("What kind of integer is that?")
So the try statement links the potental problem code to the solution you've designed for dealing with the expected problem. It's true that this and the if statement are both conditional, i.e., both share the idea that it may or may not run certain code, depending on whether something is true. (Is cupcake == 42? Did this line of code throw a ValueError?)
Hope this helps!

ms28
Python Web Development Techdegree Student 1,067 PointsHey Louise!
Thanks for that detailed answer. From what I understood, this is how try things work:
try: #the code that is probably going to cause issues except: # what to do when you get the expected issue else: # what to do after the except
Does that make sense?
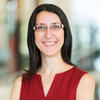
Louise St. Germain
19,424 PointsYou're very close! I would say this:
try:
#the code that is probably going to cause issues
except:
# what to do when you get the expected issue
else:
# what to do if you don't get the expected issue
# What to do after the except (or non-except) would go here,
# i.e., not indented, so not within the try/except/else statement.

ms28
Python Web Development Techdegree Student 1,067 PointsAwesome!
I think I have a grasp of the main component here.
Another question.
except as err:
Whats the "as err" part about, and why do people use it if it's not necessary all the time?