Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial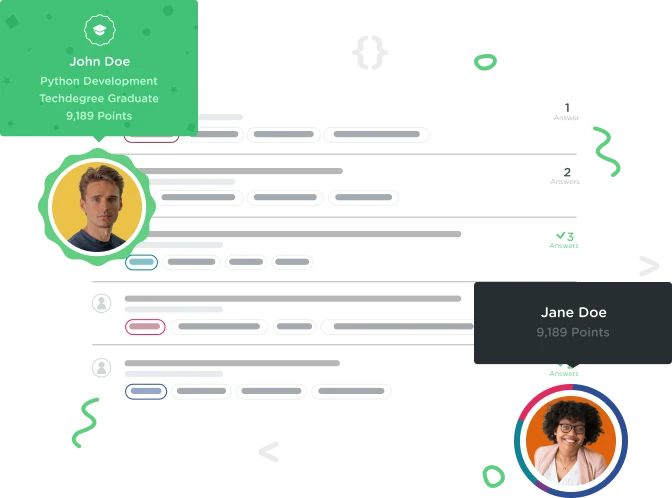
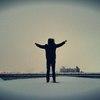
Shmuel Zilberman
1,409 Pointsim not really getting the input function can someone explain
im not really getting the input function can someone explain (python)
2 Answers
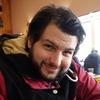
Eric M
11,546 Pointsinput()
works like most other function in your code, it takes arguments and returns a value.
For instance, say we have a function to double a number:
def times_two(original_number):
doubled_number = original_number * 2
return doubled_number
This function takes one argument, original_number
, multiplies it by two, and returns that new value. So if we want to use the new value somewhere else in our program, we have to store it somewhere, like in another variable.
Take a look at this code snippet using our times_two function:
def times_two(original_number):
doubled_number = original_number * 2
return doubled_number
def other_function():
foo = 5
doubled_foo = times_two(foo)
Here other_function
declares a variable foo
, assigns it the integer value of 5, and then stores the result from our times_two
function (when passed the argument foo) into doubled_foo
, which is another variable.
There's a lot going on with the line doubled_foo = times_two(foo)
let's focus on the right hand side of the assignment operator (the right hand side of the equals sign).
First we've passed the value foo
as an argument to the function times_two
. times_two
gets the value of foo and assigns it to the original_number
parameter. times_two
only takes one parameter, original_number
.
Then times_two
creates a new variable, doubled_number
, multiples original_number
by 2 (original_number
has foo's value of 5) then this gets returned.
When we say a value gets returned, we can think of it like the function call times_two(foo)
is replaced by the value.
So here doubled_foo = times_two(foo)
is equivalent to doubled_foo = 10
after the times_two
function has completed executing with foo passed to it.
input()
works in the same way, you pass it a string as an argument, this is the text that will be displayed to the user in the command line, then whatever the user types in the command line is returned to where you called you input function. input()
is doing a lot more behind the scenes than our times_two
function, but you don't need to worry about that for now.
Just know that when you type:
some_text = input("please type something: ")
The input function will display your string argument to the user (in this case "please type something: ") and will then return whatever the user types. Here we've said to store the return value in the some_text
, so whatever the user types will be stored there for us to use.
Let me know if you have any specific questions about this function! Handling user input allows you to do much more creative things with your programs.

Lucas Garcia
6,529 PointsThe input() function prompts the user to type when you run the script and whatever the user types is stored as a string. If you set the input function to a variable like this_variable = input() then whatever the user types will be stored in this_variable as a string. Hope this helps.