Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial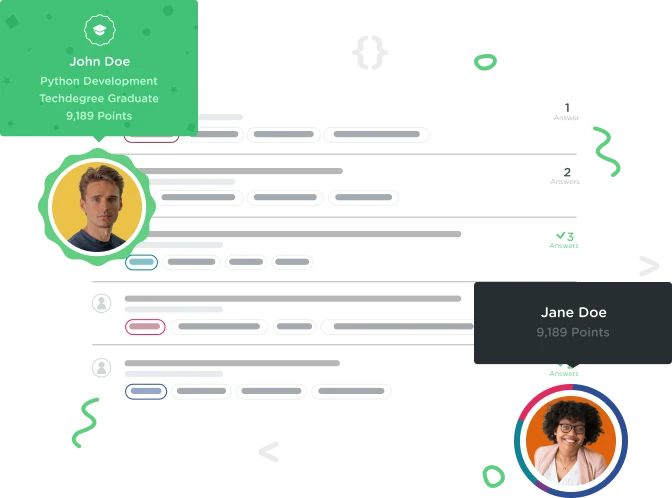

Naomi Koo
2,963 PointsI'm not sure how to go through each element in mContactMethods when I do my for loop
I didn't understand sets and maps all that well, and I'm not sure how to go through each element of a map
package com.example.model;
import java.util.Map;
import java.util.Set;
import java.util.HashSet;
import java.util.HashMap;
public class Contact {
private String mFirstName;
private String mLastName;
private Map<String, String> mContactMethods;
public Contact(String firstName, String lastName) {
mFirstName = firstName;
mLastName = lastName;
/* This stores contact methods by name
* eg: "phone" => "(555) 555-1234"
*/
mContactMethods = new HashMap<String, String>();
}
public void addContactMethod(String method, String value) {
mContactMethods.put(method,value);
}
/**
* Returns the available contact methods. eg: phone, pager,
*
* @return The name of the contact methods that are available
*/
public Set<String> getAvailableContactMethods() {
// FIXME: This should return the current contact method names.
Set<String> setKeys = new HashSet<String>();
for (Map<String, String> mContactMethods: mContactMethods){
setKeys.add(key);
}
return setKeys;
}
/**
* Returns the value for the contact method if it exists,
*
* @param methodName The name of the contact method to look up.
* @return The name of the contact methods that are available
*/
public String getContactInfo(String methodName) {
// FIXME: return the value for the passed in *methodName*
return null;
}
public String getFirstName() {
return mFirstName;
}
public String getLastName() {
return mLastName;
}
}
2 Answers

Ken Alger
Treehouse TeacherNaomi;
Welcome to Treehouse!
Let's have a look.
Task 2
In this task, let's fix the
getAvailableContactMethods
method. We want to return a set of the currently defined contact methods.HINT: How about a set of the keys from mContactMethods?
Here is our prompting code for the getAvailableContactMethods
method.
public Set<String> getAvailableContactMethods() {
// FIXME: This should return the current contact method names.
return null;
}
In looking at the code, we need our return type to be a Set<String>
. If you recall from the videos this is an implementation of the Set
interface, and in this instance it is a Set
of String
s. Now, if we look at the hint Mr. Dennis provided, we should be able to access the String
representation of the keys in mContactMethods
, which if we look up at the top of the challenge code is defined as a HashMap<String, String>
.
How can we get that information though? There is a method called keySet()
that allows us to do just that! In looking in the Java Docs for the Map
Interface, we find that under the Method Summary section keySet()
"Returns a Set view of the keys contained in this map." Sounds like just what we need.
Therefore, we should be able to do something like:
Set<String> methods = (Set) mContactMethods.keySet();
Then we just need to return the data from the getAvailableContactMethods()
method.
Post back if you are still stuck.
Happy coding,
Ken
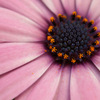
Yael P.
2,816 PointsYou can also use it that way:
public Set<String> getAvailableContactMethods() {
return mContactMethods.keySet();
}