Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial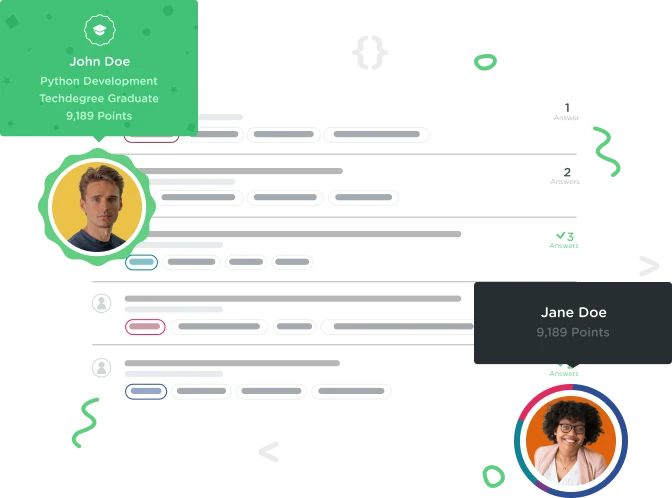

Pilar Fernandez
3,085 PointsI'm not sure how to select the p and button elements from the same list element using parentNode
I need to select the button so when the user clicks on it, it adds the class to the sibling paragraph element, but I don't know how to select them using parentNode.
const list = document.getElementsByTagName('ul')[0];
const p = document.querySelector('ul li p');
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
let btn = p.nextElementSibling;
if(btn){
p.classList.add('highlight');
}
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p>Element Selection</p><button>Highlight</button></li>
<li><p>Events</p><button>Highlight</button></li>
<li><p>Event Listening</p><button>Highlight</button></li>
<li><p>DOM Traversal</p><button>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
1 Answer

tobiastrinkler
Full Stack JavaScript Techdegree Student 16,538 PointsHi Pilar,
Since you have to use DOM Traversal you should not create an extra variable to grab onto the paragraph element on the page. When you look at the HTML code you can see that the button and paraghraph element are siblings. You can also see that paragraph element comes before the button which means in our handler we can use the previousElementSibling method to grab onto the paragraph element when a button is clicked.
Hope I could help you out with this :).
const list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
e.target.previousElementSibling.className = 'highlight';
}
});
Pilar Fernandez
3,085 PointsPilar Fernandez
3,085 PointsThank you Tobias Trinkler !!! It was way simpler than what I was doing lol