Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial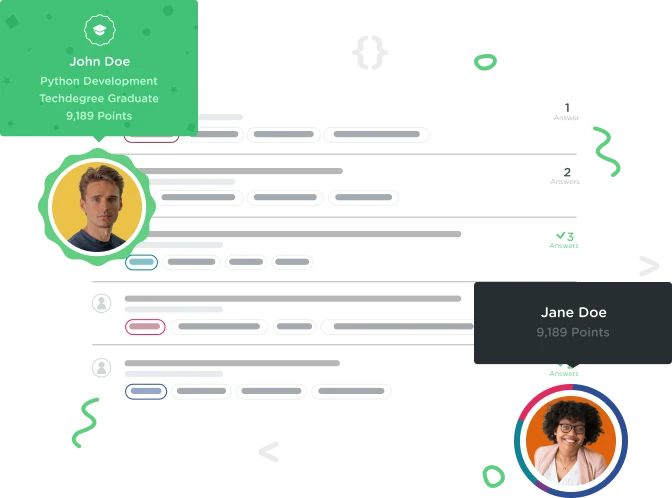
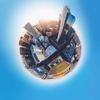
Joel Crouse
4,727 PointsI'm not sure how to solve this.
I'm not sure how to solve this code challenge. I'm supposed to add all the numbers in numbers array and assign the sum to sum variable.
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter <= numbers.count {
counter += 1
sum = sum + numbers[counter]
}
1 Answer
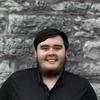
Michael Hulet
47,912 PointsYour syntax here is correct and your code will compile just fine, but you have a couple of logical errors here. I think they'll become most obvious if we step through your code.
Your first problem is an ever-common "off by 1" bug. At the first iteration of the loop, counter
will be 0
, which is less than or equal to numbers.count
, so the code in the loop will run. The first thing that happens is that 1
is added to counter
, so counter
is now equal to 1
. Next, you use the counter
variable to index into the numbers
array and add the value at the index of the current value of counter
to sum
. Remember that array indexes start at 0
, but the first time this line runs, counter
is 1
, so it actually gets the second item in the numbers
array. This will continue for the entire loop, and ultimately the first value will be skipped entirely. What you really wanna do here is increment your counter after you've done all the work in your loop.
Once you've fixed that issue, you still have one more problem, but it won't present itself until the very last iteration of the loop. On the last iteration, counter
is equal to numbers.count
, which is 7
in this case. This passes the check in your condition, so the code in the loop runs. When it gets to the point where you index into the numbers
array, it'll try to get the value at index 7
, and your program will crash. This is also because array indexing starts at 0
. Because of that, the highest index in an array will always be equal to array.count - 1
. Thus, the index 7
doesn't exist in this array. Thus, the code challenge asks you to check if count
is less than numbers.count
, and not less than or equal to.
Once you fix those 2 issues, your code should pass just fine!
Joel Crouse
4,727 PointsJoel Crouse
4,727 PointsThanks! I passed the code challenge.