Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial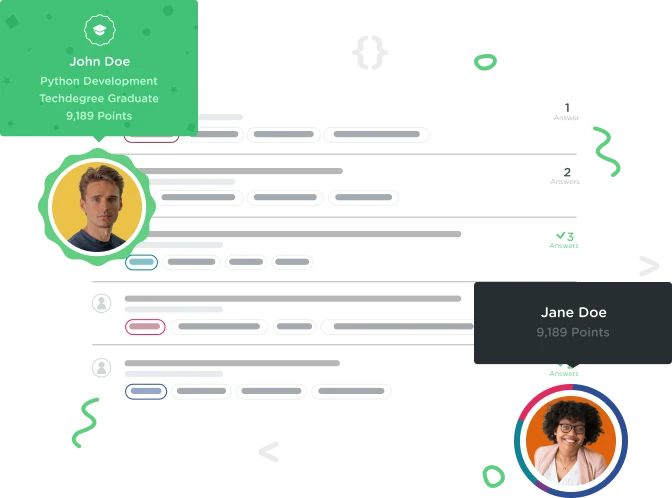
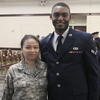
Darrin Spell Jr
Full Stack JavaScript Techdegree Student 10,303 PointsI'm not sure I quite understand this challenge.
I don't think my approach to this challenge is correct. Can someone help explain this to me please.
def stringcases ("single",*args):
for string in stringcases
print string.uppercase
print string.lowercase
print string.titlecased
print string.reversed
return string
1 Answer

Andrew Bruce
7,822 PointsHi Darrin,
The challenge is asking you to take in one string, and output a tuple containing the same string but in different cases (e.g. upper case, lower case etc.).
A tuple is a Python collection which has an unchangeable order and is represented by round brackets () separated by commas. You need to use string methods to alter the string for the first 3 like so -
All uppercase = string.upper()
All lowercase - string.lower()
Titlecased = string.title()
To reverse the string you need to use a slice like so - Reversed = string[::-1]
More on slices here - https://www.pythoncentral.io/how-to-slice-listsarrays-and-tuples-in-python/
Once you have the strings you need to put them into a tuple and return them, you were printing them in your example, this is not the same thing. Returning the value just returns it to the console, if you were to print it you would call the following -
print(stringcases())
You can shorten this into 2 lines though with the following -
def stringcases(string):
return (string.upper(), string.lower(), string.title(), string[::-1])
Istvan Nonn
2,092 PointsIstvan Nonn
2,092 PointsThanks Andrew, just so everybody has a clear idea about the code checker. So what I had is:
you can see I did text.lower() method first and than text.upper(). But they ask for the upper string first than for lower so I switched the order