Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial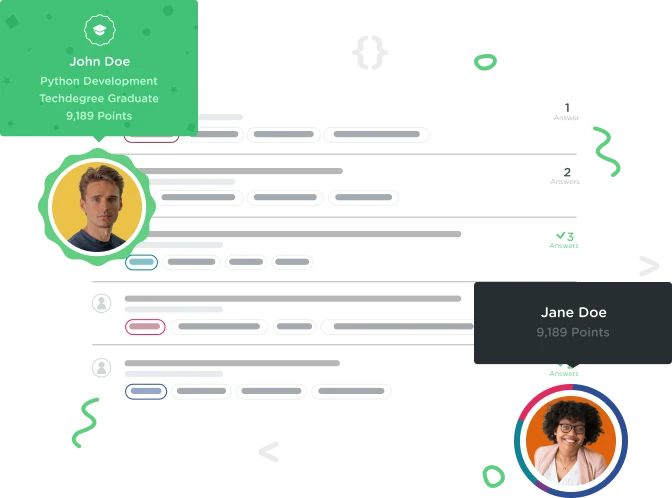

Dan Holroyd
1,172 PointsI'm not sure if I'm getting "Bummer" because of a syntax error or because of something I'm leaving out.
If someone might give me a clue, I'd be appreciative. Thank you.
def loopy(items):
# Code goes here
try:
for item in items:
print(item)
except:
if item.lower == "a":
else:
print(item)
3 Answers
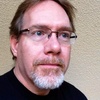
Chris Freeman
Treehouse Moderator 68,454 PointsYou have the right elements, but a few fixes are needed:
- The 'try/except
code is not needed A
try` block is used to capture errors when working with possible unknown results. - when calling method
lower()
include parens to call the function. Otherwise you're just pointing to the function. - To reference the first character use the slice notation:
item.lower()[0]
- be sure to print if
not
an "a"
Structure should be:
# for statement
# if statement
# print statement
Post back if you need more help. Good luck!!!
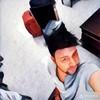
Ari Misha
19,323 PointsHiya Dan! Lets look at the example given in the challenge: ["abc", "xyz"] will just print "xyz". So for each item in the list thats being passed to the function, if the character at index 0 is "a" , the we want to skip that step and continue, otherwise we need to print the current member. So your code should look like this:
def loopy(items):
# Code goes here
for item in items:
if item[0] == "a":
continue
else:
print(item)

Frank Torchia
18,346 PointsHi Dan,
Try blocks should really be reserved for error handling. For example, if you wanted to loop through the items and make sure there were no special characters (#^&$%) or null items.
That being said, this is what I changed for your answer
def loopy(items):
for item in items:
if item[0].lower() != "a":
print(item)
Notice how I'm comparing item[0]
instead of item
? Using item
means that our code is using the entire string. For the exercise, we need to find the first letter, or in other words, the letter in the first index in the string, hence item[0]
.
I also used the !=
equality operator which translates to does not equal. In English if item[0].lower() != "a":
means if the first letter in the item does not equal 'a'
Hope this helps!
Andrew Fitzpatrick
3,013 PointsAndrew Fitzpatrick
3,013 Pointsim having the same problem i cant figure out how to make the program look just at the index 0 to check for 'a'