Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial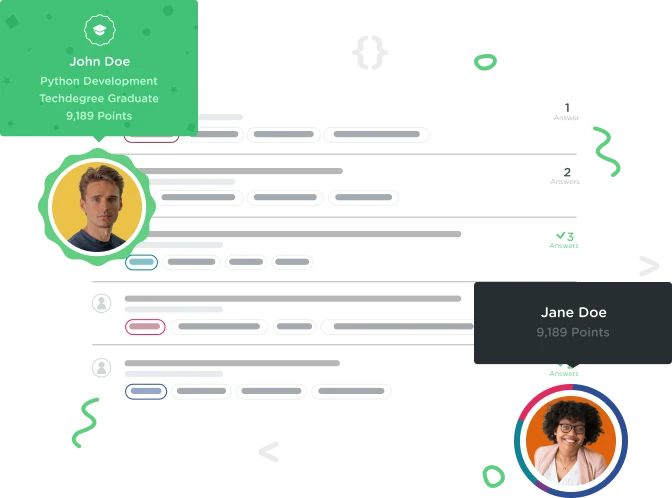
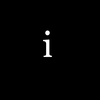
Furquan Ahmad
5,148 PointsI'm not sure on how to do the looping
How do i know if they have the tile and increment it ? I'm really lost.
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount (char tile) {
for(char tile) {
int Counter + 1;
}
return Counter;
}
}
3 Answers
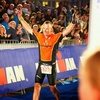
Steve Hunter
57,712 PointsThe method needs to take a char as a parameter. The for:each
loop then iterates through the mHand
string and counts how many instances of that char
occur. So, if the player's hand was H E L L O
, the method would be called like: getTileCount('L');
and it would return 2
, the number of Ls in the hand.
My solution looks like:
public int getTileCount(char tile){
int count = 0;
for (char letter : mHand.toCharArray()) {
if(letter == tile){
count++;
}
}
return count;
}
I hope that makes sense!
Steve.
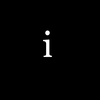
Furquan Ahmad
5,148 PointsOk thanks so much Steve, this is what makes the challengers harder in my opinion the lack of clarity of how the other code got there :I
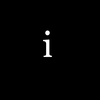
Furquan Ahmad
5,148 PointsYeah thanks so much Steve, about the parameter where does it receive it's data from ?
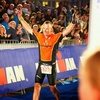
Steve Hunter
57,712 PointsYou pass the parameter into the method so I guess that would depend how you implement the game.
It is only an example so the detail isn't really there.
Furquan Ahmad
5,148 PointsFurquan Ahmad
5,148 PointsThis kind of makes sense, how comes you can call the member variable without declaring it.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsYou mean
mHand
? The method is held inside the class so every instance of the class can use the method and methods in each instance can access the member variables.Does that make sense?
Steve.
Furquan Ahmad
5,148 PointsFurquan Ahmad
5,148 PointsYes this makes sense, it's getting really difficult especially when on the HangMan class, declaring all the other stuff isn't really explained too well.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsIt should start getting easier as you get used to it all. There's a lot to take in!
Keep on going and just shout in the Community pages if you get stuck - there's always someone around to help.
Furquan Ahmad
5,148 PointsFurquan Ahmad
5,148 PointsSorry trying to code this myself i like to expalain things through to help me understand the concept. You get the tile through the paramter char. You create a variable for counting purposes. This is the bit i'm not 100% sure on. You do a for each loop which letter stores the contents of mHand in a spaced out format. If the letter is the same as tile +1 each time.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi there,
This method is used to see how many times a certain letter is in the Scrabble hand. It is used by passing the letter you want to now about to the method;
getTileCount('L');
, for example.Inside the method, the letter is received as the
char
that I calledtile
. We then set up a variable just to do the counting; I called itcount
because I lack imagination. ;-) We start withcount
being zero.Next, we create a
for:in
loop. The loop iterates overmHand
after that string has been turned into an array with the methodtoCharArray
. The loop defines a local variable just for the loop - it is achar
and I called itletter
. This variable changes each time the loop runs. It holds the value of each letter inmHand
in turn.Let's stick with the example I used before (even though there aren't enough letters in it!!) and use "HELLO" as
mHand
. On the first pass of the loop,letter
holds 'H'. This is then compared withtile
that was passed in. Ifletter
andtile
equal the same thing, then we add one to count;count++
. Else we carry on and do nothing.Either way, we get to the next iteration;
letter
now hold 'E' and the loop goes on as before. Ifletter
is the same astile
,count++
else do nothing. And repeat until we run out of letters inmHand
.OK with that?
Steve.