Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial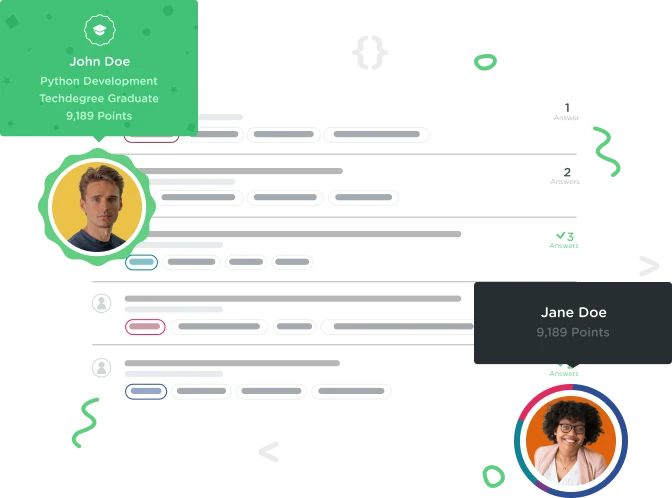

Patrick Largen
3,650 PointsI'm not sure what I am doing wrong here... It keeps telling me to store the path in a constant named plistPath.
What am I doing wrong? Since this path leads to nil, do I need to optional binding? Any help would be appreciated
import Foundation
let plistPath = NSBundle.mainBundle().pathForResource("CrazyInformation", ofType: "plist")
let instance = NSDictionary(contentsOfFile: "CrazyInformation")
2 Answers

Daniel Rosensweig
5,074 PointsYou have two problems here, Patrick.
One is safety with the optionals, as you mentioned. Wrapping it in an 'if let' statement will solve this. Strictly speaking, this isn't necessary for your code to run, but it seems to be necessary for the challenge -- it works for the challenge when I wrap it this way, and doesn't when I just assign the two constants. But, since you already knew of this problem, you probably already tried it both ways.
The other issue is that you're not USING the plistPath you created in your NSDictionary. You need to provide NSDictionary with the path to the file you want, not just a name. In your example, NSDictionary doesn't know where "CrazyInformation" is. Give it plistPath for input instead.
import Foundation
if let plistPath = NSBundle.mainBundle().pathForResource("CrazyInformation", ofType: "plist") {
let plist = NSDictionary(contentsOfFile: plistPath)
}

Patrick Largen
3,650 PointsThanks, Daniel!