Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial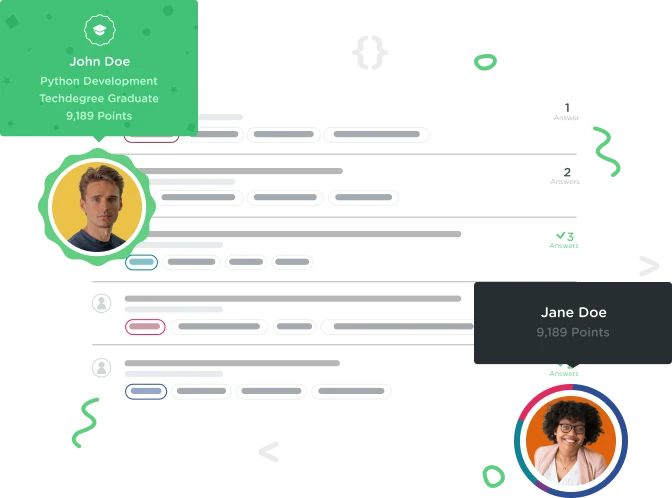

Asher Goldsby
Full Stack JavaScript Techdegree Student 4,055 PointsI'm not sure what I did wrong
Awesome! Now I'd like to see only groups that are trios, you know 3 members. So can you please only print out the trios? It should still use the joined string format from task 1. Bummer: AssertionError: 'Ad Rock, MCA, Mike D.' not found in '' : Hmmm...I'm not getting the output I expected. RestartPreviewGet HelpRecheck work groups.py
1 musical_groups = [ 2 ["Ad Rock", "MCA", "Mike D."], 3 ["John Lennon", "Paul McCartney", "Ringo Starr", "George Harrison"], 4 ["Salt", "Peppa", "Spinderella"], 5 ["Rivers Cuomo", "Patrick Wilson", "Brian Bell", "Scott Shriner"], 6 ["Chuck D.", "Flavor Flav", "Professor Griff", "Khari Winn", "DJ Lord"], 7 ["Axl Rose", "Slash", "Duff McKagan", "Steven Adler"], 8 ["Run", "DMC", "Jam Master Jay"], 9 ] 10 11 if len(musical_groups) == 3: 12 for group in musical_groups: 13 print(", ".join(musical_groups[0])) 14 print(", ".join(musical_groups[1])) 15 print(", ".join(musical_groups[2])) 16 print(", ".join(musical_groups[3])) 17 print(", ".join(musical_groups[4])) 18 print(", ".join(musical_groups[5])) 19 print(", ".join(musical_groups[6])) 20
musical_groups = [
["Ad Rock", "MCA", "Mike D."],
["John Lennon", "Paul McCartney", "Ringo Starr", "George Harrison"],
["Salt", "Peppa", "Spinderella"],
["Rivers Cuomo", "Patrick Wilson", "Brian Bell", "Scott Shriner"],
["Chuck D.", "Flavor Flav", "Professor Griff", "Khari Winn", "DJ Lord"],
["Axl Rose", "Slash", "Duff McKagan", "Steven Adler"],
["Run", "DMC", "Jam Master Jay"],
]
if len(musical_groups) == 3:
for group in musical_groups:
print(", ".join(musical_groups[0]))
print(", ".join(musical_groups[1]))
print(", ".join(musical_groups[2]))
print(", ".join(musical_groups[3]))
print(", ".join(musical_groups[4]))
print(", ".join(musical_groups[5]))
print(", ".join(musical_groups[6]))
11 Answers

Ashcon Khoubyari
12,907 PointsHere is the answer:
for group in musical_groups:
if len(group) == 3:
print(", ".join(group))
It's one of those bugs that you need to close out of the window and go back into it.
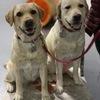
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Asher,
Your first line of code reads as follows:
if len(musical_groups) == 3:
This is checking whether there are 3 elements in the list musical_groups
and if there are, executes all the code inside your if
block. There are not 3 elements in the list musical_groups
, there are 7. So this line evaluates to False and none of the rest of your code is ever executed.
What you want to do is test each element of musical_groups
to see whether that element has a length of 3.
You already have a for loop, so each iteration of the loop you are looking at one element in musical_groups
, which has the temporary variable name group
. Thus, each time you go through the loop you want to check if group
has a length of 3.
If so, print the members of just that group as a comma separated string.
Hope that clears everything up for you.
Cheers
Alex

Adam Tanner
7,101 PointsThis helped a lot, thanks!

Feisty Mullah
25,849 PointsHi, I just came here to find the answer of same question as I could not find the answer. I tried in REPL myself a number of times and eventually passed my challenge. here is my line of code. You may have found already the answer as you have asked for help about 2 months ago but I thought it's still a good idea to share it for someone else may need in future.
musical_groups = [
["Ad Rock", "MCA", "Mike D."],
["John Lennon", "Paul McCartney", "Ringo Starr", "George Harrison"],
["Salt", "Peppa", "Spinderella"],
["Rivers Cuomo", "Patrick Wilson", "Brian Bell", "Scott Shriner"],
["Chuck D.", "Flavor Flav", "Professor Griff", "Khari Winn", "DJ Lord"],
["Axl Rose", "Slash", "Duff McKagan", "Steven Adler"],
["Run", "DMC", "Jam Master Jay"],
]
for musical_group in musical_groups:
if len(musical_group) == 3:
print(", ".join(musical_group))
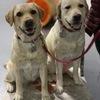
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Asher,
Let's a try a simple example and see if we can clarify what we are trying to achieve.
To begin, let's create a 2D list of characters:
character_lists = [
["Jake", "Finn", "BMO", "Princess Bubblegum"],
["Ralph", "Vanellope", "Felix"],
["Emmet", "Lucy", "Unikitty", "Batman],
]
Now, let's suppose we want to iterate through each of the character_lists, check each one to see if it has 4 characters, and if it does, print that character_list:
for character_list in character_lists:
if len(character_list) == 4:
print(character_list)
Now, in the real example you need to do a little more inside the print statement, but nothing that you haven't already done in the previous step.
Hope that's clear,
Cheers
Alex
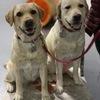
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Asher,
You still have all those print statements. Each time you iterate through your for loop you only want to print either one or none groups: if the group has three members, print just that group. If it doesn't have three members, don't print anything.
The way you have written your code, every time your for loop hits a group with three members, it will print ALL the groups.
Looking at the example groups provided, your for loop does this:
-
First iteration:
group
is["Ad Rock", "MCA", "Mike D."]
. Yourif
statement checks ifgroup
has a length of three, which it does. So the if branch is executed and you print every group:"Ad Rock, MCA, Mike D." "John Lennon, Paul McCartney, Ringo Starr, George Harrison" "Salt, Peppa, Spinderella" "Rivers Cuomo, Patrick Wilson, Brian Bell, Scott Shriner" "Chuck D., Flavor Flav, Professor Griff, Khari Winn, DJ Lord" "Axl Rose, Slash, Duff McKagan, Steven Adler" "Run, DMC, Jam Master Jay"
Second iteration:
group
is["John Lennon", "Paul McCartney", "Ringo Starr", "George Harrison"]
. Yourif
statement checks ifgroup
has a length of three. It doesn't, so the if branch is not executed and we go straight to the next iteration of the loop.-
Third iteration:
group
is["Salt", "Peppa", "Spinderella"]
. Yourif
statement checks ifgroup
has a length of three, which it does. So the if branch of your code is executed and you once again print every group:"Ad Rock, MCA, Mike D." "John Lennon, Paul McCartney, Ringo Starr, George Harrison" "Salt, Peppa, Spinderella" "Rivers Cuomo, Patrick Wilson, Brian Bell, Scott Shriner" "Chuck D., Flavor Flav, Professor Griff, Khari Winn, DJ Lord" "Axl Rose, Slash, Duff McKagan, Steven Adler" "Run, DMC, Jam Master Jay"
Fourth iteration:
group
is["Rivers Cuomo", "Patrick Wilson", "Brian Bell", "Scott Shriner"]
. Yourif
statement checks ifgroup
has a length of three. It doesn't, so the if branch is not executed and we go straight to the next iteration of the loop.Fifth iteration:
group
is["Chuck D.", "Flavor Flav", "Professor Griff", "Khari Winn", "DJ Lord"]
. Yourif
statement checks ifgroup
has a length of three. It doesn't, so the if branch is not executed and we go straight to the next iteration of the loop.Sixth iteration:
group
is["Axl Rose", "Slash", "Duff McKagan", "Steven Adler"]
. Yourif
statement checks ifgroup
has a length of three. It doesn't, so the if branch is not executed and we go straight to the next iteration of the loop.-
Seventh iteration:
group
is["Run", "DMC", "Jam Master Jay"]
. Yourif
statement checks ifgroup
has a length of three, which it does. So the if branch of your code is executed and you once again print every group:"Ad Rock, MCA, Mike D." "John Lennon, Paul McCartney, Ringo Starr, George Harrison" "Salt, Peppa, Spinderella" "Rivers Cuomo, Patrick Wilson, Brian Bell, Scott Shriner" "Chuck D., Flavor Flav, Professor Griff, Khari Winn, DJ Lord" "Axl Rose, Slash, Duff McKagan, Steven Adler" "Run, DMC, Jam Master Jay"
Hopefully it is obvious why this is not the correct output. Let's compare this to the behaviour of the example code I provided earlier:
character_lists = [
["Jake", "Finn", "BMO", "Princess Bubblegum"],
["Ralph", "Vanellope", "Felix"],
["Emmet", "Lucy", "Unikitty", "Batman],
]
for character_list in character_lists:
if len(character_list) == 4:
print(character_list)
-
First iteration:
character_list
is["Jake", "Finn", "BMO", "Princess Bubblegum"]
. Myif
statement checks ifgroup
has length of four, which it does. So the if branch of my code is executed and I print:["Jake", "Finn", "BMO", "Princess Bubblegum"]
Second iteration:
character_list
is["Ralph", "Vanellope", "Felix"]
. Myif
statement checks ifgroup
has length of four. It doesn't, so the if branch is not executed and we go straight to the next iteration of the loop.-
Third iteration:
character_list
is["Emmet", "Lucy", "Unikitty", "Batman]
. Myif
statement checks ifgroup
has length of four, which it does. So the if branch of my code is executed and I print:["Emmet", "Lucy", "Unikitty", "Batman]
As you can see, I only ever print the values that match my conditional.
Let me know if something is still unclear.
Cheers
Alex

Asher Goldsby
Full Stack JavaScript Techdegree Student 4,055 PointsWhat you are saying makes sense but I can’t get the right the code. I have to check if each group has 3 elements in it
I tried various forms of if len(group) == 3:
Print(so and so forth)
But it didn’t work. I put it in the top above the print section as well above each print individually and it didn’t work
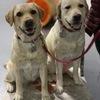
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Asher,
Can you paste your actual code into the answer, please. Maybe there is a subtle typo.
Cheers
Alex

Asher Goldsby
Full Stack JavaScript Techdegree Student 4,055 PointsThis just isn’t making sense
I’ve spent hours trying to do this and I don’t get it
Honestly I’m not spending $200 a month for tech degree where I can waste days if not weeks stuck on a single challenge

Asher Goldsby
Full Stack JavaScript Techdegree Student 4,055 PointsIt didn’t work
I put substituted the first two lines of code and still didn’t work
In fact I tried that exact before

Asher Goldsby
Full Stack JavaScript Techdegree Student 4,055 Pointsfor group in musical_groups: if len(group) == 3: print(", ".join(musical_groups[0])) print(", ".join(musical_groups[1])) print(", ".join(musical_groups[2])) print(", ".join(musical_groups[3])) print(", ".join(musical_groups[4])) print(", ".join(musical_groups[5])) print(", ".join(musical_groups[6]))

Shelley Brown
7,613 PointsThis should work.
for group in musical_groups: if len(group) == 3: print(", ".join(group))