Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial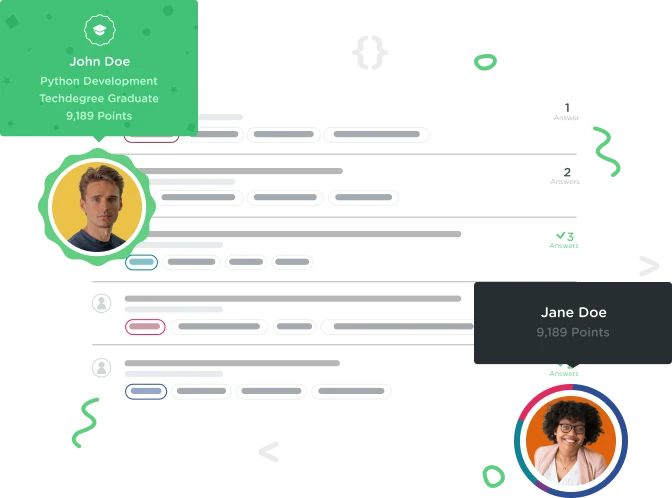
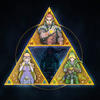
Don Newcomb
4,755 PointsI'm not sure what I've done wrong. please help!
I've been working on this for a few days. Having a really hard time with this final step in particular. Please tell me what I've done wrong.
Thank you.
public class Forum {
private String topic;
public Forum(String topic){
topic = topic;
}
public String getTopic() {
return topic;
}
public void addPost(ForumPost post) {
// When all is ready uncomment this...
System.out.printf("New post from %s %s about %s \n and %s",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle(),
post.getDescription());
}
}
public class User {
private String firstName;
private String lastName;
public User(String firstName, String lastName) {
// TODO: Set the private fields here
firstName =firstName;
lastName = lastName;
}
public String getFirstName(){
return firstName;
}
public String getLastName(){
return lastName;
}
}
public class ForumPost {
private User author;
private String title;
private String description;
public ForumPost(User author, String title, String description){
author = author;
title = title;
title = " ";
description = description;
description = " ";
}
public User getAuthor() {
return author;
}
public String getTitle() {
return title;
}
// TODO: We need to expose the description
public String getDescription(){
return description;
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
// Take the first two elements passed args
User author = new User(args[0], args[1]);
// Add the author, title and description
ForumPost post = new ForumPost(author,"Finishing OOP","It feels Great!" );
forum.addPost(post);
}
}
2 Answers
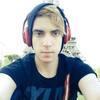
Melodie 1
3,931 PointsYour constructors are the problem , when you initialize a variable inside a constructor you must either use the keyword this or prefix the instance variables with m .
example 1 : public Forum(String topic){ this.topic = topic; }
example 2 : private String mTopic ; public Forum(String topic){ mTopic = topic; }

Sara Pasinato
1,023 Pointsin Public User you are named the private variables equals the variable in the public User and the compiler don't understand the difference :)
public User(String firstName, String lastName) {
// TODO: Set the private fields here
this.firstName =firstName;
this.lastName = lastName;
I hope can help you :)
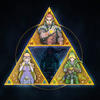
Don Newcomb
4,755 PointsThank you very much! pointing this out helped me a great deal :)
Don Newcomb
4,755 PointsDon Newcomb
4,755 PointsThank you so much. I solved the problem. You made it easier to understand :)