Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial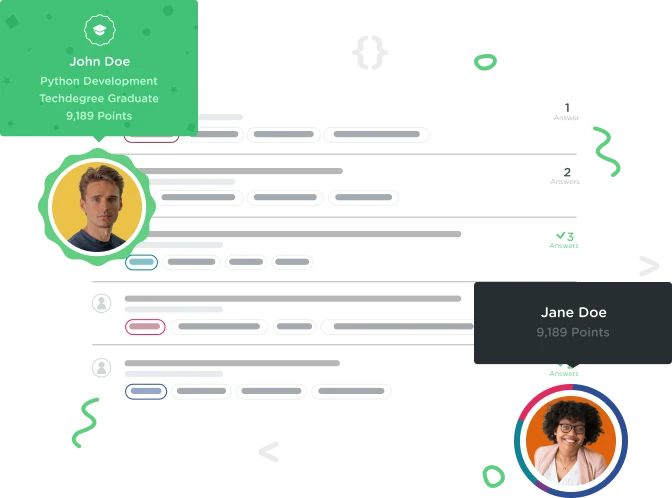

Joshua szkodlarski
8,604 PointsIm not sure what to do here
After the name field, add another input for email addresses. Set the type attribute to "email", the id attribute to "email", and the name to "user_email".
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>HTML Forms</title>
</head>
<body>
<form action="index.html" method="post">
<label for ="name">name:</label>
<input type="text" id="name" name="user_name">
<label for="comment">Comment:</label>
<textarea id="comment" name="user_comment"></textarea>
<button type="submit">Submit Comment</button>
</form>
</body>
</html>
3 Answers
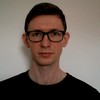
Joe Consterdine
13,965 Points<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>HTML Forms</title>
</head>
<body>
<form action="index.html" method="post">
<label for ="name">name:</label>
<input type="text" id="name" name="user_name">
<input type="email" id="email" name="user_email">
<label for="comment">Comment:</label>
<textarea id="comment" name="user_comment"></textarea>
<button type="submit">Submit Comment</button>
</form>
</body>
</html>
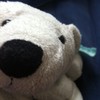
bothxp
16,510 PointsHi,
Task 3 of 4 : "After the name field, add another input for email addresses. Set the type attribute to "email", the id attribute to "email", and the name to "user_email"."
You already have the label & input elements for both 'name' & 'comment'. The question is asking you to now create a new input element between the 'name' & 'comment' areas, using the type, id & name attributes given in the tasks text.
So that is a new input tag using type="email", id="email" & name="user_email" added to the form after the 'name' input.
<form action="index.html" method="post">
<label for ="name">name:</label>
<input type="text" id="name" name="user_name">
<input type="email" id="email" name="user_email">
<label for="comment">Comment:</label>
<textarea id="comment" name="user_comment"></textarea>
<button type="submit">Submit Comment</button>
</form>
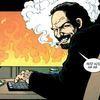
Greg Kitchin
31,522 PointsIt's not the best-worded question I found. I added the line after the 'name' label, figuring that was what was required. The above two are correct, it should be added after the 'user_name' line.
Taylor O'Reilly
9,417 PointsTaylor O'Reilly
9,417 PointsHey Josh!
So what this is asking you to do is to create a new input element within the form that will allow the user to place their
A fieldset is just a way to break related pieces of information into their own spaces. This is good if you have say an area where they enter personal information about themselves and then another where they enter a shipping address.
a fieldset looks something like this
For the email input this will be very similar to what you did to create the input for the name except, this time the attribute or "type" would be email.
This allows for easy input on most mobile devices. Technically the "text" attribute/type would work but that would just have the normal keyboard show up meaning the user wouldn't have front line access to special characters (mainly the @ symbol and the .com in the case of email).
There is a really easy to understand page on type selectors here http://www.w3schools.com/html/html_form_input_types.asp
using specific type selectors for inputs allows for a much better user experience and better practice overall!
Hope This helps!