Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial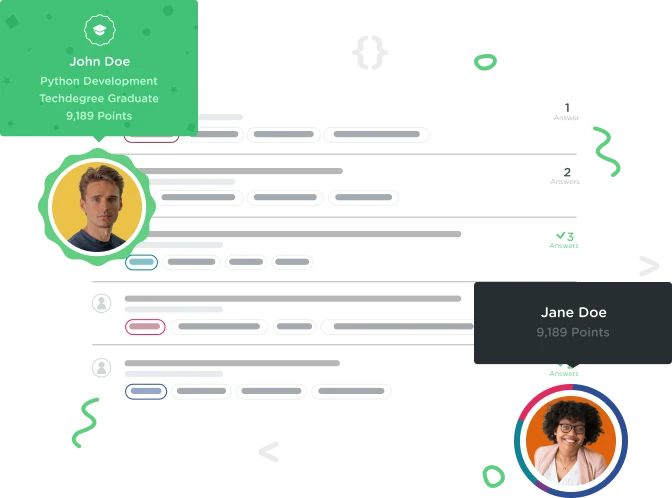

Alejandro Ochoa
Courses Plus Student 10,671 PointsIm not sure whats wrong with my code... any help..??
the task essentially says to "Make a new Model named Ingredient"... already did that but it just says " Try again"... Ive already looked at the given documentation and my fields seem to be ok.
Thanks
import datetime
from peewee import *
DATABASE = SqliteDatabase('recipes.db')
class Recipe(Model):
name = CharField()
created_at = DateTimeField(default=datetime.datetime.now)
class Meta:
database = DATABASE
class Ingredient(Model):
name = CharField()
description = TextField()
quantity = IntegerField()
measurement_type = Charfield()
recipe = ForeignKey()
class Meta:
database = DATABASE
# TODO: Ingredient model
# name - string (e.g. "carrots")
# description - string (e.g. "chopped")
# quantity - decimal (e.g. ".25")
# measurement_type - string (e.g. "cups")
# recipe - foreign key
from flask.ext.restful import Resource
import models
2 Answers
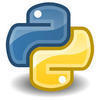
Gerald Wells
12,763 PointsThere are two field names that are incorrect:
-
IntegerField
---->DecimalField
-
ForeignKey
---->ForeignKeyField
Next, you aren't referencing anything with the ForiegnKeyField, without the value Recipe
in that field there is no relational construct.
Below is the corrected code, hope this helps!
class Ingredient(Model):
name = CharField()
description = CharField()
quantity = DecimalField()
measurement_type = CharField()
recipe = ForeignKeyField(Recipe)
class Meta:
database = DATABASE
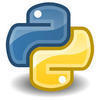
Gerald Wells
12,763 PointsSo I am assuming you know about class inheritance? If not heres a quick rundown,
class Parent:
value1 = 'This is Value 1'
value2 = 'This is Value 2'
class Parent2:
value3 = 'Parent class 2 varible of value 1'
value4 = 'Parent class 2 varible of value 2'
class Child(Parent, Parent2):
pass
###############################################################
>>> p = Parent()
>>> p2 = Parent2()
>>> c = Child()
>>> p.value1
'This is Value 1'
>>> p2.value4
'Parent class 2 varible of value 2'
>>> c.value1
'This is Value 1'
>>> c.value4
'Parent class 2 varible of value 2'
>>>
The relation formed in the above example is similar to the one ForiegnKeyField creates. The challenge states: I have the recipe model done but we need one to represent each of the ingredients in a recipe.
Logically you know that ingredients by themselves aren't really ingredients, they are just products. What makes them an ingredient is the recipe, the recipe gives the ingredients relation. Just like there isn't a relation between eggs and flour, until you are asked to bake a cake.
When you call ForeignKeyField you are telling your database that the current table is relational to another. If you leave that field blank an obvious error would be thrown because it is expecting you to tell it what relation needs to be made.
Alejandro Ochoa
Courses Plus Student 10,671 PointsAlejandro Ochoa
Courses Plus Student 10,671 PointsGerald Wells Thanks m8 worked like a charm,
I appreciate your help... now could you please elaborate on the part where without the ForeignKeyField referencing anything there is no rational construct?