Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial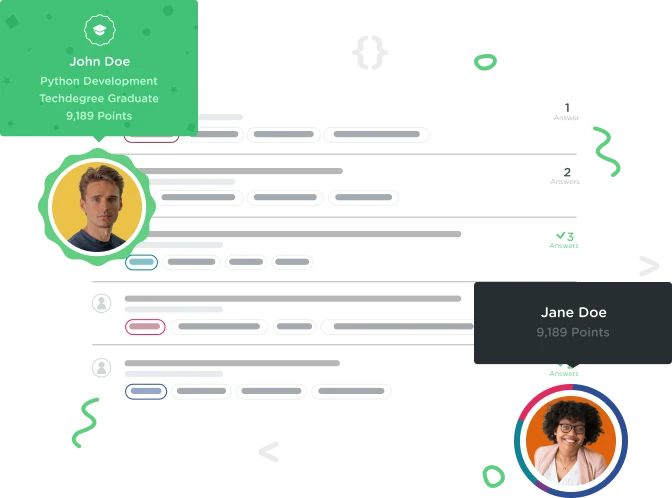

Austin Johnston
3,927 PointsI'm not sure why my answer is wrong, and I'm not sure what to change in my expected message line.
@Test public void restockingWithDifferentItemsIsNotAllowed() throws Exception { thrown.expect(IllegalArgumentException.class); thrown.expectMessage("Cannot be restocked with this item"); bin.restock("Cheetos", 1, 100, 50); bin.restock("Doritos", 1, 100, 50); } is my answer, but it says I'm displaying the wrong expected message, and not sure what that means.
package com.teamtreehouse.vending;
import org.junit.Before;
import org.junit.Rule;
import org.junit.Test;
import org.junit.rules.ExpectedException;
import static org.junit.Assert.*;
public class BinTest {
private Bin bin ;
@Rule
public ExpectedException thrown = ExpectedException.none();
@Before
public void setUp() throws Exception {
bin = new Bin(10);
}
@Test
public void restockingWithDifferentItemsIsNotAllowed() throws Exception {
thrown.expect(IllegalArgumentException.class);
thrown.expectMessage("Cannot be restocked with this item");
bin.restock("Cheetos", 1, 100, 50);
bin.restock("Doritos", 1, 100, 50);
}
@Test
public void whenEmptyPriceIsZero() throws Exception {
assertEquals(0, bin.getItemPrice());
}
@Test
public void whenEmptyNameIsNull() throws Exception {
assertNull(bin.getItemName());
}
@Test
public void overstockingNotAllowed() throws Exception {
thrown.expect(IllegalArgumentException.class);
thrown.expectMessage("There are only 10 spots left");
bin.restock("Fritos", 2600, 100, 50);
}
}
package com.teamtreehouse.vending;
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.BlockingQueue;
public class Bin {
private final BlockingQueue<Item> items;
public Bin(int maxItems) {
items = new ArrayBlockingQueue<>(maxItems);
}
public boolean isEmpty() {
return items.isEmpty();
}
public int getAvailableSlots() {
return items.remainingCapacity();
}
public String getItemName() {
if (isEmpty()) return null;
return items.peek().getName();
}
public int getItemPrice() {
if (isEmpty()) {
return 0;
}
return items.peek().getRetailPrice();
}
public Item release() {
return items.poll();
}
public void restock(String name, int amount, int wholesalePrice, int retailPrice) {
if (!isEmpty() && !name.equalsIgnoreCase(getItemName())) {
throw new IllegalArgumentException(String.format("Cannot restock %s with %s", getItemName(), name));
}
if (amount > getAvailableSlots()) {
throw new IllegalArgumentException(String.format("There are only %d spots left", getAvailableSlots()));
}
for (int i = 0; i < amount; i++) {
items.add(new Item(name, wholesalePrice, retailPrice));
}
}
}
package com.teamtreehouse.vending;
public class Item {
private final String name;
private final int wholesalePrice;
private final int retailPrice;
public Item(String name, int wholesalePrice, int retailPrice) {
this.name = name;
this.wholesalePrice = wholesalePrice;
this.retailPrice = retailPrice;
}
public String getName() {
return name;
}
public int getWholesalePrice() {
return wholesalePrice;
}
public int getRetailPrice() {
return retailPrice;
}
}
1 Answer

Benjamin Barslev Nielsen
18,958 PointsThe code from your solution:
@Test
public void restockingWithDifferentItemsIsNotAllowed() throws Exception {
thrown.expect(IllegalArgumentException.class);
thrown.expectMessage("Cannot be restocked with this item");
bin.restock("Cheetos", 1, 100, 50);
bin.restock("Doritos", 1, 100, 50);
}
"displaying the wrong expected message" means that the String given in the constructor to the exception thrown does not match "Cannot be restocked with this item", so now I will explain how to find the correct message:
The important part of the restock method:
public void restock(String name, int amount, int wholesalePrice, int retailPrice) {
if (!isEmpty() && !name.equalsIgnoreCase(getItemName())) {
throw new IllegalArgumentException(String.format("Cannot restock %s with %s", getItemName(), name));
}
...
}
When restock is called the second time, name is "Doritos", getItemName() is "Cheetos" and the exception is thrown. Remember that the argument to thrown.expectMessage(argument), needs to be the string, which is given to the constructor of the exception thrown. This means that the argument to thrown.expectMessage should be the String evaluated from: String.format("Cannot restock %s with %s", getItemName(), name). Since we have the expected values "Cheetos" and "Doritos", we can evaluate String.format("Cannot restock %s with %s", getItemName(), name) to "Cannot restock Cheetos with Doritos". Therefore the call should be:
thrown.expectMessage("Cannot restock Cheetos with Doritos");
Hope this helps