Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial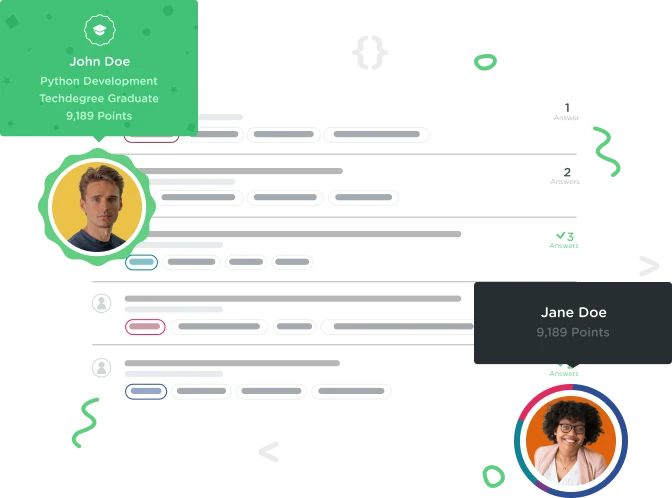

Kevin Morgan
1,475 PointsIm not sure why this code doesn't pass? It works in Playground...
I'm not sure why this doesn't pass the Challenge task 1 - it works in playground...
while counter < numbers.count {
sum = sum + numbers[counter]
counter = counter + 1
}
3 Answers
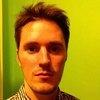
Brendan Whiting
Front End Web Development Techdegree Graduate 84,736 PointsAre you sure it works in the playground? I put in your code and I got the message: "Expected "{" after while condition."
At any rate, another problem is that your while condition needs to be a boolean expression. In this case, so long as the counter is less than the length of the array we should keep going and everything will line up nicely. We can use this boolean expression:
counter < numbers.count
to take care of this. So the whole thing would look like this:
while counter < numbers.count {
sum += numbers[counter]
counter++
}

Kevin Morgan
1,475 PointsIt was the first brace { !!!! Thank you :-)

Martin Wildfeuer
Courses Plus Student 11,071 PointsYou might not have posted the code you are using in Playground, as this won't compile. So let's take it step by step with the code you posted:
First of all, this code is missing the opening curly brace
while counter
...
}
should be
while counter {
...
}
Secondly, the while loop needs a condition
However, this won't compile either, as a while loop needs a condition that evaluates to true or false to run correctly.
With while counter {}
this is not the case, we want to make sure the while loop is executed x number of times, where x is the number of items in the numbers
array.
while counter < numbers.count {
...
}
<small>Please note that array indices are zero-based, that is the first value is at index 0. array.count gives you the total number of elements in an array, so when looping through an array, the last index will be one less than the total number of elements.</small>
In your code, you are incrementing counter
correctly, otherwise you might end up in an infinite loop.
Thirdly, use the counter value to get the right Int out of the numbers array
As you are incrementing counter with every loop, you can use it to step through the indexes of the numbers array. So the final code would look like this:
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
while counter < numbers.count {
sum += numbers[counter]
counter++
}
Hope that helped to clarify things a bit :)