Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial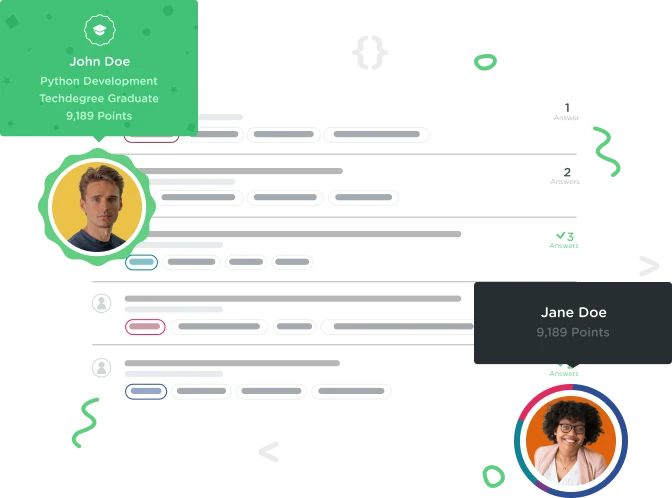
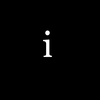
Furquan Ahmad
5,148 Pointsi'm not sure why this doesn't fit the requirements
i'm basically trying to say if the first letter is an M and the second letter is capitalized return true, otherwise throw an error.
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
if(fieldName.startsWith"m" || fieldName.startsWith "M" & Character.isUppercase(fieldName.charAt(1)))
{
return fieldName;
}
else {
throw new IllegalArguementException ("Must start with M and 2nd letter must be in capitals");
}
}
}
3 Answers
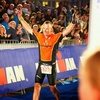
Steve Hunter
57,712 PointsHi there,
I think the fieldName
needs to start with a lower case 'm' then have a capitalized second letter.
That looks something like:
if(fieldName.charAt(0) == 'm' && Character.isUpperCase(fieldName.charAt(1))) {
// both things are true - do whatever success looks like
return fieldName;
} else {
throw new IllegalArgumentException("This does not meet the requirements!");
}
// or you can return fieldName here
return fieldName;
}
Steve.

Steven Stanton
59,998 PointsA few points:
- The AND operator is expressed with a double ampersand - && whereas your code has a single one.
- The && takes precedence over || - see http://introcs.cs.princeton.edu/java/11precedence/ - in other words, the phrase "fieldName.startsWith"M" & Character.isUppercase(fieldName.charAt(1))" will be evaluated first as true or false, and then the OR statement will be evaluated. You can prevent this by use of parenthesis:
((fieldName.startsWith("m")||fieldName.startsWith("M")) & Character.isUppercase(fieldName.charAt(1)))
(Also you need brackets for your arguments to fieldName.startsWith - (that's a lot of brackets, I know :) ))
- I'm pretty sure that the return key word needs to come near the bottom of the method, so you need to have the throw statement before that. (Not sure if this is syntactically required or just good practice, but it's one of the two)... So you can change the code as follows:
if(!((fieldName.startsWith("m")||fieldName.startsWith("M")) & Character.isUppercase(fieldName.charAt(1)))){ throw new ... }
return fieldName;
Hope that's helpful.
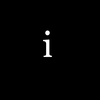
Furquan Ahmad
5,148 PointsHow comes you have a not at the start of the statement ?

Steven Stanton
59,998 PointsBecause you only want to throw an exception if that condition is not met - it does the same thing as your "else" in the code you posted, but allows you to reverse the order and have the return statement at the bottom, instead of inside the "if" block.
Pauliina K
1,973 PointsPauliina K
1,973 PointsShouldn't this code work too? If it does NOT meet these requirements throw an exception, otherwise return fieldName. Isn't that the same as if it DOES meet the requirements return fieldName, otherwise throw exception?
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsYep - reversing everything should work fine too.
Steve.
Pauliina K
1,973 PointsPauliina K
1,973 PointsHah, cool! I am finally understanding things! Woo!
Steve Hunter
57,712 PointsSteve Hunter
57,712 Points\o/ yay!
Pauliina K
1,973 PointsPauliina K
1,973 PointsBtw, how much should we know by now? I mean, I understand most things and can do the challenges and so on, but if we were to make any of these assignments (the Hangman game for example) on our own, I'd be lost. I would have no idea on where to start and so on. Is that normal? Or should I be able to do everything that we have learned, on my own by now?
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsI was in the same position you are - I'd have struggled to do anything on my own. Or, at least, I felt like that. What the reality actually was is an academic point now.
Don't worry - just keep going, it will stick eventually!
Pauliina K
1,973 PointsPauliina K
1,973 PointsAlright, thanks Steve! I'll keep practicing!
Furquan Ahmad
5,148 PointsFurquan Ahmad
5,148 PointsI'm the same as Pauliina, i kind of understand the concepts, but i really wouldn't be able to do this on my own, i would be completely lost