Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial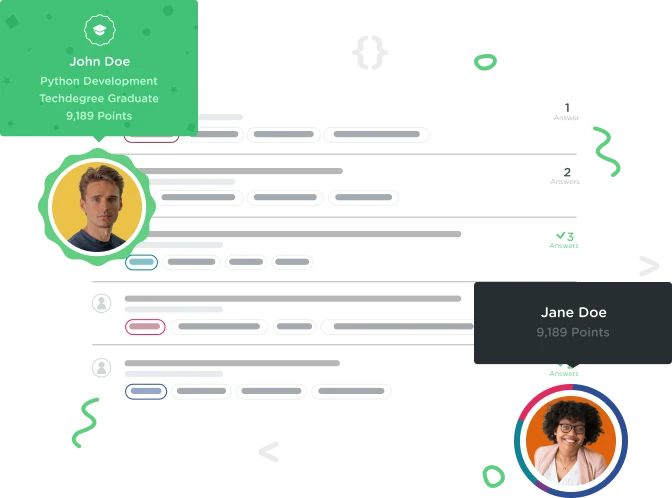
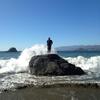
Joe Williams
4,014 PointsI'm not sure why this for statement is looping infinitely in my browser.
In one of my challenges, the goal is to write a program where it runs the numbers 100 through 0, and prints to the console.
I got that one easy enough.
for (var number = 100; number; number -1) {
console.log(number)
}
This got me thinking: what if I wanted to start at 0 and have the program stop when it hits 100? My friend, who knows Java, said this should work, but it's still running infinitely in my browser.
for (var number = 0; number < 100 ; number +1) {
console.log(number)
}
I also tried
for (var number = 0; number <= 100 ; number +1) {
console.log(number)
}
What's going on?
4 Answers
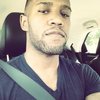
Ricky Walker
12,863 PointsHi Joe, I am experiencing your problem as well using that syntax but I encourage you to try using the syntax here:
for (var number = 0; number < 100 ; number++) {
console.log(number);
}
This does not give you an infinite loop like the previous code. The change I made was simply using the "++" operator for the increment condition rather than the "+ 1". I think that somehow messes up the condition which causes the infinite loop. Also, you don't have to use var in the initialization condition of the for loop but it's personal preference. Let me know if you're still having the same issue after making the changes. Have fun!
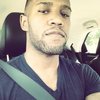
Ricky Walker
12,863 PointsTry using "number += 2" for the last condition.
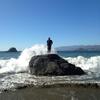
Joe Williams
4,014 PointsThat did it!
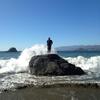
Joe Williams
4,014 PointsAlright, using this newfound logic, I tried to subtract 2. However, =-2, --2 and -2 didn't work.
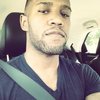
Ricky Walker
12,863 PointsAlmost there! You have to use "number -=2" lol. Whichever operation you want to take place, it has to be performed THEN set equal which is why the operator comes before the "=" sign. If you wanted to do multiplication, it would be "number *= 2".
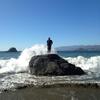
Joe Williams
4,014 PointsYou are a saint. THANK YOU. I've been going crazy trying to figure this out all night.
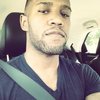
Ricky Walker
12,863 PointsHaha happy to help! I enjoy learning along with fellow coders. You also cement your new found knowledge when you teach it to others!

Ivan Yapeter
12,535 PointsHei Joe!
What happen in your code there, it's never get out of the number +1.
To use + 1 on third statement:
for (var number = 0; number < 100 ; number = number + 1) {
console.log(number);
}
or another way usually people do:
for (var number = 0; number < 100 ; number++) {
console.log(number);
}
Hope it helps, Cheers!
Reference: http://www.w3schools.com/js/js_loop_for.asp
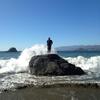
Joe Williams
4,014 PointsWhat if I wanted to add by an increment of 2? I got an error with both
for (var number = 0; number < 100 ; number+2) {
console.log(number);
}
and
for (var number = 0; number < 100 ; number++2) {
console.log(number);
}
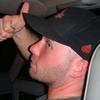
Lush Sleutsky
14,044 Pointsfor (var number = 0; number < 100 ; number+=2) {
console.log(number);
}
That should do the trick for you, for that one.
Just so you are aware, writing something like
number+=2
is the same as writing
number = number + 2
In your above code, when you write
number++
that is the same as writing
number = number + 1
Simply having something like
number++2
will not allow JavaScript to figure out what you are trying to increment, cause you are not setting your increment equal to anything...
Make sense?
Joe Williams
4,014 PointsJoe Williams
4,014 PointsThis worked! Thank you very much.
Ricky Walker
12,863 PointsRicky Walker
12,863 PointsNo prob! Have fun!