Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial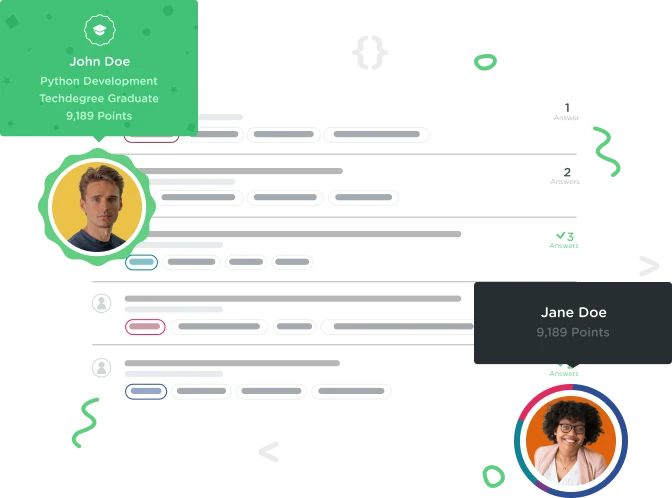
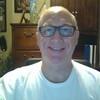
Tom Kaufman
5,130 PointsI'm not using val() properly ... so it tells me. Any guidance would be appreciated.
I guess val() can be a getter or setter. That's about all I know about it.
//Select the input for the title for blog post.
var $titleInput = $("#title");
//Select the preview h1 tag
var $previewTitle = $("#titlePreview");
// Every second update the preview
var previewTimer = setInterval(updatePreview, 1000);
function updatePreview(){
//Get the user's input
var titleValue=prompt("Type something please.");
//Set the user input as the preview title.
$previewTitle.text(titleValue);
$titleInput.val($previewTitle);
}
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" type="text/css" href="theme.css">
</head>
<body>
<div id="form">
<label for="title">Blog Post Title</label><input id="title" name="title" value="" placeholder="Enter your blog title here">
</div>
<div id="preview">
<h1 id="titlePreview"></h1>
</div>
<script src="//code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
#form {
width: 45%;
float: left;
}
#preview {
width: 45%;
float: right;
}
label {
width: 20%;
display: inline-block;
}
input {
width: 70%;
}
2 Answers

andren
28,558 PointsThe only thing you actually have to do to solve this challenge is to set the "titleValue" variable to the user's input, you don't need to add any new code to the page beyond that like you have done.
Also while I can certainly understand why you would think you have to prompt for the user input, that is not actually what the task wants. The user input it is looking for is the value that has been entered into the input element with the "title" id.
So the task wants you to set the "titleValue" variable equal to the value contained in the #title input element, that element is stored in the "$titleInput" variable.
The way you get a value out of a variable storing an element is by simply calling .val() on it, without passing in any arguments. If you pass an argument then it will act as a setter instead of a getter.
So basically remove the last line of your current solution since it is not needed, and change what you are setting the "titleValue" variable equal to, with the hints I give above you should be able to solve the challenge.
If you are still stuck then just say so, and I can post the solution for you if you want to see it.
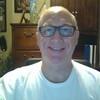
Tom Kaufman
5,130 PointsThanks Andren. That makes sense and I was able to solve the challenge.